Building our first Dapr sample
It is time to see Dapr in action: we are going to build a web API that returns a hello world message. I chose to base all my samples in the C:\Repos\dapr-samples\
folder, and I created a C:\Repos\dapr-samples\chapter01
folder for this first sample. We'll take the following steps:
- Let's start by creating a Web API .NET project, as follows:
PS C:\Repos\dapr-samples\chapter01> dotnet new webapi -o dapr.microservice.webapi
- Then, we add the reference to the Dapr SDK for ASP.NET. The current version is
1.1.0
. You can look for the package versions on NuGet at https://www.nuget.org/packages/Dapr.Actors.AspNetCore with thedotnet add package
command, as illustrated in the following code snippet:PS C:\Repos\dapr-samples\chapter01> dotnet add package Dapr.AspNetCore --version 1.1.0
- We need to apply some changes to the template we used to create the project. These are going to be much easier to do via VS Code—with the
<directory>\code .
command, we open it in the scope of the project folder. - To support Dapr in ASP.NET, I made a few changes to the code. In
Startup.cs
, I changed theConfigureServices
method toservices.AddControllers().AddDapr();
.In
Configure
, I also addedendpoints.MapSubscribeHandler();
. This is not necessary for our sample, as we will not use the pub/sub features of Dapr. Nevertheless, it is better to have it in mind as the base set of changes you need to apply to a default ASP.NET project.Finally, in order to simplify the code, I removed
app.UseHttpsRedirection();
.Here is the modified code of the
Startup.cs
class:using Microsoft.AspNetCore.Builder; using Microsoft.AspNetCore.Hosting; using Microsoft.Extensions.Configuration; using Microsoft.Extensions.DependencyInjection; using Microsoft.Extensions.Hosting; namespace dapr.microservice.webapi { public class Startup { public Startup(IConfiguration configuration) { Configuration = configuration; } public IConfiguration Configuration { get; } // This method gets called by the runtime. Use //this method to add services to the container. public void ConfigureServices (IServiceCollection services) { services.AddControllers().AddDapr(); } // This method gets called by the runtime. Use //this method to configure the HTTP request pipeline. public void Configure(IApplicationBuilder app, IWebHostEnvironment env) { if (env.IsDevelopment()) { app.UseDeveloperExceptionPage(); } app.UseRouting(); app.UseAuthorization(); app.UseEndpoints(endpoints => { endpoints.MapSubscribeHandler(); endpoints.MapControllers(); }); } } }
In the preceding code block, I instructed Dapr to leverage the model-view-controller (MVC) pattern in ASP.NET. Keep in mind there is an alternate approach for Dapr in ASP.NET, which does rely on routing with
MapGet(...)
andMapPost(...)
. You can see an example at https://github.com/dapr/dotnet-sdk/tree/master/examples/AspNetCore/RoutingSample. - Finally, I added a controller named
HelloWorldController
, as illustrated in the following code snippet:using Dapr; using Microsoft.AspNetCore.Mvc; using System; namespace dapr.microservice.webapi.Controllers { [ApiController] public class HelloWorldController : ControllerBase { [HttpGet("hello")] public ActionResult<string> Get() { Console.WriteLine("Hello, World."); return "Hello, World"; } } }
In the preceding code snippet, you can see
[HttpGet("hello")]
: this ASP.NET attribute is evaluated by Dapr to identify the method name. - In order to run a Dapr application, you use the following format:
dapr run --app-id <your app id> --app-port <port of the application> --dapr-http-port <port in Dapr> dotnet run
I left the ASP.NET default port as
5000
but I changed the Dapr HTTP port to5010
. The following command line launches the Dapr application:PS C:\Repos\dapr-samples\chapter01\dapr.microservice.webapi> dapr run --app-id hello-world --app-port 5000 --dapr-http-port 5010 dotnet run Starting Dapr with id hello-world. HTTP Port: 5010. gRPC Port: 52443
The initial message informs you that Dapr is going to use port
5010
for HTTP as specified, while for gRPC it is going to auto-select an available port.The log from Dapr is full of information. To confirm your application is running correctly in the context of the Dapr runtime, you can look for this part:
Updating metadata for app command: dotnet run You're up and running! Both Dapr and your app logs will appear here.
At this stage, ASP.NET is responding locally on port
5000
and Dapr is responding on port5010
. In order to test Dapr, let's invoke acurl
command, as follows. Using the browser is equally fine:PS C:\Repos\dapr-samples\chapter01> curl http://localhost:5010/v1.0/invoke/hello-world/method/hello Hello, World
This exciting response has been returned by Dapr, which passed our (the client's) initial request to the ASP.NET Web API framework. You should also see it logged as
Console.WriteLine
sends its output to the Dapr window, as follows:== APP == Hello, World.
- From another window, let's verify our Dapr service details: instead of using the
dapr list
command, let's open the Dapr dashboard, like this:PS C:\Windows\System32> dapr dashboard Dapr Dashboard running on http://localhost:8080
We can open the dashboard by navigating to http://localhost:8080, to reveal the following screen:
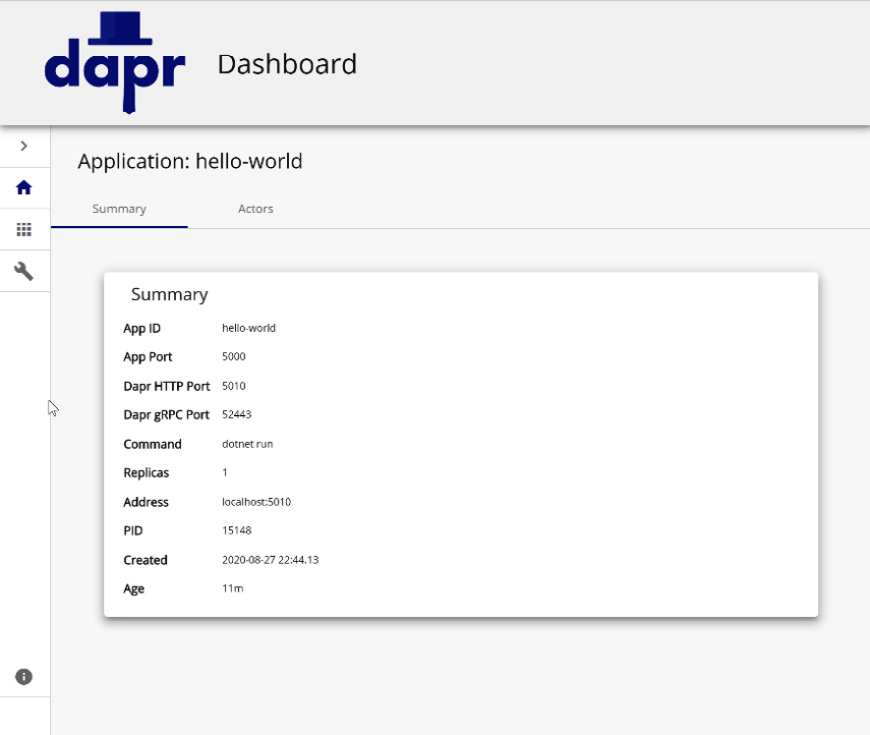
Figure 1.3 – Dapr dashboard application
The Dapr dashboard shown in Figure 1.3 illustrates the details of our hello-world
application.
In this case, the Dapr dashboard shows only this sample application we are running on the development machine. In a Kubernetes environment, it would show all the microservices running, along with the other components.
The Dapr dashboard also displays the configured components in the hosting environment, as we can see here:
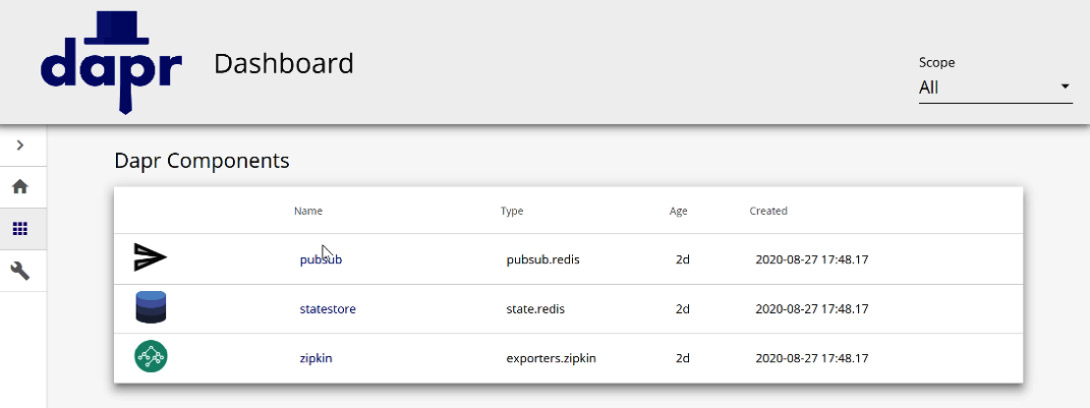
Figure 1.4 – Dapr dashboard components
In Figure 1.4, the Dapr dashboard shows us that the local installation of Redis is configured as state store and pub/sub components, in addition to the deployment of Zipkin.
This ends our introductory section, where we were able to build our first Dapr sample.