Reactive programming is a paradigm where the main focus is working with an asynchronous data flow. You may read many books and see throughout the Internet that the reactive paradigm is about propagation of changes or some technical explanation that only makes it harder to understand.
Imperative programming makes you describe the steps a computer must do to execute a task. In comparison, functional reactive programming gives you the constructs to propagate the changes so you can focus on what to do instead of how to do it.
This can be illustrated in a simple sum of two numbers. In imperative programming a = b + c is evaluated only in that line of code, so if you change the value of b or c, it doesn't change the value of a. But in a reactive programming world, you can listen for the changes. Imagine the same sum in a Microsoft Excel spreadsheet, and every time you change the value of the column b (or c), it recalculates the value of a, so you are always propagating the change for the ones interested in those changes.
The truth is that you probably already use an asynchronous data flow every time you add a listener to a mouse click or a keystroke in a web page you pass as an argument to a function to react to that user input. So, a mouse click can be seen as a stream of events that you can observe and execute a function on when it happens. But this is only one usage of event streams.
Reactive programming takes this to the next level–using it you can listen and react to changes in anything creating a stream of events from it, so you can react to changes in a variable or property, database, user inputs, external sources, and so on. For example, you can see the changes of value in a stock as an event stream and use it to show your user when to buy or sell in real time. Another common example for external sources streams is your Twitter feed or your Facebook timeline. Also, functional reactive programming gives you the possibility to filter, map, combine, buffer, and do a lot more with your streams of data or events. So using the stock example, you can easily listen to different stocks using a filter function to get the ones worth buying and show to the user a real time list of them, as shown in the following diagram:
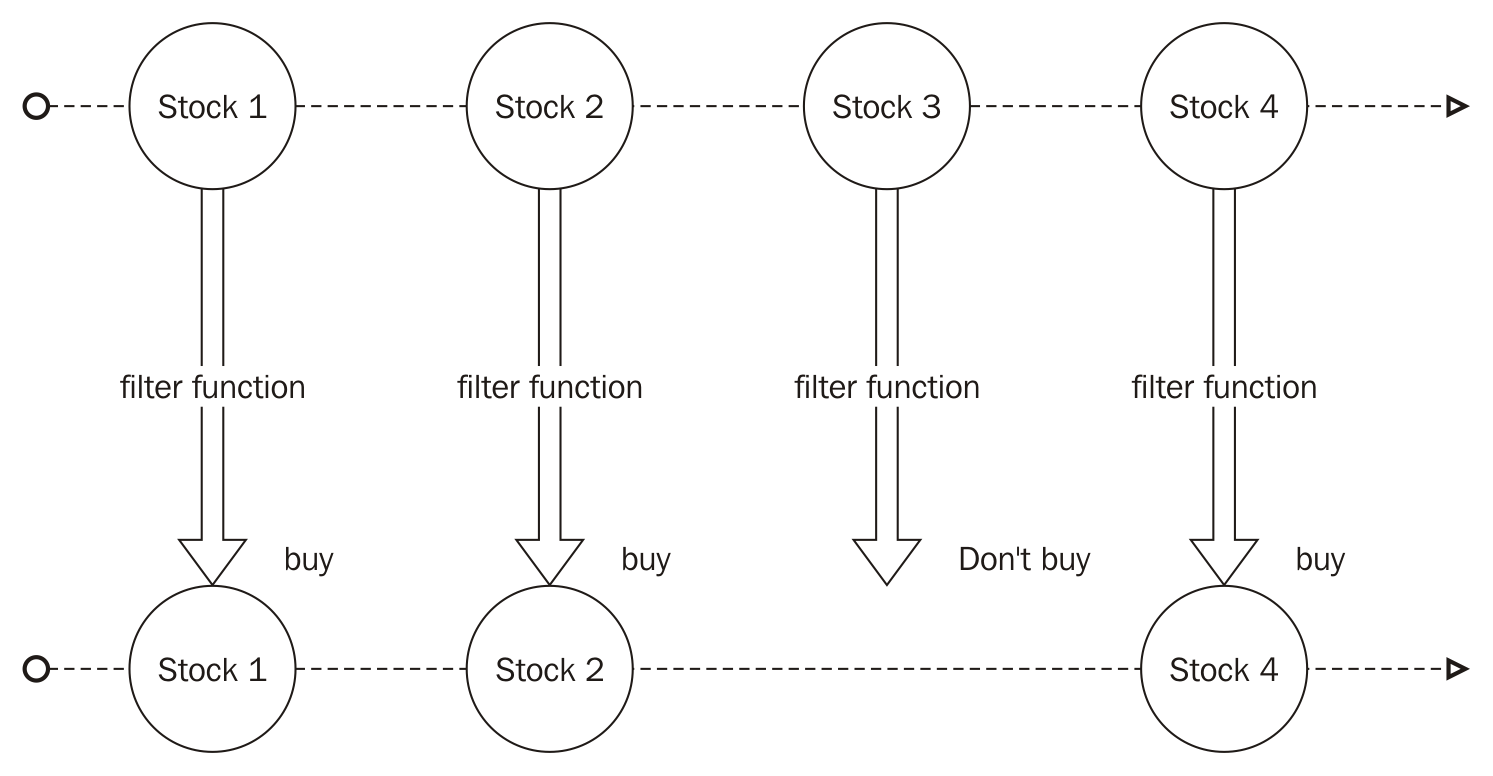