Adding mock data to your database
Sample data is a key part of any development process. The benefits of using mock data in our application are clear:
- It provides a better overview of the application
- It saves testing time as you do not need to introduce the data yourself in the app
- It helps you identify errors in your application as you will see the application working sooner
- When you release the app, you can use this mock data to feed your test environments
As mock data does not need to be real or accurate, you may just create some random sentences and copy them into a .csv
file. Still, there is a better alternative and you should aim to have useful mock data. Here are some tips you may follow:
- Try to find data that makes sense to your context. For instance, if you have to fill in a phone number, do not add random numbers. Instead, use a number with 9 digits that match your phone number format.
- Do not use real information as your mock data. It might be leaked and you do not want to expose your family and friends.
- Try to automate the process as much as possible.
- You probably need as many types of mock data, as entities you have.
So, keeping these tips in mind, we are going to create a little script that helps us generate our mock data.
Defining the content of our mock data
For each of our entities, we’ll generate a CSV file with sample data. We will import this data into our recently created tables, so we need it to have a specific structure.
For each entity, we will have the following columns (one per field):
- Customers: Firstname, lastname, email, company name, company ID, company address, and phone
- Invoices: Services provided and amount
- Projects: Name, description, and a deadline
- Tasks: Name, description, state, and deadline
Automating mock data generation
It’s a good idea to have more than one sample item per entity in our component. The more items you have, the more cases you can test and notice when developing your component. Also, having random items helps you discover issues and errors in an early phase. You may create sample items one by one by hand, but that will be a repetitive, boring task prone to human error. Instead, we are going to automate the creation of sample data using a simple PHP script.
As we are developing for Joomla! and we love PHP (also, since most of the Joomla! coding is done in PHP, it makes sense not to introduce another language), we are going to code a small PHP script. This script will use a Faker PHP library to automate the process of creating the data. You can find more information about Faker in the Further reading section at the end of this chapter.
The Faker PHP library can create fake data for several type fields and it’s very popular in the PHP community.
Let’s create a folder in our repository called Mock
and copy this composer.json
file:
{ "name": "project-manager/mock-data", "description": "A script to generate mock data for the project", "type": "project", "license": "GPL v2", "autoload": { "psr-4": { "Carcam\\MockData\\": "src/" } }, "require": { "fakerphp/faker": "^1.19" } }
This composer.json
file defines our project and tells Composer that we want to use the Faker PHP library in it. After that, we must execute composer install
in our folder and create the create-mock-customers.php
script. In this file, we start loading the Composer autoloader file. This allows us to use namespaces in our script. If you are not familiar with Composer, I recommend that you check out the relevant link in the Further reading section of this chapter:
<?php require_once 'vendor/autoload.php';
Then, we must define the headers of the CSV file and the number of lines of data we want to create.
How much fake data should I create?
For the number of lines in our CSV, we use a number bigger than 20. In Joomla!, by default, we have 20 items per page, so this helps us show at least two pages of items in the listings.
We must also define the path to save the resulting file before we create the data:
$headers = ['firstname', 'lastname', 'email', 'company_name', 'company_id', 'company_address', 'phone']; $totalLines = 30; $filepath = './data/customer.csv';
Once we have set up the script, we must initialize the Faker
class and start creating our CSV file in a for
loop. Inside this for
loop, we must initialize an array to store each column and add the values obtained from the Faker
class. Finally, we must assign the $data
array to the CSV line:
$faker = Faker\Factory::create('en_US'); $csv[0] = $headers; for ($line = 0; $line < $totalLines – 1; $line++) { $data = array(); $data[] = $faker->firstName(); $data[] = $faker->lastName(); $data[] = $faker->email(); $data[] = $faker->company(); $data[] = $faker->ein(); $data[] = $faker->address(); $data[] = $faker->phoneNumber(); $csv[$line] = $data; }
As soon as we finish adding the lines to the data array, we must create the file that will store this data. First, we must create and open the file; then, we must go through our $csv
array with a loop to write down every line of the .
csv
file:
if (!file_exists(dirname($filepath))) { mkdir(dirname($filepath), 0755); } $file = fopen($filepath, 'w'); foreach ($csv as $row) { fputcsv($file, $row); } fclose($file);
The process for creating the other entities’ mock data files is similar, so I leave it up to you to check the code in this book’s GitHub repository.
Importing mock data into the database
Once we have created our mock data, we need to add it to our database to be able to use it or retrieve it from our component.
At this point, we are going to import the data manually. However, later in this book, we will learn how we can include it in the installation files, though I do not recommend this approach.
Note
Adding sample data in your installation files will install this data every time your user installs the component. This might be interesting for first-time users, but it is annoying for users who are already acquainted with the component.
To begin importing, follow these steps:
- To import data into your database, you need to access phpMyAdmin or a similar tool and go to the #__spm_customers table we created in the previous section.
- Then, click on Import, add your CSV file, and click on Import:
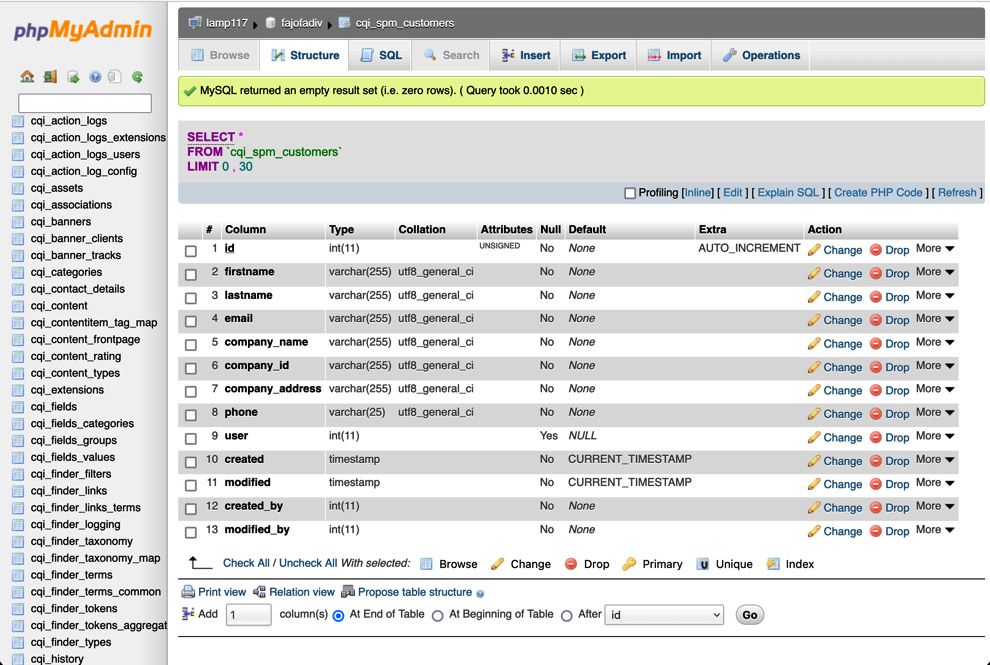
Figure 1.8 – The #__spm_customers table with the Import button at the top right
Now, our database is full of mock data and we are ready to start coding our component to see the results of this import.