JavaScript's most fundamental data type is the Object data type. JavaScript objects can be seen as mutable key-value-based collections. In JavaScript, arrays, functions, and RegExp are objects while numbers, strings, and Booleans are object-like constructs that are immutable but have methods. In this chapter, you will learn the following topics:
- Understanding objects
- Instance properties versus prototype properties
- Inheritance
- Getters and setters
Before we start looking at how JavaScript treats objects, we should spend some time on an object-oriented paradigm. Like most programming paradigms, object-oriented programming (OOP) also emerged from the need to manage complexity. The main idea is to divide the entire system into smaller pieces that are isolated from each other. If these small pieces can hide as many implementation details as possible, they become easy to use. A classic car analogy will help you understand this very important point about OOP.
When you drive a car, you operate on the interface—the steering, clutch, brake, and accelerator. Your view of using the car is limited by this interface, which makes it possible for us to drive the car. This interface is essentially hiding all the complex systems that really drive the car, such as the internal functioning of its engine, its electronic system, and so on. As a driver, you don't bother about these complexities. A similar idea is the primary driver of OOP. An object hides the complexities of how to implement a particular functionality and exposes a limited interface to the outside world. All other systems can use this interface without really bothering about the internal complexity that is hidden from view. Additionally, an object usually hides its internal state from other objects and prevents its direct modification. This is an important aspect of OOP.
In a large system where a lot of objects call other objects' interfaces, things can go really bad if you allow them to modify the internal state of such objects. OOP operates on the idea that the state of an object is inherently hidden from the outside world and it can be changed only via controlled interface operations.
OOP was an important idea and a definite step forward from the traditional structured programming. However, many feel that OOP is overdone. Most OOP systems define complex and unnecessary class and type hierarchies. Another big drawback was that in the pursuit of hiding the state, OOP considered the object state almost immaterial. Though hugely popular, OOP was clearly flawed in many areas. Still, OOP did have some very good ideas, especially hiding the complexity and exposing only the interface to the outside world. JavaScript picked up a few good ideas and built its object model around them. Luckily, this makes JavaScript objects very versatile. In their seminal work, Design Patterns: Elements of Reusable Object-Oriented Software, the Gang of Four gave two fundamental principles of a better object-oriented design:
- Program to an interface and not to an implementation
- Object composition over class inheritance
These two ideas are really against how classical OOP operates. The classical style of inheritance operates on inheritance that exposes parent classes to all child classes. Classical inheritance tightly couples children to its parents. There are mechanisms in classical inheritance to solve this problem to a certain extent. If you are using classical inheritance in a language such as Java, it is generally advisable to program to an interface, not an implementation. In Java, you can write a decoupled code using interfaces:
//programming to an interface 'List' and not implementation 'ArrayList' List theList = new ArrayList();
Instead of programming to an implementation, you can perform the following:
ArrayList theList = new ArrayList();
How does programming to an interface help? When you program to the List
interface, you can call methods only available to the List
interface and nothing specific to ArrayList
can be called. Programming to an interface gives you the liberty to change your code and use any other specific child of the List
interface. For example, I can change my implementation and use LinkedList
instead of ArrayList
. You can change your variable to use LinkedList
instead:
List theList = new LinkedList();
The beauty of this approach is that if you are using the List
at 100 places in your program, you don't have to worry about changing the implementation at all these places. As you were programming to the interface and not to the implementation, you were able to write a loosely coupled code. This is an important principle when you are using classical inheritance.
Classical inheritance also has a limitation where you can only enhance the child class within the limit of the parent classes. You can't fundamentally differ from what you have got from the ancestors. This inhibits reuse. Classical inheritance has several other problems as follows:
- Inheritance introduces tight coupling. Child classes have knowledge about their ancestors. This tightly couples a child class with its parent.
- When you subclass from a parent, you don't have a choice to select what you want to inherit and what you don't. Joe Armstrong (the inventor of Erlang) explains this situation very well—his now famous quote:
"The problem with object-oriented languages is they've got all this implicit environment that they carry around with them. You wanted a banana but what you got was a gorilla holding the banana and the entire jungle."
With this background, let's explore how JavaScript objects behave. In broad terms, an object contains properties, defined as a key-value pair. A property key (name) can be a string and the value can be any valid JavaScript value. You can create objects using object literals. The following snippet shows you how object literals are created:
var nothing = {}; var author = { "firstname": "Douglas", "lastname": "Crockford" }
A property's name can be any string or an empty string. You can omit quotes around the property name if the name is a legal JavaScript name. So quotes are required around first-name
but are optional around firstname
. Commas are used to separate the pairs. You can nest objects as follows:
var author = { firstname : "Douglas", lastname : "Crockford", book : { title:"JavaScript- The Good Parts", pages:"172" } };
Properties of an object can be accessed by using two notations: the array-like notation and dot notation. According to the array-like notation, you can retrieve the value from an object by wrapping a string expression in []
. If the expression is a valid JavaScript name, you can use the dot notation using .
instead. Using .
is a preferred method of retrieving values from an object:
console.log(author['firstname']); //Douglas console.log(author.lastname); //Crockford console.log(author.book.title); // JavaScript- The Good Parts
You will get an
undefined
error if you attempt to retrieve a non-existent value. The following would return undefined
:
console.log(author.age);
A useful trick is to use the ||
operator to fill in default values in this case:
console.log(author.age || "No Age Found");
You can update values of an object by assigning a new value to the property:
author.book.pages = 190; console.log(author.book.pages); //190
If you observe closely, you will realize that the object literal syntax that you see is very similar to the JSON format.
Methods are properties of an object that can hold function values as follows:
var meetingRoom = {}; meetingRoom.book = function(roomId){ console.log("booked meeting room -"+roomId); } meetingRoom.book("VL");
Apart from the properties that we add to an object, there is one default property for almost all objects, called a prototype. When an object does not have a requested property, JavaScript goes to its prototype to look for it. The Object.getPrototypeOf()
function returns the prototype of an object.
Many programmers consider prototypes closely related to objects' inheritance—they are indeed a way of defining object types—but fundamentally, they are closely associated with functions.
Prototypes are used as a way to define properties and functions that will be applied to instances of objects. The prototype's properties eventually become properties of the instantiated objects. Prototypes can be seen as blueprints for object creation. They can be seen as analogous to classes in object-oriented languages. Prototypes in JavaScript are used to write a classical style object-oriented code and mimic classical inheritance. Let's revisit our earlier example:
var author = {}; author.firstname = 'Douglas'; author.lastname = 'Crockford';
In the preceding code snippet, we are creating an empty object and assigning individual properties. You will soon realize that this is not a very standard way of building objects. If you know OOP already, you will immediately see that there is no encapsulation and the usual class structure. JavaScript provides a way around this. You can use the new
operator to instantiate an object via constructors. However, there is no concept of a class in JavaScript, and it is important to note that the new
operator is applied to the constructor function. To clearly understand this, let's look at the following example:
//A function that returns nothing and creates nothing function Player() {} //Add a function to the prototype property of the function Player.prototype.usesBat = function() { return true; } //We call player() as a function and prove that nothing happens var crazyBob = Player(); if(crazyBob === undefined){ console.log("CrazyBob is not defined"); } //Now we call player() as a constructor along with 'new' //1. The instance is created //2. method usesBat() is derived from the prototype of the function var swingJay = new Player(); if(swingJay && swingJay.usesBat && swingJay.usesBat()){ console.log("SwingJay exists and can use bat"); }
In the preceding example, we have a player()
function that does nothing. We invoke it in two different ways. The first call of the function is as a normal function and second call is as a constructor—note the use of the new()
operator in this call. Once the function is defined, we add a usesBat()
method to it. When this function is called as a normal function, the object is not instantiated and we see undefined
assigned to crazyBob
. However, when we call this function with the new
operator, we get a fully instantiated object, swingJay
.
Instance properties are the properties that are part of the object instance itself, as shown in the following example:
function Player() { this.isAvailable = function() { return "Instance method says - he is hired"; }; } Player.prototype.isAvailable = function() { return "Prototype method says - he is Not hired"; }; var crazyBob = new Player(); console.log(crazyBob.isAvailable());
When you run this example, you will see that Instance method says - he is hired is printed. The isAvailable()
function defined in the Player()
function is called an instance of Player
. This means that apart from attaching properties via the prototype, you can use the this keyword to initialize properties in a constructor. When we have the same functions defined as an instance property and also as a prototype, the instance property takes precedence. The rules governing the precedence of the initialization are as follows:
- Properties are tied to the object instance from the prototype
- Properties are tied to the object instance in the constructor function
This example brings us to the use of the this
keyword. It is easy to get confused by the this
keyword because it behaves differently in JavaScript. In other OO languages such as Java, the this
keyword refers to the current instance of the class. In JavaScript, the value of this
is determined by the invocation context of a function and where it is called. Let's see how this behavior needs to be carefully understood:
- When
this
is used in a global context: Whenthis
is called in a global context, it is bound to the global context. For example, in the case of a browser, the global context is usuallywindow
. This is true for functions also. If you usethis
in a function that is defined in the global context, it is still bound to the global context because the function is part of the global context:function globalAlias(){ return this; } console.log(globalAlias()); //[object Window]
- When
this
is used in an object method: In this case,this
is assigned or bound to the enclosing object. Note that the enclosing object is the immediate parent if you are nesting the objects:var f = { name: "f", func: function () { return this; } }; console.log(f.func()); //prints - //[object Object] { // func: function () { // return this; // }, // name: "f" //}
- When there is no context: A function, when invoked without any object, does not get any context. By default, it is bound to the global context. When you use
this
in such a function, it is also bound to the global context. - When
this
is used in a constructor function: As we saw earlier, when a function is called with anew
keyword, it acts as a constructor. In the case of a constructor,this
points to the object being constructed. In the following example,f()
is used as a constructor (because it's invoked with anew
keyword) and hence,this
is pointing to the new object being created. So when we saythis.member = "f"
, the new member is added to the object being created, in this case, that object happens to beo
:var member = "global"; function f() { this.member = "f"; } var o= new f(); console.log(o.member); // f
We saw that instance properties take precedence when the same property is defined both as an instance property and prototype property. It is easy to visualize that when a new object is created, the properties of the constructor's prototype are copied over. However, this is not a correct assumption. What actually happens is that the prototype is attached to the object and referred when any property of this object is referred. Essentially, when a property is referenced on an object, either of the following occur:
- The object is checked for the existence of the property. If it's found, the property is returned.
- The associated prototype is checked. If the property is found, it is returned; otherwise, an
undefined
error is returned.
This is an important understanding because, in JavaScript, the following code actually works perfectly:
function Player() { isAvailable=false; } var crazyBob = new Player(); Player.prototype.isAvailable = function() { return isAvailable; }; console.log(crazyBob.isAvailable()); //false
This code is a slight variation of the earlier example. We are creating the object first and then attaching the function to its prototype. When you eventually call the isAvailable()
method on the object, JavaScript goes to its prototype to search for it if it's not found in the particular object (crazyBob
, in this case). Think of this as hot code loading—when used properly, this ability can give you incredible power to extend the basic object framework even after the object is created.
If you are familiar with OOP already, you must be wondering whether we can control the visibility and access of the members of an object. As we discussed earlier, JavaScript does not have classes. In programming languages such as Java, you have access modifiers such as private
and public
that let you control the visibility of the class members. In JavaScript, we can achieve something similar using the function scope as follows:
- You can declare private variables using the
var
keyword in a function. They can be accessed by private functions or privileged methods. - Private functions may be declared in an object's constructor and can be called by privileged methods.
- Privileged methods can be declared with
this.method=function() {}
. - Public methods are declared with
Class.prototype.method=function(){}
. - Public properties can be declared with
this.property
and accessed from outside the object.
The following example shows several ways of doing this:
function Player(name,sport,age,country){ this.constructor.noOfPlayers++; // Private Properties and Functions // Can only be viewed, edited or invoked by privileged members var retirementAge = 40; var available=true; var playerAge = age?age:18; function isAvailable(){ return available && (playerAge<retirementAge); } var playerName=name ? name :"Unknown"; var playerSport = sport ? sport : "Unknown"; // Privileged Methods // Can be invoked from outside and can access private members // Can be replaced with public counterparts this.book=function(){ if (!isAvailable()){ this.available=false; } else { console.log("Player is unavailable"); } }; this.getSport=function(){ return playerSport; }; // Public properties, modifiable from anywhere this.batPreference="Lefty"; this.hasCelebGirlfriend=false; this.endorses="Super Brand"; } // Public methods - can be read or written by anyone // Can only access public and prototype properties Player.prototype.switchHands = function(){ this.batPreference="righty"; }; Player.prototype.dateCeleb = function(){ this.hasCelebGirlfriend=true; } ; Player.prototype.fixEyes = function(){ this.wearGlasses=false; }; // Prototype Properties - can be read or written by anyone (or overridden) Player.prototype.wearsGlasses=true; // Static Properties - anyone can read or write Player.noOfPlayers = 0; (function PlayerTest(){ //New instance of the Player object created. var cricketer=new Player("Vivian","Cricket",23,"England"); var golfer =new Player("Pete","Golf",32,"USA"); console.log("So far there are " + Player.noOfPlayers + " in the guild"); //Both these functions share the common 'Player.prototype.wearsGlasses' variable cricketer.fixEyes(); golfer.fixEyes(); cricketer.endorses="Other Brand";//public variable can be updated //Both Player's public method is now changed via their prototype Player.prototype.fixEyes=function(){ this.wearGlasses=true; }; //Only Cricketer's function is changed cricketer.switchHands=function(){ this.batPreference="undecided"; }; })();
Let's understand a few important concepts from this example:
- The
retirementAge
variable is a private variable that has no privileged method to get or set its value. - The
country
variable is a private variable created as a constructor argument. Constructor arguments are available as private variables to the object. - When we called
cricketer.switchHands()
, it was only applied to thecricketer
and not to both the players, although it's a prototype function of thePlayer
itself. - Private functions and privileged methods are instantiated with each new object created. In our example, new copies of
isAvailable()
andbook()
would be created for each new player instance that we create. On the other hand, only one copy of public methods is created and shared between all instances. This can mean a bit of performance gain. If you don't really need to make something private, think about keeping it public.
Inheritance is an important concept of OOP. It is common to have a bunch of objects implementing the same methods. It is also common to have an almost similar object definition with differences in a few methods. Inheritance is very useful in promoting code reuse. We can look at the following classic example of inheritance relation:
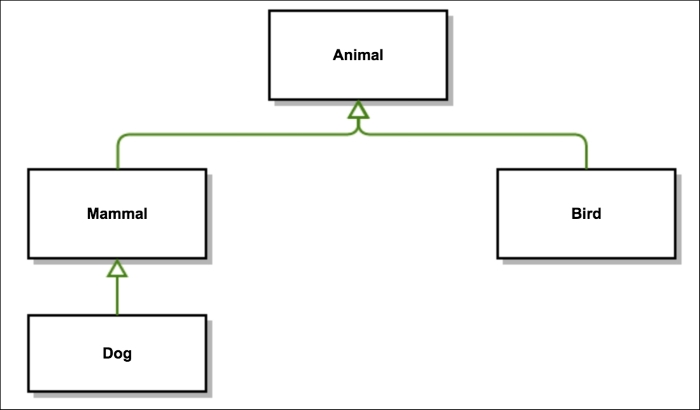
Here, you can see that from the generic Animal class, we derive more specific classes such as Mammal and Bird based on specific characteristics. Both the Mammal and Bird classes do have the same template of an Animal; however, they also define behaviors and attributes specific to them. Eventually, we derive a very specific mammal, Dog. A Dog has common attributes and behaviors from an Animal class and Mammal class, while it adds specific attributes and behaviors of a Dog. This can go on to add complex inheritance relationships.
Traditionally, inheritance is used to establish or describe an IS-A relationship. For example, a dog IS-A mammal. This is what we know as classical inheritance. You would have seen such relationships in object-oriented languages such as C++ and Java. JavaScript has a completely different mechanism to handle inheritance. JavaScript is classless language and uses prototypes for inheritance. Prototypal inheritance is very different in nature and needs thorough understanding. Classical and prototypal inheritance are very different in nature and need careful study.
In classical inheritance, instances inherit from a class blueprint and create subclass relationships. You can't invoke instance methods on a class definition itself. You need to create an instance and then invoke methods on this instance. In prototypal inheritance, on the other hand, instances inherit from other instances.
As far as inheritance is concerned, JavaScript uses only objects. As we discussed earlier, each object has a link to another object called its prototype. This prototype object, in turn, has a prototype of its own, and so on until an object is reached with null
as its prototype; null
, by definition, has no prototype, and acts as the final link in this prototype chain.
To understand prototype chains better, let's consider the following example:
function Person() {} Person.prototype.cry = function() { console.log("Crying"); } function Child() {} Child.prototype = {cry: Person.prototype.cry}; var aChild = new Child(); console.log(aChild instanceof Child); //true console.log(aChild instanceof Person); //false console.log(aChild instanceof Object); //true
Here, we define a Person
and then Child
—a child IS-A person. We also copy the cry
property of a Person
to the cry
property of Child
. When we try to see this relationship using instanceof
, we soon realize that just by copying a behavior, we could not really make Child
an instance of Person
; aChild instanceof Person
fails. This is just copying or masquerading, not inheritance. Even if we copy all the properties of Person
to Child
, we won't be inheriting from Person
. This is usually a bad idea and is shown here only for illustrative purposes. We want to derive a prototype chain—an IS-A relationship, a real inheritance where we can say that child IS-A person. We want to create a chain: a child IS-A person IS-A mammal IS-A animal IS-A object. In JavaScript, this is done using an instance of an object as a prototype as follows:
SubClass.prototype = new SuperClass(); Child.prototype = new Person();
Let's modify the earlier example:
function Person() {}
Person.prototype.cry = function() {
console.log("Crying");
}
function Child() {}
Child.prototype = new Person();
var aChild = new Child();
console.log(aChild instanceof Child); //true
console.log(aChild instanceof Person); //true
console.log(aChild instanceof Object); //true
The changed line uses an instance of Person
as the prototype of Child
. This is an important distinction from the earlier method. Here we are declaring that child IS-A person.
We discussed about how JavaScript looks for a property up the prototype chain till it reaches Object.prototype
. Let's discuss the concept of prototype chains in detail and try to design the following employee hierarchy:
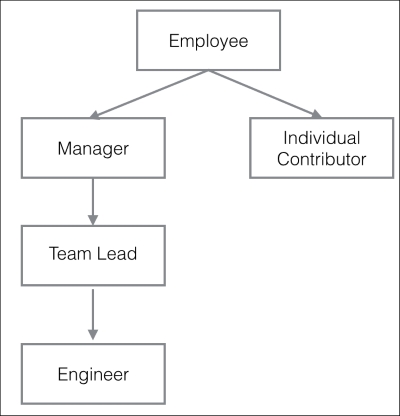
This is a typical pattern of inheritance. A manager IS-A(n) employee. Manager has common properties inherited from an Employee. It can have an array of reportees. An Individual Contributor is also based on an employee but he does not have any reportees. A Team Lead is derived from a Manager with a few functions that are different from a Manager. What we are doing essentially is that each child is deriving properties from its parent (Manager being the parent and Team Lead being the child).
Let's see how we can create this hierarchy in JavaScript. Let's define our Employee
type:
function Employee() { this.name = ''; this.dept = 'None'; this.salary = 0.00; }
There is nothing special about these definitions. The Employee
object contains three properties—name, salary, and department. Next, we define Manager
. This definition shows you how to specify the next object in the inheritance chain:
function Manager() { Employee.call(this); this.reports = []; } Manager.prototype = Object.create(Employee.prototype);
In JavaScript, you can add a prototypical instance as the value of the prototype property of the constructor function. You can do so at any time after you define the constructor. In this example, there are two ideas that we have not explored earlier. First, we are calling Employee.call(this)
. If you come from a Java background, this is analogous to the super()
method call in the constructor. The call()
method calls a function with a specific object as its context (in this case, it is the given the this
value), in other words, call allows to specify which object will be referenced by the this
keyword when the function will be executed. Like super()
in Java, calling parentObject.call(this)
is necessary to correctly initialize the object being created.
The other thing we see is Object.create()
instead of calling new
. Object.create()
creates an object with a specified prototype. When we do new Parent()
, the constructor logic of the parent is called. In most cases, what we want is for Child.prototype
to be an object that is linked via its prototype to Parent.prototype
. If the parent constructor contains additional logic specific to the parent, we don't want to run this while creating the child object. This can cause very difficult-to-find bugs. Object.create()
creates the same prototypal link between the child and parent as the new
operator without calling the parent constructor.
To have a side effect-free and accurate inheritance mechanism, we have to make sure that we perform the following:
- Setting the prototype to an instance of the parent initializes the prototype chain (inheritance); this is done only once (as the prototype object is shared)
- Calling the parent's constructor initializes the object itself; this is done with every instantiation (you can pass different parameters each time you construct it)
With this understanding in place, let's define the rest of the objects:
function IndividualContributor() { Employee.call(this); this.active_projects = []; } IndividualContributor.prototype = Object.create(Employee.prototype); function TeamLead() { Manager.call(this); this.dept = "Software"; this.salary = 100000; } TeamLead.prototype = Object.create(Manager.prototype); function Engineer() { TeamLead.call(this); this.dept = "JavaScript"; this.desktop_id = "8822" ; this.salary = 80000; } Engineer.prototype = Object.create(TeamLead.prototype);
Based on this hierarchy, we can instantiate these objects:
var genericEmployee = new Employee(); console.log(genericEmployee);
You can see the following output for the preceding code snippet:
[object Object] { dept: "None", name: "", salary: 0 }
A generic Employee
has a department assigned to None
(as specified in the default value) and the rest of the properties are also assigned as the default ones.
Next, we instantiate a manager; we can provide specific values as follows:
var karen = new Manager(); karen.name = "Karen"; karen.reports = [1,2,3]; console.log(karen);
You will see the following output:
[object Object] { dept: "None", name: "Karen", reports: [1, 2, 3], salary: 0 }
For TeamLead
, the reports
property is derived from the base class (Manager in this case):
var jason = new TeamLead(); jason.name = "Json"; console.log(jason);
You will see the following output:
[object Object] { dept: "Software", name: "Json", reports: [], salary: 100000 }
When JavaScript processes the new operator, it creates a new object and passes this object as the value of this
to the parent—the TeamLead
constructor. The constructor function sets the value of the projects
property and implicitly sets the value of the internal __proto__
property to the value of TeamLead.prototype
. The __proto__
property determines the prototype chain used to return property values. This process does not set values for properties inherited from the prototype chain in the jason
object. When the value of a property is read, JavaScript first checks to see whether the value exists in that object. If the value does exist, this value is returned. If the value is not there, JavaScript checks the prototype chain using the __proto__
property. Having said this, what happens when you do the following:
Employee.prototype.name = "Undefined";
It does not propagate to all the instances of Employee
. This is because when you create an instance of the Employee
object, this instance gets a local value for the name. When you set the TeamLead
prototype by creating a new Employee
object, TeamLead.prototype
has a local value for the name
property. Therefore, when JavaScript looks up the name
property of the jason
object, which is an instance of TeamLead
), it finds the local value for this property in TeamLead.prototype
. It does not try to do further lookups up the chain to Employee.prototype
.
If you want the value of a property changed at runtime and have the new value be inherited by all the descendants of the object, you cannot define the property in the object's constructor function. To achieve this, you need to add it to the constructor's prototype. For example, let's revisit the earlier example but with a slight change:
function Employee() { this.dept = 'None'; this.salary = 0.00; } Employee.prototype.name = ''; function Manager() { this.reports = []; } Manager.prototype = new Employee(); var sandy = new Manager(); var karen = new Manager(); Employee.prototype.name = "Junk"; console.log(sandy.name); console.log(karen.name);
You will see that the name
property of both sandy and karen has changed to Junk
. This is because the name
property is declared outside the constructor function. So, when you change the value of name
in the Employee's prototype, it propagates to all the descendants. In this example, we are modifying Employee's prototype after the sandy
and karen
objects are created. If you changed the prototype before the sandy
and karen
objects were created, the value would still have changed to Junk
.
All native JavaScript objects—Object, Array, String, Number, RegExp, and Function—have prototype properties that can be extended. This means that we can extend the functionality of the language itself. For example, the following snippet extends the String
object to add a reverse()
method to reverse a string. This method does not exist in the native String object but by manipulating String's prototype, we add this method to String:
String.prototype.reverse = function() { return Array.prototype.reverse.apply(this.split('')).join(''); }; var str = 'JavaScript'; console.log(str.reverse()); //"tpircSavaJ"
Though this is a very powerful technique, care should be taken not to overuse it. Refer to http://perfectionkills.com/extending-native-builtins/ to understand the pitfalls of extending native built-ins and what care should be taken if you intend to do so.
Getters are convenient methods to get the value of specific properties; as the name suggests, setters are methods that set the value of a property. Often, you may want to derive a value based on some other values. Traditionally, getters and setters used to be functions such as the following:
var person = { firstname: "Albert", lastname: "Einstein", setLastName: function(_lastname){ this.lastname= _lastname; }, setFirstName: function (_firstname){ this.firstname= _firstname; }, getFullName: function (){ return this.firstname + ' '+ this.lastname; } }; person.setLastName('Newton'); person.setFirstName('Issac'); console.log(person.getFullName());
As you can see, setLastName()
, setFirstName()
, and getFullName()
are functions used to do get and set of properties. Fullname
is a derived property by concatenating the firstname
and lastname
properties. This is a very common use case and ECMAScript 5 now provides you with a default syntax for getters and setters.
The following example shows you how getters and setters are created using the object literal syntax in ECMAScript 5:
var person = { firstname: "Albert", lastname: "Einstein", get fullname() { return this.firstname +" "+this.lastname; }, set fullname(_name){ var words = _name.toString().split(' '); this.firstname = words[0]; this.lastname = words[1]; } }; person.fullname = "Issac Newton"; console.log(person.firstname); //"Issac" console.log(person.lastname); //"Newton" console.log(person.fullname); //"Issac Newton"
Another way of declaring getters and setters is using the Object.defineProperty()
method:
var person = { firstname: "Albert", lastname: "Einstein", }; Object.defineProperty(person, 'fullname', { get: function() { return this.firstname + ' ' + this.lastname; }, set: function(name) { var words = name.split(' '); this.firstname = words[0]; this.lastname = words[1]; } }); person.fullname = "Issac Newton"; console.log(person.firstname); //"Issac" console.log(person.lastname); //"Newton" console.log(person.fullname); //"Issac Newton"
In this method, you can call Object.defineProperty()
even after the object is created.
Now that you have tasted the object-oriented flavor of JavaScript, we will go through a bunch of very useful utility methods provided by Underscore.js. We discussed the installation and basic usage of Underscore.js in the previous chapter. These methods will make common operations on objects very easy:
keys()
: This method retrieves the names of an object's own enumerable properties. Note that this function does not traverse up the prototype chain:var _ = require('underscore'); var testobj = { name: 'Albert', age : 90, profession: 'Physicist' }; console.log(_.keys(testobj)); //[ 'name', 'age', 'profession' ]
allKeys()
: This method retrieves the names of an object's own and inherited properties:var _ = require('underscore'); function Scientist() { this.name = 'Albert'; } Scientist.prototype.married = true; aScientist = new Scientist(); console.log(_.keys(aScientist)); //[ 'name' ] console.log(_.allKeys(aScientist));//[ 'name', 'married' ]
values()
: This method retrieves the values of an object's own properties:var _ = require('underscore'); function Scientist() { this.name = 'Albert'; } Scientist.prototype.married = true; aScientist = new Scientist(); console.log(_.values(aScientist)); //[ 'Albert' ]
mapObject()
: This method transforms the value of each property in the object:var _ = require('underscore'); function Scientist() { this.name = 'Albert'; this.age = 90; } aScientist = new Scientist(); var lst = _.mapObject(aScientist, function(val,key){ if(key==="age"){ return val + 10; } else { return val; } }); console.log(lst); //{ name: 'Albert', age: 100 }
functions()
: This returns a sorted list of the names of every method in an object—the name of every function property of the object.pick()
: This function returns a copy of the object, filtered to just the values of the keys provided:var _ = require('underscore'); var testobj = { name: 'Albert', age : 90, profession: 'Physicist' }; console.log(_.pick(testobj, 'name','age')); //{ name: 'Albert', age: 90 } console.log(_.pick(testobj, function(val,key,object){ return _.isNumber(val); })); //{ age: 90 }
omit()
: This function is an invert ofpick()
—it returns a copy of the object, filtered to omit the values for the specified keys.
JavaScript applications can improve in clarity and quality by allowing for the greater degree of control and structure that object-orientation can bring to the code. JavaScript object-orientation is based on the function prototypes and prototypal inheritance. These two ideas can provide an incredible amount of wealth to developers.
In this chapter, we saw basic object creation and manipulation. We looked at how constructor functions are used to create objects. We dived into prototype chains and how inheritance operates on the idea of prototype chains. These foundations will be used to build your knowledge of JavaScript patterns that we will explore in the next module. We will discuss various testing and debugging techniques in the next chapter.