Now that we have our top level or the root window ready, it is time to think over the question: which components should appear in the window? In Tkinter jargon, these components are called widgets.
The syntax that is used to add a widget is as follows:
my_widget = tk.Widget-name (its container window, ** its configuration options)
In the following example ( 1.02.py ), we will add two widgets, a label and a button, to the root container. Also, note how all the widgets are added between the skeleton code that we defined in the first example:
import tkinter as tk
root = tk.Tk()
label = tk.Label(root, text="I am a label widget")
button = tk.Button(root, text="I am a button")
label.pack()
button.pack()
root.mainloop()
Running the preceding code(1.02.py) will generate a window with a label and a button widget, as shown in the following screenshot:
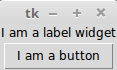
The following is a description of the preceding code:
- This code added a new instance named label for the label widget. The first parameter defined root as its parent or container. The second parameter configured its text option to read I am a label widget.
- Similarly, we defined an instance of a Button widget. This is also bound to the root window as its parent.
- We used the pack() method, which is essentially required to position the label and button widgets within the window. We will discuss the pack() method and several other related concepts when exploring the geometry management task. However, you must note that some sort of geometry specification is essential for the widgets to be displayed.