Let's create the simplest possible program in Vue.js, the obligatory Hello World program. The objective here is to get our feet wet with how Vue manipulates your webpage and how data binding works.
To complete this introductory recipe, we will only need the browser. That is, we will use JSFiddle to write our code:
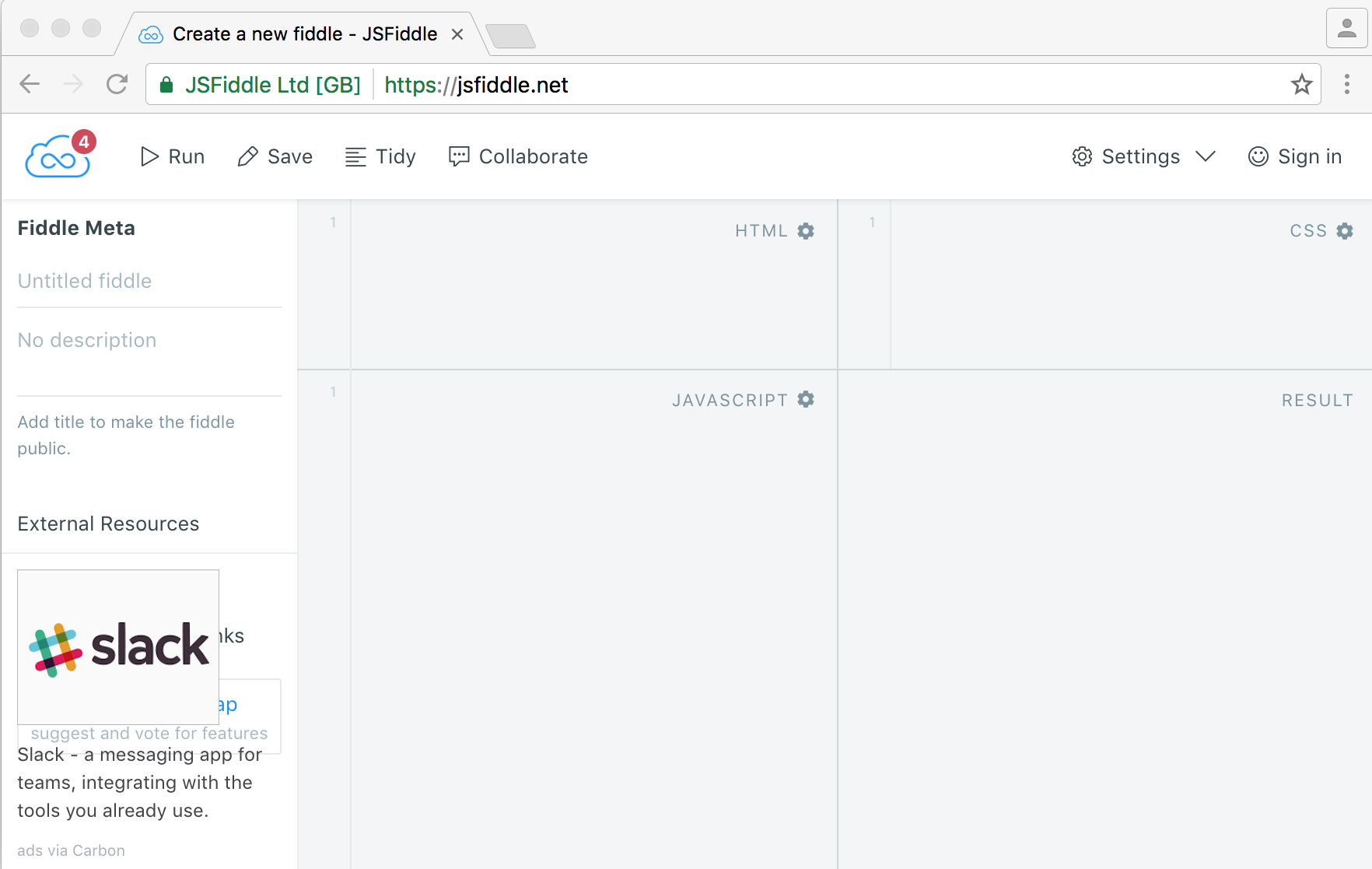
If you have never used JSFiddle, don't worry; you are about to become an expert frontend developer and using JSFiddle will become a handy tool in your pocket:
- Head your browser to https://jsfiddle.net:
You will be presented with a blank page divided into quadrants. The bottom-left is where we will write our JavaScript code. Going clockwise, we have an HTML section, a CSS section, and finally our preview of the resulting page.
Before beginning, we should tell JSFiddle that we want to use the Vue library.
- In the top-right part of the JavaScript quadrant, press the cogwheel and select Vue 2.2.1 from the list (you should find more than one version, "edge" refers to the latest version and at the time of writing corresponds to Vue 2).
We are now ready to write our first Vue program.
- In the JavaScript section, write:
new Vue({el:'#app'})
- In the HTML quadrant, we create the
<div>
:
<div id="app"> {{'Hello ' + 'world'}} </div>
- Click the
Run
button in the upper-left corner; we see the page greeting us withHello world
:
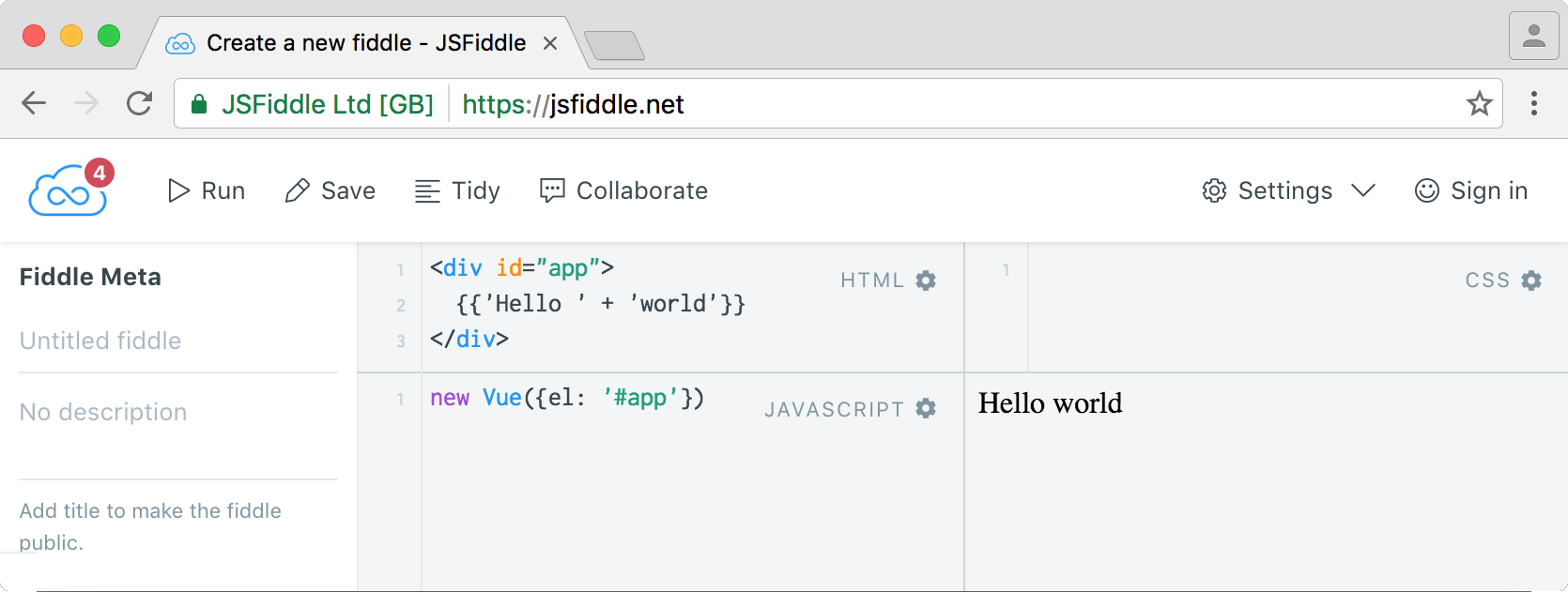
new Vue({el:'#app'})
will instantiate a new Vue instance. It accepts an options object as a parameter. This object is central in Vue, and defines and controls data and behavior. It contains all the information needed to create Vue instances and components. In our case, we only specified the el
option which accepts a selector or an element as an argument. The #app
parameter is a selector that will return the element in the page with app
as the identifier. For example, in a page like this:
<!DOCTYPE html> <html> <body> <div id="app"></div> </body> </html>
Everything that we will write inside the <div>
with the ID as app
will be under the scope of Vue.
Now, JSFiddle takes everything we write in the HTML quadrant and wraps it in body tags. This means that if we just need to write the <div>
in the HTML quadrant, JSFiddle will take care of wrapping it in the body tags.
Note
It's also important to note that placing the #app
on the body
or html
tag will throw an error, as Vue advises us to mount our apps on normal elements, and its the same thing goes for selecting the body
in the el
option.
The mustaches (or handlebars) are a way to tell Vue to take everything inside them and parse it as code. The quotes are a normal way to declare a literal string in JavaScript, so Vue just returns the string concatenation of hello
and world
. Nothing fancy, we just concatenated two strings and displayed the result.
We can leverage that to do something more interesting. If we were aliens and we wanted to greet more than one world at a time, we could write:
We conquered 5 planets.<br/> {{'Hello ' + 5 + ' worlds'}}
We may lose track of how many worlds we conquer. No problem, we can do math inside the mustaches. Also, let's put Hello
and worlds
outside brackets:
We conquered {{5 + 2}} planets.<br/> Hello {{5 + 2}} worlds
Having the number of worlds as raw numbers inside the mustaches is just messy. We are going to use data binding to put it inside a named variable inside our instance:
<div id="app"> We conquered {{countWorlds}} planets.<br/> Hello {{countWorlds}} worlds </div> new Vue({ el:'#app', data: { countWorlds: 5 + 2 } })
This is how tidy applications are done. Now, every time we conquer a planet, we have to edit only the countWorlds
variable. In turn, every time we modify this variable, the HTML will be automatically updated.
Congratulations, you completed your first step into the Vue world and are now able to build simple interactive applications with reactive data-binding and string interpolation.