Understanding and using databases
In most web applications, data forms the core part of the application. Unless we’re talking about a very simple application such as a calculator, in most cases, we need to store data, process it, and display it to the user on a page. Since most operations in user-facing web applications involve data, there is a need to store data somewhere secure, easily accessible, and readily available. This is where databases come in handy. Imagine a library operation before the advent of computers. The librarian would have to maintain records of book inventories, book lending, returns from students, and so on. All of these would have been maintained in physical records. While carrying out their day-to-day activities, the librarian would modify these records for each operation, for example, when lending a book to someone or when the book was returned.
Today, we have databases to help us with such administrative tasks. A database looks like a spreadsheet or an Excel sheet containing records, with each table consisting of multiple rows and columns. An application can have many such tables. Here is an example table of a book inventory in a library:
Book Number |
Author |
Title |
Number of Copies |
Howto4563 |
Adam Chappel |
How to Build a House |
4 |
Travel5327 |
Charlie Hunt |
How to Holiday in Switzerland |
5 |
Fiction3453 |
Evan Stark |
The Mystery Cat |
2 |
Howto4453 |
Bruce Williams |
Sailing Guide |
7 |
Table 2.1: Table of a book inventory for a library
In the preceding table, we can see that there are columns with details about various attributes of the books in the library, while the rows contain entries for each book. To manage a library, there can be many such tables working together as a system. For example, along with an inventory, we may have other tables such as student information, book lending records, and so on. Databases are built with the same logic, where software applications can easily manage data.
A database is a structured collection of data that helps manage information easily. A software layer called the database management system (DBMS) is used to store, maintain, and perform operations on the data. There are two types of databases, relational databases, and non-relational databases. In the following sections, we will learn in brief about relational databases and how data is stored in such databases.
Relational databases
Relational databases or structured query language (SQL) databases store data in a pre-determined structure of rows and columns called tables. A database can be made up of more than one such table, and these tables have a fixed structure of attributes, data types, and relations with other tables. For example, as we just saw in Figure 2.1, the book inventory table has a fixed structure of columns comprising Book Number, Author, Title, and Number of Copies, and the entries form the rows in the table. There could be other tables as well, such as Student Information and Lending Records, which could be related to the inventory table. Also, whenever a book is lent to a student, the records will be stored per the relationships between multiple tables (say, the Student Information and the Book Inventory tables).
This pre-determined structure of rules defining the data types, tabular structures, and relationships across different tables acts like scaffolding or a blueprint for a database. This blueprint is collectively called a database schema. It will prepare the database to store application data when applied to a database. To manage and maintain these databases, there is a common language for relational databases called SQL. Some examples of relational databases are SQLite, PostgreSQL, MySQL, and OracleDB.
In the next section, you will be introduced to non-relational databases, some examples of non-relational databases, and the reasons for using them in web applications.
Non-relational databases
Non-relational databases or not only SQL (NoSQL) databases are designed to store unstructured data. They are well suited to large amounts of generated data that does not follow rigid rules, as is the case with relational databases. Some examples of non-relational databases are Cassandra, MongoDB, CouchDB, and Redis.
For example, imagine that you need to store the stock value of companies in a database using Redis. Here, the company name will be stored as the key and the stock value as the value. Using the key-value type NoSQL database in this use case is appropriate because it stores the desired value for a unique key and is faster to access.
For the scope of this book, we will be dealing only with relational databases, as Django does not officially support non-relational databases. However, if you wish to explore, there are many forked projects, such as Django non-rel, that support NoSQL databases.
In the next section, you will be introduced to database CRUD operations using SQL commands.
Database operations using SQL
SQL uses a set of commands to perform a variety of database operations, such as creating an entry, reading values, updating an entry, and deleting an entry. These operations are collectively called CRUD operations. To understand database operations in detail, let’s first get some hands-on experience with SQL commands. Most relational databases share a similar SQL syntax; however, some operations will differ.
For the scope of this chapter, we will use SQLite as the database. SQLite is a lightweight relational database and is a part of Python’s standard libraries. That’s why Django uses SQLite as its default database configuration. However, we will also learn more about how to perform configuration changes to use other databases in Chapter 17, Deploying a Django Application (Part 1 – Server Setup). This chapter can be downloaded from the GitHub repository of this book, here: https://github.com/PacktPublishing/Web-Development-with-Django-Second-Edition/tree/main/Chapter17.
In the next section, we will learn about the importance of data types in a database.
Data types in relational databases
Databases provide us with a way to restrict the type of data that can be stored in a given column. These are called data types. Some examples of data types for a relational database, such as SQLite3, are given here:
INTEGER
: This is used for storing integersTEXT
: This can store textREAL
: This is used for floating-point values
For example, you would want the title of a book to have TEXT
as the data type. So, the database will enforce a rule that no type of data, other than text data, can be stored in that column. Similarly, the book’s price can have a REAL
data type, and so on.
Using some of the concepts learned before about databases, in the next section, we will get hands-on by creating a database and a database table.
Exercise 2.01 – creating a book database
In this exercise, you will create a book database for a book review application. For better visualization of the data in the SQLite database, you will install an open source tool called DB Browser for SQLite. This tool helps visualize the data and provides a shell to execute the SQL commands.
If you haven’t done so already, visit https://sqlitebrowser.org, and from the Download section, install the application as per your operating system and launch it. Detailed instructions for DB Browser installation can be found in the Preface. To create a database, follow these steps:
Note
Database operations can be performed using a command-line shell as well.
- After launching the application, create a new database by clicking New Database in the top-left corner of the application. Create a database named
bookr
, as you are working on a book review application:
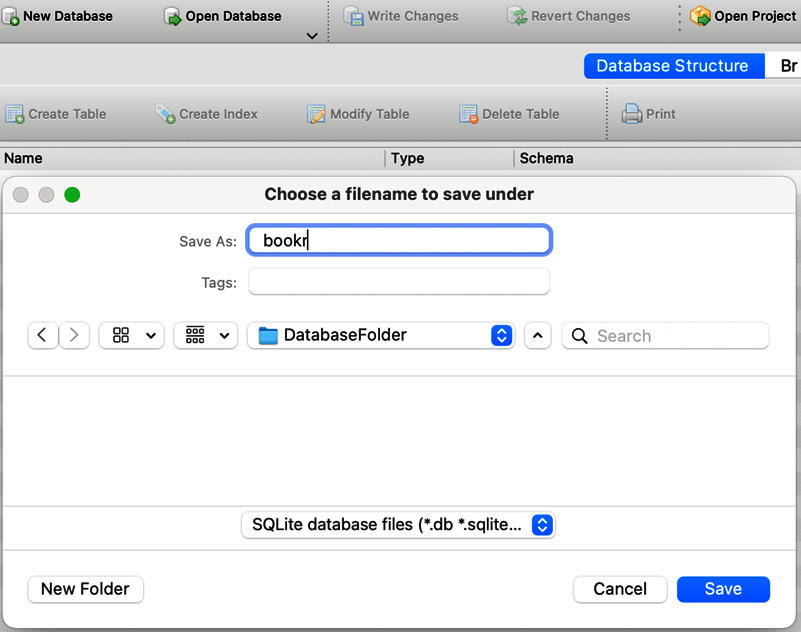
Figure 2.1 – Creating a database named bookr
- Next, click on the Create Table button in the top-left corner and enter
book
as the table name.
Note
After clicking the Save button, you may find that the window for creating a table opens up automatically. In that case, you won’t have to click the Create Table button; simply proceed with the creation of the book
table as specified in the preceding step.
- Now, click the Add field button, enter the field name as
title
and select the TEXT type from the drop-down menu. Here, TEXT is the data type for thetitle
field in the database:
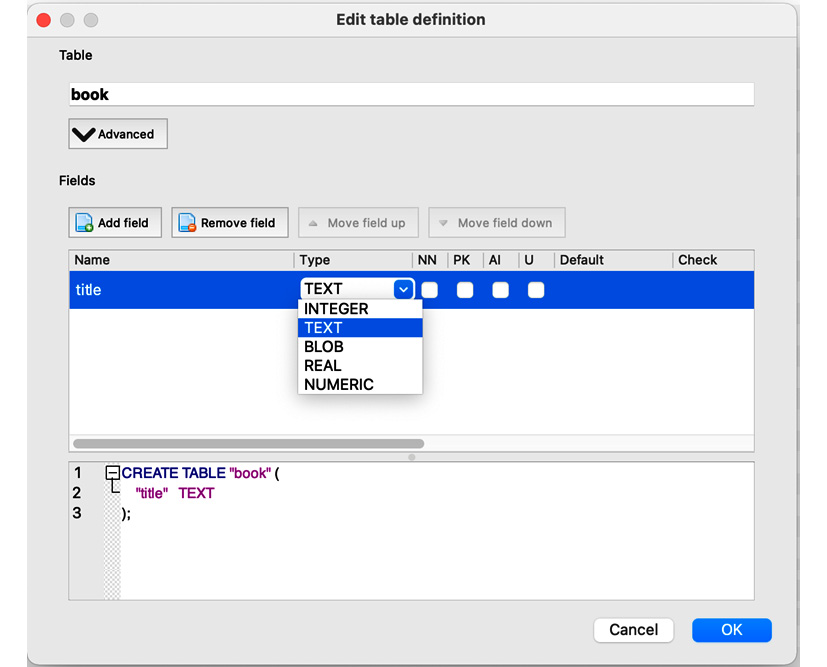
Figure 2.2: Adding a TEXT field named title
- Similarly, add two more fields for the table named publisher and author and select the TEXT type for both fields. Then, click on the OK button:
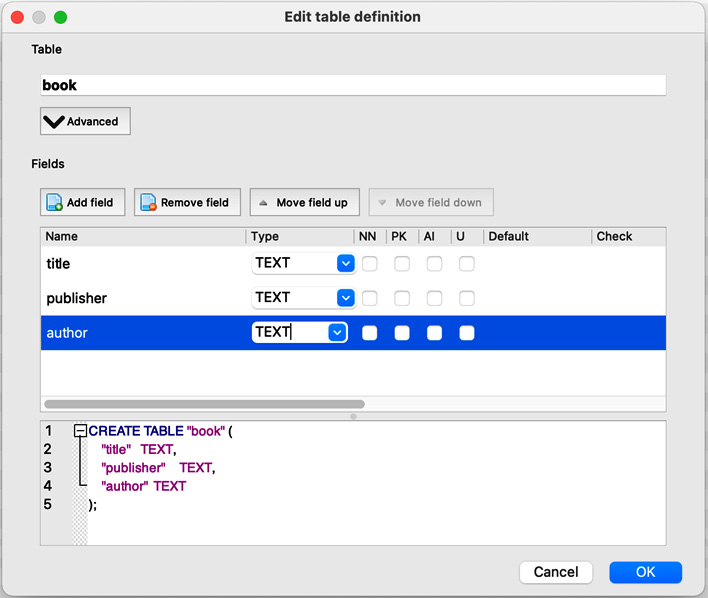
Figure 2.3: Creating TEXT fields named publisher and author
This creates a database table called book
in the bookr
database with the title
, publisher
, and author
fields. This can be seen as follows:
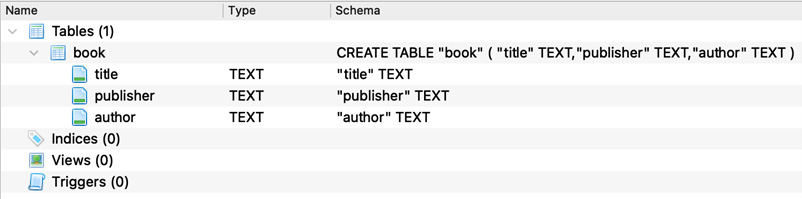
Figure 2.4: Database with the title, publisher, and author fields
Overall, in this section about databases, we have learned about why we need databases and briefly about how they store data, the two most common types of databases. Additionally, we created a simple database and a table using an open source tool called DB Browser (SQLite). In the next section, we will learn more about how to do CRUD operations on the database using SQl.