Sometimes modifying your code while debugging is just too slow. This recipe will introduce you to the web console and give you some pointers on using it.
We will need a sandbox to play in. For the purpose of this recipe, we'll use one of the default examples built into Meteor.
In a terminal window, enter the following command:
$ meteor create --example leaderboard
Once created, navigate to the leaderboard
folder and start Meteor:
$ cd leaderboard $ meteor
We can now navigate to our leaderboard example page (http://localhost:3000
) using the web browser of our choice.
We need to enable the web console in various browsers. In no particular order, here's how to get to the web console on the browsers you're likely to be working with:
Enable the Develop menu by going to Safari | Preferences | Advanced (CMD+, shortcut if you prefer) and making sure the Show Development Menu in menu bar option is enabled:

Now, on any web page, click on Show Error Console under Develop (or use the CMD + Alt + C shortcut):
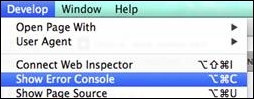
This will bring up the web console, as shown in the following screenshot:
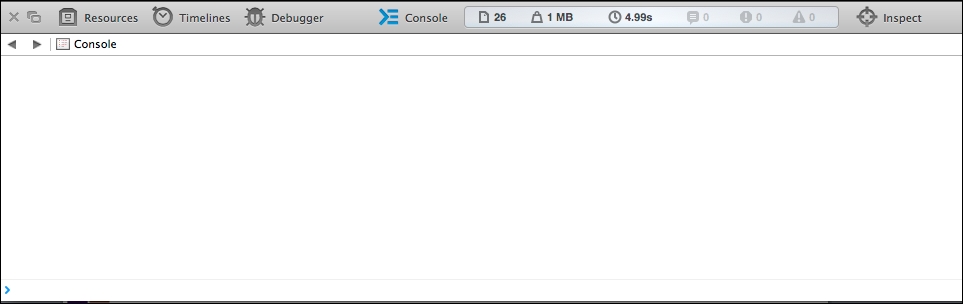
To show what's possible with a web console, let's create a new scientist, and add some points to Tesla. Because, Imma let you finish, but Nikola Tesla was the greatest scientist of all time. Of. All. Time.
If you haven't done so already, navigate to
http://localhost:3000/
.Now, open the web console if it's not already open.
In the console, run the following command:
> Players.insert({name:"Stephen Hawking"})
The name Stephen Hawking will now be in the list. You can select his name and add some points:
Now, let's give Tesla the love he deserves. First, let's find the
_id
value for the Tesla record. Run the following command in the console:> Players.findOne({name:"Nikola Tesla"})
Harvest the
_id
valuefrom the result and modify the score, similar to the following:> Players.update({_id:"bPDpqp7hgEx4d6Eui"}, {$set:{score:334823}})
Tesla will now be placed at the top of the leaderboard:
One last manipulation, let's deselect any of the scientists mentioned in the preceding screenshot. Enter the following command in the console:
> Session.set("selectedPlayer",null)
You will notice that, now no scientist is selected.
The web console acts as an extension of your code. You are able to receive log messages, call exposed methods and events, or change and instantiate global variables. You are able to do this dynamically in real time, which can have a lot of advantages as you're testing or debugging.
With a few well-composed lines of code, we were able to replicate the behavior found in the event handlers and methods that belong to the app. In other words, we were able to manually test things, rather than having to modify, save, and retest the code. This kind of impromptu testing can really save us time.
The web console and the associated development tools can perform all kinds of client-side debugging and testing tasks. You can run prewritten scripts, pause code during execution, monitor variables and the call stack, and even create your own methods and variables dynamically.
Tip
The web console varies a little bit from browser to browser, but you can find instructions that apply to nearly every web console at https://developers.google.com/chrome-developer-tools/docs/console.