First, let's look at how we maintain state in our Angular application. Since Angular apps are dynamic and not static, we need to understand the mechanisms that are used to make sure that these dynamic values are kept up to date as the data in an application gets updated. For example, in our application, how does the number of guesses get updated on the screen? How does the application decide to display the correct message about whether the guess is correct based on the user input?
Since we have been emphasizing so far that Angular uses the component design pattern, you will probably not be surprised to know that the basic container for the application state is the component itself. This means that when we have a component instance, all the properties in the component and their values are available for the template instance that is referenced in the component. At a practical level, this means that we can use these values directly in expressions and bindings in the template without having to write any plumbing code to wire them up.
In the sample app, for example, to determine what message to display, we can use deviation
directly in the template expression. Angular will scan our component to find a property with that name and use its value. The same is true for noOfTries
; Angular will look for the value of this property within our component and then use it to set its value in the interpolation within the template. We don't have to write any other code:
<div> <p *ngIf="deviation<0" class="alert alert-warning"> Your guess is higher.</p> <p *ngIf="deviation>0" class="alert alert-warning"> Your guess is lower.</p> <p *ngIf="deviation===0" class="alert alert-success"> Yes! That's it.</p></div> <p class="text-info">No of guesses : <span class="badge">{{noOfTries}}</span> </p> </div>
So how does Angular keep track of changes in our component as it runs? So far, it appears as if this is all done by magic. We just set up our component properties and methods, and then we bind them to the view using interpolation along with property and event binding. Angular does the rest!
But this does not happen by magic, of course, and in order to make effective use of Angular, you need to understand how it updates these values as they change. This is called change detection, and Angular has a very different approach to doing this than what previously existed.
If you use the debugger tool in your browser to walk through the application, you will see how change detection works. Here, we are using Chrome's Developer tools and setting a watch for the noOfTries
property. If you place a breakpoint at the end of the verifyGuess()
method, you will see that when you enter a guess, the noOfTries
property is first updated as soon as you hit the breakpoint, as follows:
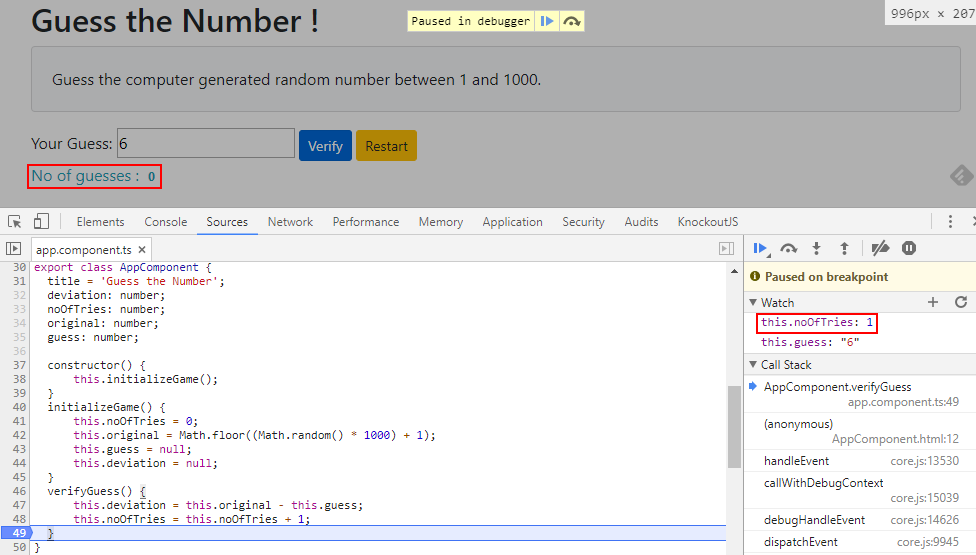
Once you move past the breakpoint, the display on the screen updates with the correct number of guesses, as seen in the following screenshot:
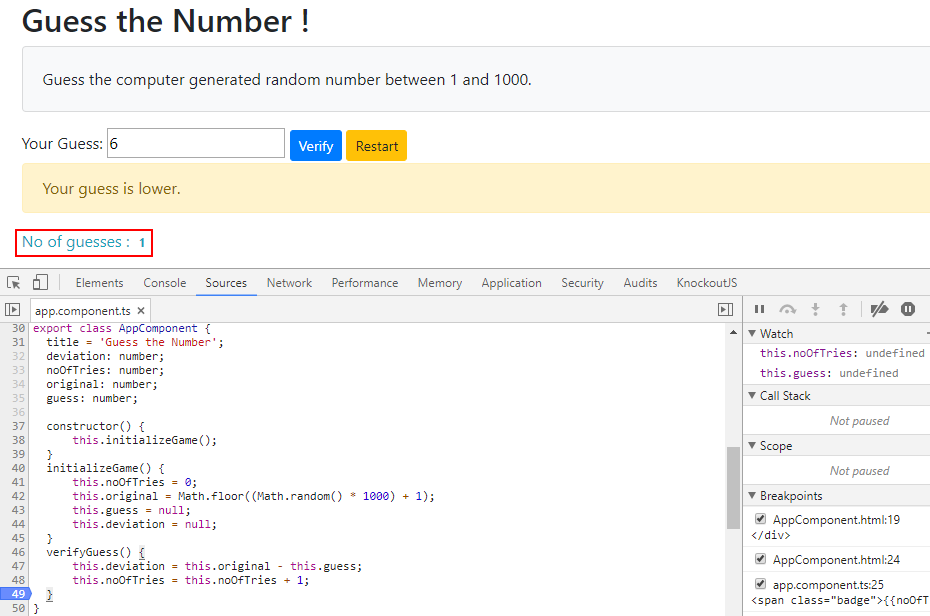
What is really going on under the hood is that Angular is reacting to events in the application and using change detectors, which go through every component, to determine whether anything has changed that affects the view. In this case, the event is a button click. The event generated by the button click calls the verifyGuess()
method on the component that updates the noOfTries
property.
That event triggers the change detection cycle, which identifies that the noOfTries
property that is being used in the view has changed. As a result, Angular updates the element in the view that is bound to noOfTries
with the new value of that property.
As you can see, this is a multistep process where Angular first updates the components and domain objects in response to an event, then runs change detection, and finally rerenders elements in the view that have changed. And, it does this on every browser event (as well as other asynchronous events, such as XHR requests and timers). Change detection in Angular is reactive and one way.
This approach allows Angular to make just one pass through the change detection graph. It is called one-way data binding, and it vastly improves the performance of Angular.
Note
We'll be covering Angular change detection in depth in Chapter 8, Some Practical Scenarios. For a description of this process by the Angular team, visit https://vsavkin.com/two-phases-of-angular-2-applications-fda2517604be#.fabhc0ynb.