To create our application, we must be able to serve at least two static files, the index.html
, which is the HTML page, and the bundle.js
file, which contains all the logic for the application written in JavaScript.
Serving the static
file using express
is extremely easy. To do so, let's change the index.js
file inside the src
folder (src
/index.js
) where we implemented our express server, and paste the following code:
app.use(express.static('static'));
This code tells express to serve all files in the static
folder as static files; now we will be able to see our page for the first time. To do this, run your application with:
node src/index.js
Do not forget to start the Redis server as we are using it to communicate the messages. If you do not start your Redis server you will see an error like this:
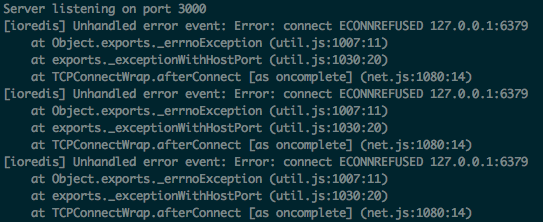
So, do not forget to run the Redis server.
After starting the Redis server, and running our node application, we can already see the web chat
application on...