Writing unit tests for Visualforce page controllers is often a source of confusion for developers new to the technology. A common mistake is to assume that the page must somehow be rendered and interacted with in the test context, whereas in reality, the page is very much a side issue. Instead, tests must instantiate the controller and set its internal state as though the user interaction has already taken place, and then execute one or more controller methods and confirm that the state has changed as expected.
In this recipe, we will unit test SearchFromURLController
from the Reacting to URL parameters recipe.
This recipe requires that you have already completed the Reacting to URL parameters recipe, as it relies on SearchFromURLController
being present in your Salesforce instance.
Create the unit test class by navigating to the Apex Classes setup page and by clicking on Your Name | Setup | Develop | Apex Classes.
Click on the New button.
Paste the contents of the
SearchFromURLControllerTest.cls
Apex class from the code download into the Apex Class area.Click on the Save button.
On the resulting page, click on the Run Tests button.
The tests successfully execute, as shown in the following screenshot:
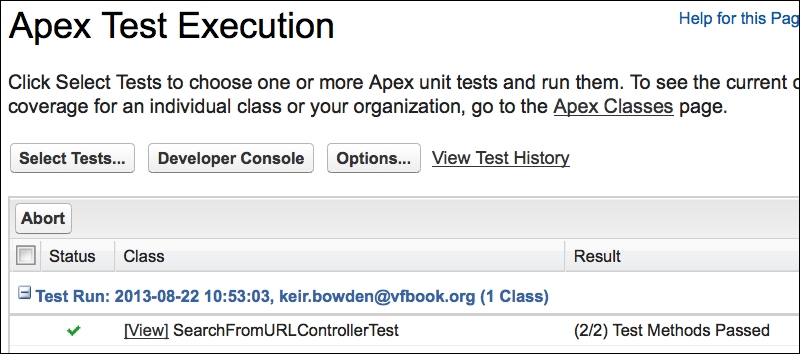
Open the Developer Console by clicking on your name at the top right of the screen and selecting Developer Console from the resulting drop-down menu:
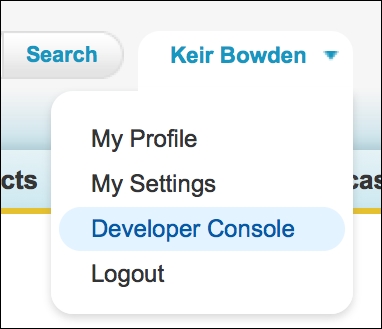
Select the Tests tab and you will see the test coverage information on the right-hand side, showing 100% code coverage for the SearchFromURLController
class:
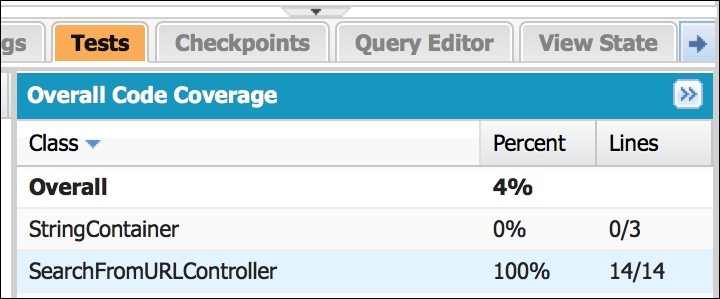
Note
Percentage coverage is important as at least 75 % coverage across all code must be achieved before classes may be deployed to a production organization.
The test class contains two unit test methods. The first method tests that the search is correctly executed when the search term is passed on the page URL. As unit tests do not have access to organization data, the first task for the test is to set up three test accounts:
List<Account> accs=new List<Account>(); accs.add(new Account(Name='Unit Test')); accs.add(new Account(Name='Unit Test 2')); accs.add(new Account(Name='The Test Account')); insert accs;
As the controller is reacting to parameters on the URL, the page reference must be set up and populated with the name
parameter:
PageReference pr=Page.SearchFromURL; pr.getParameters().put('name', 'Unit'); Test.setCurrentPage(pr);
Finally, the controller is instantiated, which causes the action method that executes the search to be invoked from the constructor. The test method then confirms that the search was executed and the actual number of matches equals the expected number:
SearchFromURLController controller=new SearchFromURLController(); System.assertEquals(true, controller.searched); System.assertEquals(2, controller.accounts.size());
The second unit test method tests that the search is correctly executed when the user enters a search term. In this case, there is no interaction with the information on the page URL, so the test simply instantiates the controller and confirms that no search has been executed by the constructor:
SearchFromURLController controller=new SearchFromURLController(); System.assertEquals(false, controller.searched);
The test then sets the search term, executes the search method, and confirms the results:
controller.name='Unit'; System.assertEquals(null, controller.executeSearch()); System.assertEquals(2, controller.accounts.size());