Relational databases have been one of the most widely used and most common forms of software systems for the storage of data since the 1970s. They are highly structured and store data in the form of tables, that is, with rows and columns. Structuring and storing data in the form of rows and columns has its own advantages; for example, it is easier to understand and locate data, reduce data redundancy by applying normalization, maintain data integrity, and much more.
But is this the best way to store any kind of data?
Let's consider an example of social networking:
Mike, John, and Claudia are friends. Claudia is married to Wilson. Mike and Wilson work for the same company.
Here is one of the possible ways to structure this data in a relational database:
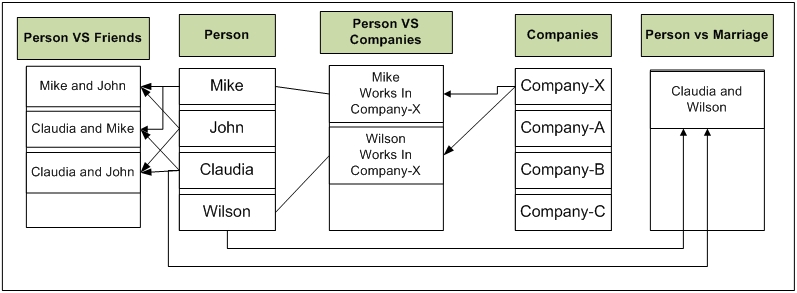
Complex, isn't it? And it can be more complex!
We should remember that relationships are evolving, and will evolve over a period of time. There could be new relationships, or there could be changes to existing relationships.
We can design a better structure but in any case, wouldn't that be forcibly fitting the model into a structure?
RDBMS is good for use cases where the relationship between entities is more or less static and does not change over a period of time. Moreover, the focus of RDBMS is more on the entities and less on the relationships between them.
There could be many more examples where RDBMS may not be the right choice:
Model and store 7 billion people objects and 3 billion non-people objects to provide an "earth view" drill-down from the planet to a sidewalk
Network management
Genealogy
Public transport links and road maps
Consider another way of modelling the same data:
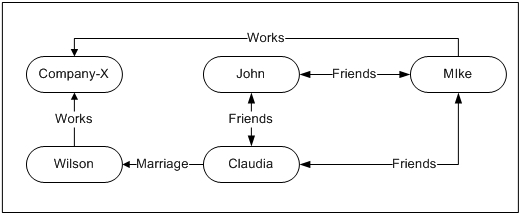
Simple, isn't it?
Welcome to the world of Neo4j—a graph database.
Although there is no single definition of graphs, here is the simplest one (http://en.wikipedia.org/wiki/Graph_(abstract_data_type)), which helps us to understand the theory of graphs:
A graph data structure consists of a finite (and possibly mutable) set of nodes or vertices, together with a set of ordered pairs of these nodes (or, in some cases, a set of unordered pairs). These pairs are known as edges or arcs. As in mathematics, an edge (x,y) is said to point or go from x to y. The nodes may be part of the graph structure, or may be external entities represented by integer indices or references.
Neo4j, as an open source graph database, is part of the NoSQL family, and provides a flexible data structure, where the focus is on the relationships between the entities rather than the entities themselves.
Its first version (1.0) was released in February 2010, and since then, it has never stopped. It is amazing to see the pace at which Neo4J has evolved over the years. At the time of writing this book, the stable version was 2.2.RC01, which was released in March 2015.
If you are reading this book, then you probably already have sufficient knowledge about graph databases and Python. You will appreciate their contribution to the complex world of relationships.
Let's move forward and jump into the nitty-gritty of developing web applications with Python and Neo4j.
In the subsequent chapters, we will cover the various aspects dealing with data modelling, programming, and data analysis by means of application development with Python and Neo4j. We will cover the concepts of working with py2neo, Django, flask, and many more.
Chapter 1, Your First Query with Neo4j, details the process of the installation of Neo4j and Python on Windows and Linux. This chapter briefly explains the function of every tool installed together with Neo4j (shell, server, and browser). More importantly, it introduces, and helps you get familiar with, the Neo4j browser. You get to run the first basic Cypher query by using different methods exposed by Neo4j (shell, Java, the browser, and REST).
Chapter 2, Querying the Graph with Cypher, starts by explaining Cypher as a graph query language for Neo4j, and then we take a deep dive into the various Cypher constructs to perform read operations. This chapter also talks about the importance of patterns and pattern matching, and their usage in Cypher with various real-world and easy-to-understand examples.
Chapter 3, Mutating Graph with Cypher, starts by covering the Cypher constructs used to perform write operations on the Neo4j database. This chapter further talks about creating relationships between nodes and discusses the constraints required for maintaining the integrity of data. At the end, it discuss about the performance tuning of Cypher queries using various optimization techniques.
Chapter 4, Getting Python and Neo4j to Talk Py2neo, introduces Py2neo as a Python framework for working with Neo4j. This chapter explores various Python APIs exposed by Py2neo for working with Neo4j. It also talks about batch imports and introduces a social network use case, which is created and unit tested by using Py2neo APIs.
Chapter 5, Build RESTful Service with Flask and Py2neo, talks about building web applications and the integration of Flask and Py2neo. This chapter starts with the basics of Flask as a framework for exposing ReSTful APIs, and further talks about the Py2neo extension OGM (short for Object Graph Mapper) and its integration with Flask for performing various CRUD and search operations on the social network use case by creating and leveraging various ReST endpoints.
Chapter 6, Using Neo4j with Django and Neomodel, starts by describing Neomodel as an ORM for Neo4j. It discusses various high-level APIs exposed by Neomodel to perform CRUD and search operations using Python APIs or by directly executing Cypher queries. Finally, it talks about integration of two of the popular Python frameworks, Django and Neomodel.
Chapter 7, Deploying Neo4j in Production, explains the logical architecture of Neo4j, its various components, or APIs, such as filesystems, data organization and so on. Then we move on to the physical architecture of Neo4j, where we talk about meeting various NFRs imposed by typical enterprise deployments, such as HA, fault tolerance, data locality, backup, and recovery. Further, this chapter talks about various advanced Neo4j configurations and also discusses the various ways to monitor our Neo4j deployments.
Readers should have programming experience in Python and some basic knowledge or understanding of any graph or NoSQL databases.
This book is aimed at competent developers who have a good knowledge and understanding of Python that can allow efficient programming of the core elements and applications.
If you are reading this book, then you probably already have sufficient knowledge of Python. This book will cover data modelling, programming, and data analysis by means of application development with Python and Neo4j. It will cover concepts such as working with py2neo, Django, flask, and so on.
In this book, you will find a number of text styles that distinguish between different kinds of information. Here are some examples of these styles and an explanation of their meaning.
Code words in text, database table names, folder names, filenames, file extensions, pathnames, dummy URLs, user input, and Twitter handles are shown as follows: "We can include other contexts through the use of the include
directive."
A block of code is set as follows:
MATCH (x { name: "Bradley" })--(y)-->() WITH x CREATE (n:Male {name:"Smith", Age:"24"})-[r:FRIEND]->(x) returnn,r,x;
Any command-line input or output is written as follows:
pip install flask Flask-RESTful
New terms and important words are shown in bold. Words that you see on the screen, for example, in menus or dialog boxes, appear in the text like this: "Now, click on the star sign in the panel on the extreme left-hand side, and click on Create a node in the provided menu."
Feedback from our readers is always welcome. Let us know what you think about this book—what you liked or disliked. Reader feedback is important for us as it helps us develop titles that you will really get the most out of.
To send us general feedback, simply e-mail <[email protected]>
, and mention the book's title in the subject of your message.
If there is a topic that you have expertise in and you are interested in either writing or contributing to a book, see our author guide at www.packtpub.com/authors.
Now that you are the proud owner of a Packt book, we have a number of things to help you to get the most from your purchase.
You can download the example code files from your account at http://www.packtpub.com for all the Packt Publishing books you have purchased. If you purchased this book elsewhere, you can visit http://www.packtpub.com/support and register to have the files e-mailed directly to you.
Although we have taken every care to ensure the accuracy of our content, mistakes do happen. If you find a mistake in one of our books—maybe a mistake in the text or the code—we would be grateful if you could report this to us. By doing so, you can save other readers from frustration and help us improve subsequent versions of this book. If you find any errata, please report them by visiting http://www.packtpub.com/submit-errata, selecting your book, clicking on the Errata Submission Form link, and entering the details of your errata. Once your errata are verified, your submission will be accepted and the errata will be uploaded to our website or added to any list of existing errata under the Errata section of that title.
To view the previously submitted errata, go to https://www.packtpub.com/books/content/support and enter the name of the book in the search field. The required information will appear under the Errata section.
Piracy of copyrighted material on the Internet is an ongoing problem across all media. At Packt, we take the protection of our copyright and licenses very seriously. If you come across any illegal copies of our works in any form on the Internet, please provide us with the location address or website name immediately so that we can pursue a remedy.
Please contact us at <[email protected]>
with a link to the suspected pirated material.
We appreciate your help in protecting our authors and our ability to bring you valuable content.
If you have a problem with any aspect of this book, you can contact us at <[email protected]>
, and we will do our best to address the problem.