Now that the session management and user store plumbing are in place, we turn our attention to user-related views and components.
The log in view is our simplest form. Here's what it looks like in action:
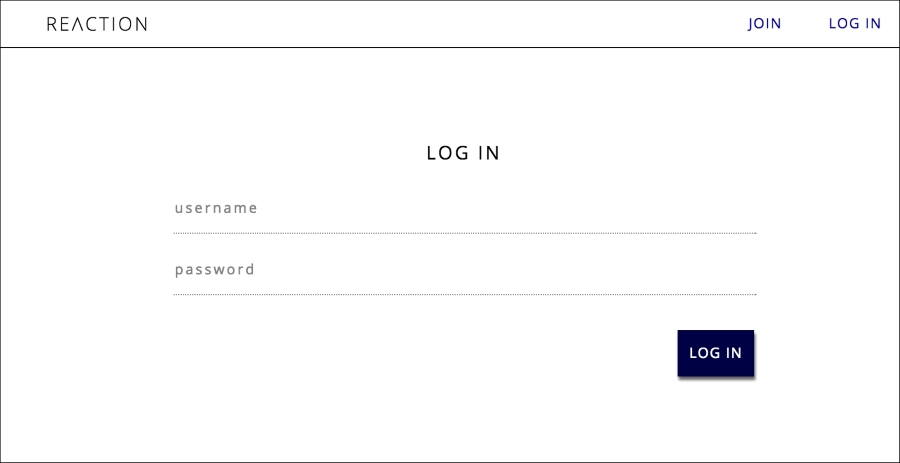
The log in view
Here's the source for the log in view:
File: js/views/login.jsx
import React from 'react'; import { History } from 'react-router'; import BasicInput from 'appRoot/components/basicInput'; import Actions from 'appRoot/actions'; export default React.createClass({ mixins: [ History ], getInitialState: function () { return {}; }, logIn: function (e) { var detail = {}; Array.prototype.forEach.call( e.target.querySelectorAll('input'), function (v) { detail[v.getAttribute('name')] = v.value; }); e.preventDefault(); e.stopPropagation(); Actions.login(detail.username, detail.password) .then(function () { this.history.pushState('', '/'); }.bind(this)) ['catch'](function...