Efficient software development means following good and consistent development practices. This is not just about creating fantastic, well-designed code, but also about the seemingly little things such as naming conventions and comments.
We will not cover all the best practice guidelines here, as it is more pertinent to explain them as we use them. We'll cover some general guidelines before we start.
First, all the objects you create or elements added to an object authored by another party must be prefixed. The prefix is usually specific to your organization; in the case of Binary, we use two prefixes—BCL for all work we carry out as a VAR, and AXP for our ISV work. The prefix cannot be of any three characters; for example, Sys
would be a bad prefix because it is also used by Microsoft for their system objects.
When adding element to your own objects, do not prefix them. All type names (extended data types, tables, classes, and so on) must begin with a capital letter, and all instance variables and methods must have a lowercase first letter.
Although the AX compiler is not case-sensitive, the CIL is; thankfully, this compiles from the p-code, so it isn't normally a problem. It is nonetheless good practice to write code as if it is case-sensitive. This also helps make the code much easier to read.
The second part of naming is that you should capitalize the first letter of each word in the type, method, or variable name; for example, CustTable
and the initFromCustTable
method. This, again, makes the code much easier to read.
A version control system cannot replace good code commenting. Any change made to an object that was not authored by you should be tagged with the following:
Your organization's prefix
Your initials
Reference to the business requirement (for example, a Technical Definition Document (TDD) reference or a Functional Requirements Document (FRD) reference)
The date
A few words as to the reason for the change and what the change is intended to do
A second line (as required) to explain what the change is intended to do
Let's take a look at the following example:
// BCL 5.2.4 (var) by SB on 2014/06/07: Set the focus to the item id control SalesLine_ItemId.setFocus(); // BCL 5.2.4 end
Tip
Use yyyy/mm/dd as a date format because dd/mm/yyyy or mm/dd/yyyy can cause confusion across continents. However, if you do see a date that is not YMD, it is most likely to be DMY, not MDY.
If you add a method to an existing object, you should add a header comment. This can easily be done by entering ///
in the first line of the method. It should contain the comment line, as shown in the preceding code snippet.
If you have made a change to a form layout, you should add a method called DevelopmentHistory
highlighting the changes. Otherwise, you may realize that it gets lost when another developer applies an update or hotfix. This content format is up to your organization; this would normally be a list of changes formatted in a table in the same format as the earlier change tag.
Adding comments is often a pain and, when focused on writing an exciting piece of code, they are easily forgotten. One way to solve this is by making the addition easy by modifying the EditorScripts
class.
The EditorScripts
class has methods that create code blocks and even entire methods for you, either through the right-click menu or by typing a keyword. The class can be modified by adding a public method that accepts an Editor
instance. Appendix C, Code Editor Scripts, covers this topic.
Editor scripts are code snippets that insert code into the current method, and can even ask for information as part of the process. They insert either statement blocks, such as a switch
statement block, or an entire method. They are simple to enter; simply type the script name and press the Tab key.
For example, if we have a class called BclFleetVehicleTableType
and enter the text construct in a method, the following code is inserted:
public static BclFleetVehicleTableType construct() { return new BclFleetVehicleTableType(); }
The available scripts can be seen by right-clicking on the method and navigating to Scripts | template, as shown in the following screenshot:
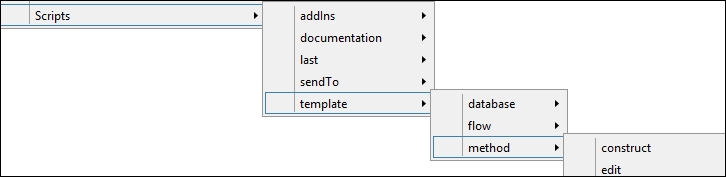