Now it's time to move on and take a look at the Microsoft .NET Standard. In this recipe, we will be looking at version 2.0 of the .NET Standard library. At the start, we will be building a small .NET Standard class library and using it with different .NET-based applications.
Let's make sure we have downloaded and installed one of the flavors of Visual Studio 2017. If you are running on Windows, you have the option of choosing Visual Studio 2017 Community Edition, Professional Edition, or Enterprise Edition. If you are running on a mac, you have the choice of Visual Studio 2017 for macOS. Also, Visual Studio Code is available for all Windows, Mac, and Linux platforms. Visit http://www.visualstudio.com and follow the instructions to download the Visual Studio of your choosing.
In the next step, we will be required to download and install .NET Core 2.0. Again, simply visit http://www.dot.net/core and download the latest version, in this case, version 2.0 of .NET Core. The site has a very simple and informative set of instructions on how to install .NET Core 2.0 on your system.
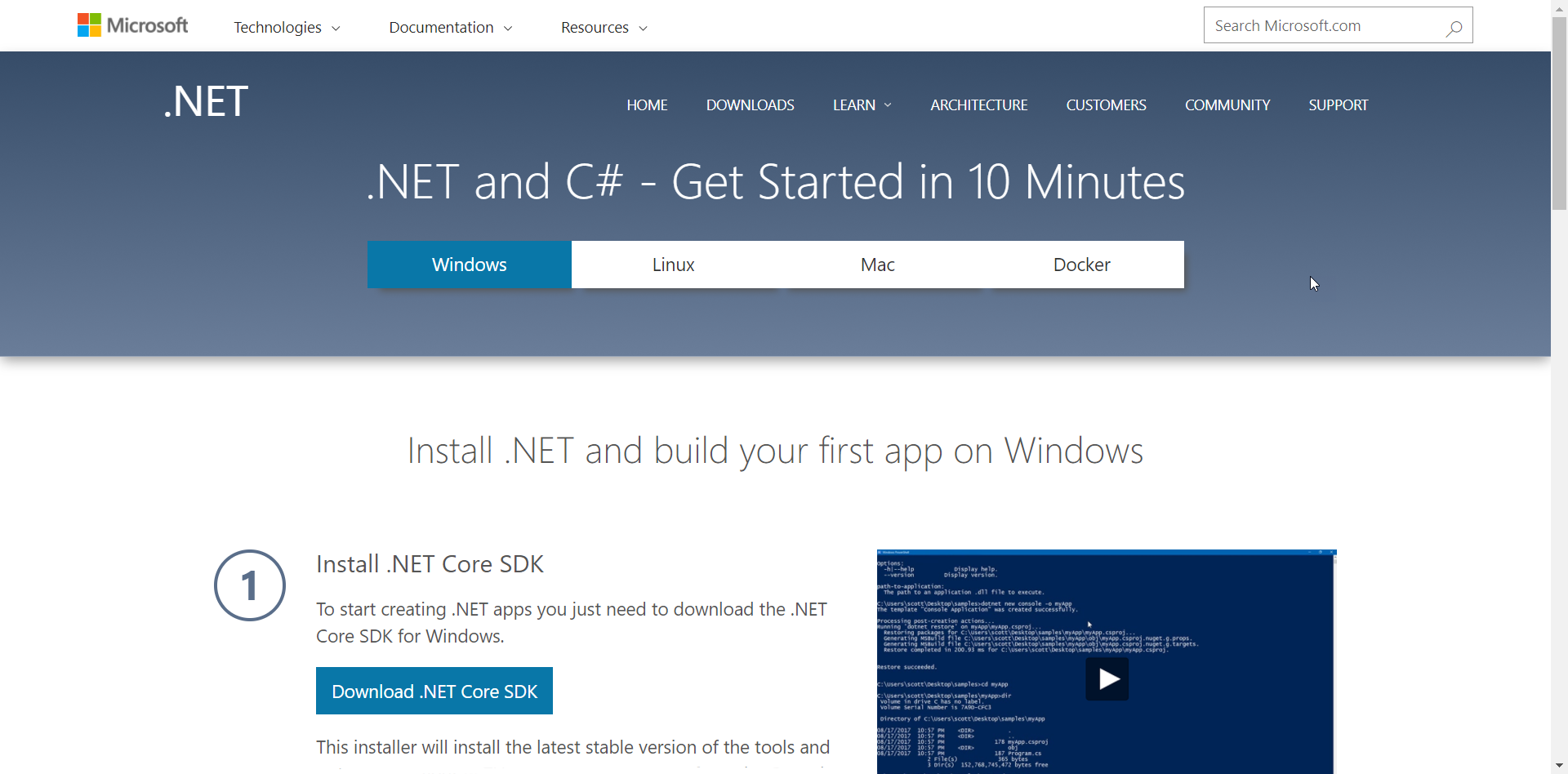
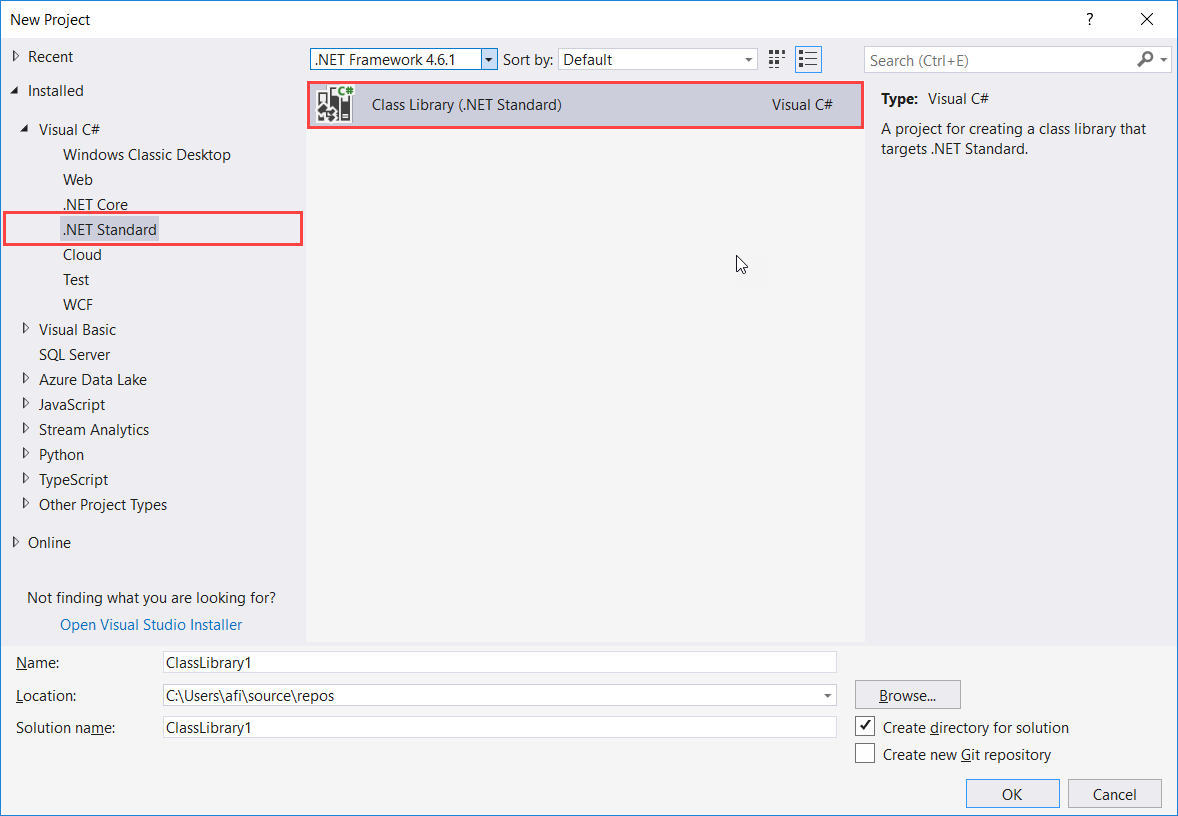
- In the
Name:
text box, type a name for your class library. Let's typeChapter1.StandardLib.HelloUniverse
and select a preferred location under theLocation:
drop-down list, or click theBrowse...
button and select a location. Leave the defaults as they are. Finally, in theSolution name:
text box, typeChapter1.StandardLib
.

- Click
OK
.
- In the
Solution Explorer
(press Ctrl + Alt + L) , click onClass1.cs
, press F2, and rename itHelloUniverse.cs
. Confirm the renaming by selecting Yes in the confirmation box. - Change the namespace from
Chapter1.StandardLib.HelloUniverse
toChapter1.StandardLib
. - Now, in between the curly brackets of the
HelloUniverse
class, type the following code:
public string SayHello(string name) { return $"Hello {name}, welcome to a whole new Universe of .NET Standard 2.0"; }
- Press Ctrl + S to save the changes and press Ctrl + Shift + B to build the code. If the build completes without any errors, we are good to go with the next recipe on how to use this class library.
.NET Standard 2.0 is the latest release of its kind. .NET Standard is all about sharing code. Unlike .NET Framework class libraries, .NET Standard class library code can be shared across almost all of the .NET ecosystem. The latest version of .NET Standard is 2.0. At the time of writing, it can be shared across NET Framework 4.6.1, .NET Core 2.0, Mono 5.4, Xamarin.iOS 10.14, Xamarin.Mac 3.8, Xamarin.Android 7.5, and the upcoming version of Universal Windows Platform (UWP). It also replaces Portable Class Libraries (PCLs) as the tool for building .NET libraries that work everywhere.
In steps 1 to 5, we have created a new .NET Standard 2.0-based class library project. In step 4, we have given a proper name to the class library as well as to the solution. It is good practice to give a meaningful name to the project and to the solution. In step 6, we have changed the name of the default class to HelloUniverse.cs
, and it automatically changed the class name thanks to refactoring features in Visual Studio. If you look at the layout of the .NET Standard 2.0 library template, you will see a Dependencies
node. In a normal .NET Framework class library, we had References
. The Dependencies
node will list all the dependent components for that class library.
In step 8, we added a simple public method that takes a string parameter and returns a message with the parameter sent to the method. Finally, we checked for syntax errors and typos by building the solution.