JavaScript provides behavior to your web pages. From changing your HTML elements' positioning to performing Ajax operations, there are many things that JavaScript can do now compared to just a few years ago. Here's just a basic list of things that JavaScript can do:
Perform animation
Add in content
Create single-page applications
Use third-party JavaScript widgets, such as Google Analytics and Facebook's social plugins
Most importantly, with the rise of JavaScript libraries, such as jQuery, AngularJS, ReactJS, and more, achieving all this has never been easier. We'll see multiple examples of JavaScript with the use of jQuery just to give you a taste of some of the code we will see and use throughout this book.
For this section, we'll start with some basic animation effects before moving on to the topics that may be of concern in security-related topics. You will also need a text editor and a browser in order to test the code.
We'll start off with simple hide/show effects.
Note
We are using jQuery for this section (and the remainder of the book) for things such as Ajax, animation, and so forth, due to its widespread use and ease of understanding. The important thing is to take note of the lessons/concepts associated with JavaScript.
To perform hide/show actions, we can make use of jQuery's hide()
and show()
functions. For example, consider the following code:
<html> <head> <style> #item { display: block; height:100px; width:100px; border:1px solid black; background-color: yellow } </style> <script src="http://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script> <script> $(document).ready(function() { $("#hide").click(function(){ $("#item").hide(); }); $("#show").click(function(){ $("#item").show(); }); }); </script> </head> <body> <button id="show">Show Me</button> <button id="hide">Hide Me</button> <div id="item">I am item</div> </body> </html>
Tip
Downloading the example code
You can download the example code files from your account at http://www.packtpub.com for all the Packt Publishing books you have purchased. If you purchased this book elsewhere, you can visit http://www.packtpub.com/ support and register to have the files e-mailed directly to you.
Copy-and-paste this code to the hide_show.html
function, and open it in your favorite browser. You should get something like this:
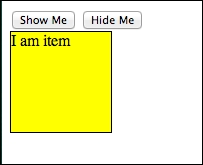
Simple show and hide actions
Clicking on
Show Me and Hide Me will show and hide the yellow box. You can do the same thing using the toggle()
function, which we will quickly cover in the next section.
The toggle()
function allows you to display and hide elements. Going back to the code we used in the previous section, create a new file and call it toggle.html
. Replace the code within $(document).ready()
with the following code:
$("#toggle_button").click(function(){ $("#item").toggle(); });
Feel free to make some changes to your button IDs and item contents. In my case, this is how my code looks:
<html> <head> <style> #item { display: block; height:100px; width:100px; border:1px solid black; background-color: yellow } </style> <script src="http://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script> <script> $(document).ready(function() { $("#toggle_button").click(function(){ $("#item").toggle(); }); }) </script> </head> <body> <button id="toggle_button">Toggle Button</button> <div id="item">Toggle Toggle Toggle</div> </body> </html>
This is what you will see when you open the file in your web browser:
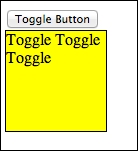
Simple toggle action
Clicking on Toggle Button will allow you to hide and show the yellow box as expected.
jQuery also provides easy methods to perform animations via the animate()
method. Copy the previous example (toggle.html
) and name it animation.html
. In animation.html
, make the following changes as shown in the highlighted lines of code:
<html> <head> <style> #item { display: block; position: relative; left:0px; height:100px; width:100px; border:1px solid black; background-color: yellow } </style> <script src="http://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script> <script> $(document).ready(function() { $("#animate_button").click(function(){ $("#item").animate({ opacity: 0.5, left: "+=50", }, 1000); }); }) </script> </head> <body> <button id="animate_button">Animate Button</button> <div id="item">Animate me</div> </body> </html>
We've basically changed #item
to display as block
with position:relative
. Now, the button ID is animate_button
. Notice that the animate()
function works on the item when the button is clicked. The following is what you will get when you click on Animate Button:
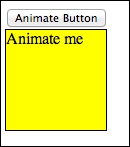
Animation
The animation looks like the following:
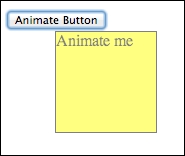
Animation part 2
One of the more interesting uses of jQuery is the chaining of functions. We'll do a basic example using the chaining of built-in animations. Go back to your text editor, create a new file called chained.html
, and paste the following code:
<html> <head> <style> #item { display: none; position: relative; left:0px; height:100px; width:100px; border:1px solid black; background-color: yellow } </style> <script src="http://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script> <script> $(document).ready(function() { $('#chain_button').click(function() { $("#item").fadeIn('slow').fadeOut('slow').fadeIn('slow'). fadeOut('slow').slideDown('slow').slideUp('slow'); }) }) </script> </head> <body> <button id="chain_button">Chained Button</button> <div id="item">Chain me</div> </body> </html>
The main thing to notice in this example is the use of the fadeIn()
, fadeout()
, slideDown()
, and slideUp()
functions. We chain the built-in animations together such that we see a series of effects when we click on the button.
Now, we focus on the jQuery Ajax operations. The basic concepts discussed here will be used in the next chapter, where we will talk about secure RESTful APIs. For a start, Ajax typically refers to Asynchronous JavaScript and XML, where your web page performs data operations with a server to get new data, create or update data, or delete data. During the past few years, with the rise in popularity of APIs (such as the Facebook Graph API and others), data is increasingly being exchanged using JSON instead of XML. Such actions typically require the cooperation of a backend server. We will not cover the server details here; for the moment, we will just focus on the jQuery operations.
In any Ajax application, single page or not, you will most likely be required to perform the basic HTTP operations, such as GET
, POST
, and so on. In this section, we will deal with the basic operations that you will most likely use in coding Ajax apps. Most importantly, you will use variants of this code in the later chapters.
A jQuery .get()
request simply performs a GET request from a server. To perform a .get()
request, you will need the following code:
var jqxhr = $.get("http://example.com/data", function() { alert( "success" ); }) .done(function() { alert( "second success" ); }) .fail(function() { alert( "error" ); }) .always(function() { alert( "finished" ); });
The hypothetical endpoint in this example, http://example.com/data
, can return either XML or JSON.
A jQuery .getJSON()
request simply performs a GET request from a server. But this time around, we are attempting to retrieve JSON data from our server. To perform a .getJSON()
request, here's what we do:
var jqxhr = $.getJSON( "http://example.com/json", function(data) { console.log( "success" ); }) .done(function(data) { console.log( "second success" ); }) .fail(function(data) { console.log( "error" ); }) .always(function(data) { console.log( "complete" ); });
In this example, we perform a getJSON()
request from http://example.com/json
; the endpoint should return a JSON response.
If you want to change the data source of your data or create a new one, you will need to perform a POST operation on your server. In this example, we perform a .post()
operation to http://example.com/endpoint
, and depending on whether our Ajax request is successful or not, we create an alert with different messages. This is done with the following code:
var jqxhr = $.post( "http://example.com/endpoint", function(data) { alert( "success" ); }) .done(function(data) { alert( "second success" ); }) .fail(function(data) { alert( "error" ); }) .always(function(data) { alert( "finished" ); });