We are going to download GWT and its prerequisites, install them to the hard disk, and then run one of the sample applications shipped with the GWT distribution to ensure that it works correctly.
In order to use the GWT, you will need to have Java SDK installed. If you do not already have the Java SDK, you can download the latest version from http://java.sun.com/javase/downloads/. Install the SDK using the instructions provided by the download for your platform.
Note
Java 1.4.2 is the safest version of Java to use with GWT, as it is completely compatible with this version, and you can be sure that your application code will compile correctly. GWT also works with the two newer versions of the Java platform—1.5 and 1.6; however, you will not be able to use any of the newer features of the Java language introduced in these versions in your GWT application code
Now, you are ready to download GWT:
1. GWT is available for Windows XP/2000, Linux, and Mac OS X platforms from the GWT download page (http://code.google.com/webtoolkit/download.html). This download includes the GWT compiler, hosted web browser, GWT class libraries, and several sample applications.
Please read the Terms and Conditions of usage before downloading it. The latest version available is 1.3 RC 1, released December 12, 2006. Select the file for your platform. Here is a sample window showing the versions available for GWT:
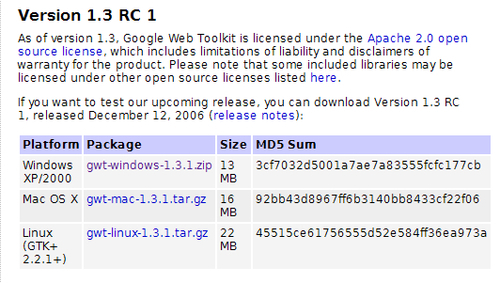
2. Unzip the downloaded GWT distribution to your hard disk. It will create a directory named
gwt-windows-xxx
on Windows andgwt-linux-xxx
on Linux, wherexxx
is the version number of the downloaded distribution. We will refer to the directory that contains the unzipped distribution asGWT_HOME
. TheGWT_HOME
directory contains asamples
folder with seven applications.3. In order to ensure that the GWT is correctly installed, run the
Hello
sample application for your platform by executing the startup script for your platform (the executable scripts for Windows have the extension.cmd
and the ones for Linux have the extension.sh
).Execute the
Hello-shell
script for your platform. Here is a screenshot of theHello
application running successfully in the hosted GWT browser:
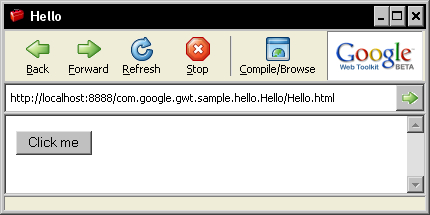
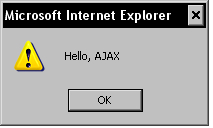
The GWT_HOME
directory contains all the scripts, files, and libraries needed for GWT development, which are as follows:
doc:
This directory contains the API documentation for the various GWT classes. The API documentation is provided in two formats—the Google custom format and the familiarjavadoc
format.samples:
A directory that contains the sample applications.gwt-*.jar:
These are the Java libraries that contain the GWT classes.index.html:
This file is used as Readme for the GWT. It also provides a starting point for the GWT documentation along with pointers to other sources of information.gwt-ll.dll
andswt-win32-3235.dll:
These are Windows' shared libraries (Windows only).libgwt-11.so, libswt-gtk-3235.so, libswt-mozilla17-profile-gcc3-gtk-3235.so, libswt-mozilla17-profile-gtk-3235.so, libswt-mozilla-gcc3-gtk-3235.so, libswt-mozilla-gtk-3235.so
, andlibswt-pi-gtk-3235.so:
These are Linux shared libraries (Linux only).applicationCreator:
This is a Script file for creating a new application.junitCreator:
This is a Script file for creating a new JUnit test.projectCreator:
This is a Script file for creating a new project.i18nCreator:
This is a Script file for creating internationalization scripts.
When you executed Hello-shell.cmd
, you started up the GWT development shell and provided the Hello.html
file as a parameter to it. The development shell then launched a special hosted web browser and displayed the Hello.html
file in it. The hosted web browser is an embedded SWT web browser that has hooks into the Java Virtual Machine (JVM). This makes it possible to debug the Java code for the application, using a Java development environment such as Eclipse.
Here is a screenshot of the development shell that starts up first:
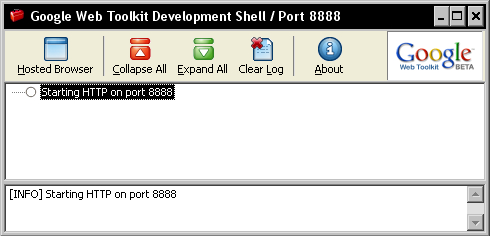
You can customize several of the options provided to the GWT development shell on startup. Run the development shell, from a command prompt, in the GWT_HOME
directory to see the various options available:
@java -cp "gwt-user.jar;gwt-dev-windows.jar" com.google.gwt.dev. GWTShell help
You will see a screen similar to this one:
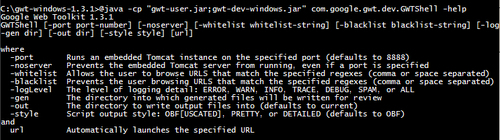
If you want to try out different settings, such as a different port numbers, you can modify the Hello-shell.cmd
file to use these options.
The Linux version of GWT contains 32-bit SWT library bindings that are used by the hosted web browser. In order to run the samples or use the GWT hosted browser on a 64-bit platform such as AMD64, you need to do the following:
Use a 32-bit JDK with 32-bit binary compatibility enabled.
Set the environment variable
LD_LIBRARY_PATH
to the Mozilla directory in your GWT distribution, before starting the GWT shell.