Working with Julia
In this section, we will set up a standard Julia development environment, and learn how to work with Julia scripts as well as the Read–Eval–Print Loop (REPL). The REPL allows you to work with Julia in an interactive way, trying out expressions, function calls, and even executing whole programs.
You’re good to go when typing in julia
at the terminal starts up the REPL:
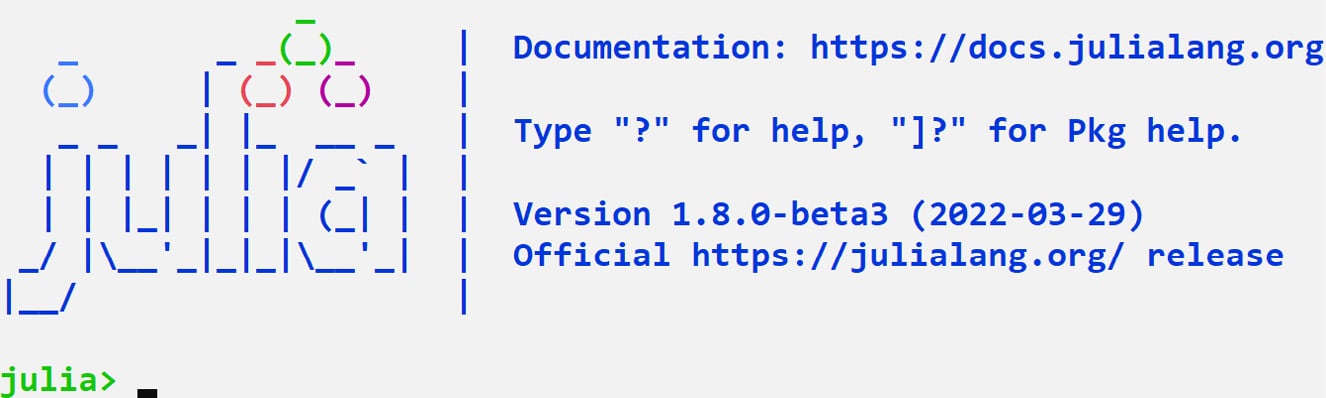
Figure 1.1 – The Julia REPL
Using the REPL to use Julia interactively
Try typing in 256^2
, giving the result 65536
, or rand()
, which gives you a random number between 0
and 1
, for example, 0.02925477322848513
. We’ll use the REPL extensively throughout the book, and the Genie web framework discussed in Part 2 allows you to build your entire web app from the REPL.
Some useful REPL keyboard shortcuts you will use often include the following:
- Ctrl + D: To exit the REPL
- Ctrl + L: To clear the screen
- Ctrl + C: To get a new prompt
- Up and down arrows: To reuse recent commands
To see which folder you are in, type pwd()
. To change the current folder, execute cd("path/to/folder")
.
A feature we’ll use a lot in Genie is creating new files from within the REPL with the touch
command. For example, to create an empty testset.jl
file in an existing folder structure, testing/phase1
, enter the following:
julia> touch(joinpath("testing", "phase1", "testset.jl"))
The REPL returns testing\\phase1\\testset.j
l on Windows and testing/phase1/testset.jl
on *nix systems.
The joinpath
function constructs the directory path starting in the current folder, and touch
creates the file.
Using the package mode to jump-start a project
The Julia ecosystem encompasses thousands of libraries, called packages (see https://julialang.org/packages/), for which Julia has a built-in package manager, Pkg.
The REPL has a special mode for working with packages, which is started by typing ]
at julia> prompt
, which brings you to package mode: (@
v1.8) pkg>
.
Some useful commands in this mode to type in after the pkg>
prompt are as follows:
st
orstatus
: Gets a list of all the packages installed in your environment.add PackageName
: Adds a new package (you can add several packages separated by,
if needed).up or update
: Updates all your packages.up or update PackageName
: Updates a specific package.activate .
: Activates the current project environment (see the Packages and projects section under Using Julia modules and packages).rm or remove PackageName
: Removes a specific package.?
: Lists all available commands.- The backspace key: Exits the
pkg>
mode.
In the next section, Parsing a CSV file, we will work with a comma-separated values (CSV) file of to-do items. The CSV package can be imported and set up to be used in your Julia REPL by typing the following:
julia> using Pkg julia> Pkg.add("CSV") julia> using CSV
The last line of the preceding command brings the definitions of the CSV package into scope.
Alternatively, from the package mode, use the following:
]: (@v1.8) pkg> add CSV
The preceding command installs all packages CSV depends on and then precompiles them. This way, the project gets a jump-start, because the just-in-time (JIT) compiler doesn’t have to do this work anymore.
Using Julia with the VS Code plugin
Julia code can also be saved and edited in files with a .jl
extension. Numerous IDEs exist to do that. In this book, we’ll use the VS Code platform with the excellent Julia plugin, which provides syntax highlighting and completion, lookup definitions, and plotting among many other features.
A handy way to start VS Code from the terminal prompt is by typing in code
.
Search in the Extensions tab for Julia and install it. Then, open up a new file, and type in println("Hi Web World from Julia!")
, and save it as hiweb.jl
.
Run the program in VS Code with F5 to see the string printed out. Or start the REPL and type the following to get the same result:
julia> include("hiweb.jl")
include
evaluates the contents of the input source file.
Or, from a terminal prompt, execute a Julia source file simply by typing the following:
julia hiweb.jl
In all cases, the output will be Hi Web World
from Julia!
The include
command loads in the Julia file and executes the code.
Continuing the example from the previous section, if you want to start editing the newly created testset.jl
file in VS Code when you are working in the REPL, simply type the following:
julia> edit(joinpath("testing", "phase1", "testset.jl"))
Now that you have some idea of working with Julia using the REPL, in package mode, and using VS Code, let us dig deeper into understanding the basics of the language. In the next section, we will explore some of the basic types, flow controls, functions, and methods in Julia.