For this task, we will focus on enhancing the visual appeal of the game by introducing and demonstrating the necessary steps required to animate the player sprite. These steps will involve adjusting the game framework to handle the loading and updating animated sprite sheets.
To begin with, we must introduce a new object to the games framework. This object will be responsible for animating the player sprite through means of a sprite sheet.
Create a new object called
AnimationManager
. With the animation object open, copy the following code in it:function AnimationManager() { this.currentFrame = 0; this.frameRate = 0; this.frameTime = 0; this.frameWidth = 0; this.frameHeight = 0; this.InitAnimationManager = function(texture, x, y, z, frameCount, framesPerSec) { this.InitDrawableObject(texture, x, y, z); this.currentFrame = 0; this.frameCount = frameCount; this.frameRate = 1 / framesPerSec; this.frameTime = this.frameRate; this.frameWidth = this.texture.width / this.frameCount; this.frameHeight = this.texture.height; return this; } this.Draw = function(deltaTime, context, deltaX, deltaY) { var sourceRect = this.frameWidth * this.currentFrame; context.drawImage(this.texture, sourceRect, 0,this.frameWidth, this.frameHeight, this.x - deltaX, this.y - deltaY, this.frameWidth, this.frameHeight); this.frameTime -= deltaTime; if (this.frameTime <= 0) { this.frameTime = this.frameRate; ++this.currentFrame; this.currentFrame %= this.frameCount; } } } AnimationManager.prototype = new DrawableObject;
The next step is to modify the call to initialize the drawing of the player sprite. Inside of the
Player
object constructor replace theInitDrawableObject
call with the following:this.InitAnimationManager(player_idle_left, 300, 300, 1, 6, 20);
Similarly replace the prototype property at the bottom of the player object with the following:
Player.prototype = new AnimationManager;
The final step is to replace the player sprite with the sprite sheet animation, which can be found within the
Chapter 1 Task 5 images
folder provided with the book. If you run the application you should see an animated player sprite in the top-left corner of the application.
The animation manager is an extension of the drawable object we created previously and as a result introduces an additional draw
function that is able to output animated sprites. The animation manager begins by declaring a number of variables that relate to the frame rate of the given sprite, the time taken to animate the sprite as well as the width and height of a given frame within a sprite sheet.
Each of these variables is initialized within the InitAnimationManager
constructor. The animation manager constructor also takes in a texture, that is, a sprite sheet, a 2D position, and a depth variable used to determine the drawing order of objects.
The draw
method within the animation manager is responsible for creating a source rectangle, which is positioned above the first cell in the sprite sheet. For each frame the source rectangle is positioned above the next cell within the sprite sheet. Once the source rectangle reaches the end of the sprite sheet its position is reset to the first cell. By doing this we are selectively picking out and displaying one cell at a time from the sprite sheet. We then loop through each cell at a frame rate, which gives the illusion of animation, in this case 12 frames per second.
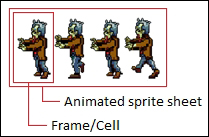
In order to display an animated sprite on the canvas, we replaced the call to initialize drawing of the player sprite within the player constructor. Previously, we passed the player texture and position to the DrawableObject
constructor. However, now that we are animating the player we also need to pass a frame count and frame rate, that is, the number of cells within the sprite sheet and the rate at which the animation is updated per second. Finally, we use the prototype keyword to extend the functionality of the DrawableObject
constructor to the AnimationManager
function.