This section discusses the main motivations behind TypeScript and how to install the TypeScript SDK. Installation concerns just developers' machines since TypeScript code is completely compiled into JavaScript code and doesn't need any runtime library to be executed.
Basics and installation
Adding types to JavaScript
TypeScript is a language that is transpiled to JavaScript; that is, TypeScript code is processed to generate JavaScript code.
As a first approximation, you may imagine that TypeScript code is obtained by adding type declarations of variables to usual JavaScript code. For instance, let's consider this JavaScript code:
var firstName = "Francesco";
var surName = "Abbruzzese";
function fullName(x, y, spaces){
x + Array(spaces+1).join(' ') + y;
}
>fullName(firstName, surName, 3)
>"Francesco Abbruzzese"
TypeScript adds a type declaration to the firstName and surName variable declarations, and it also adds types to the fullName function arguments, and to the function's return value:
var firstName: string = "Francesco";
var surName: string = "Abbruzzese";
function fullName(x: string, y: string, spaces: number): string{
x + Array(spaces+1).join(' ') + y;
}
TypeScript and JavaScript code are very similar, the only difference being the colon followed by the type immediately after each variable or argument declaration and each function declaration.
In this very simple example, the JavaScript code generated by TypeScript transpilation is identical to the code written directly in JavaScript, so what is the advantage of using TypeScript?
Simple: type checking! The TypeScript compiler verifies type compatibility, thus immediately discovering errors that might otherwise manifest themselves with strange behaviors at runtime.
Suppose, for instance, that we call fullName with its arguments in the wrong order:
fullName(3, firstName, surName)
The TypeScript transpiler immediately discovers the error since 3 is not a string and surName is not a number, while JavaScript tries to automatically convert types and gets the wrong result:
>fullName(3, firstName, surName)
>"3Francesco"
Using JavaScript of the future now!
While TypeScript was initially conceived to perform better compile-time checks than JavaScript, very soon its mission was extended to mitigate the different support for new JavaScript standards which are at the moment, ECMAScript 6-8. TypeScript includes most of ECMAScript 6-8's important features, but you may target the transpiled code at previous JavaScript versions. When a feature is not available in the target JavaScript version, the TypeScript transpiler creates code that simulates this feature in the target JavaScript version.
For instance, TypeScript includes ECMAScript 6 classes that will be covered in Chapter 4, Using Classes and Interfaces, and ECMAScript 8 async/await, which will be covered in the Promises and async/await notation section of Chapter 9, Decorators and Advanced ES6 Features. Here is an example of async/await use:
async function asyncAwaitExample(url: string): string {
let response= await fetch(url);
return await response.text();
}
The syntax is completely analogous to C# async/await.
Installing the Visual Studio 2017 TypeScript SDK
As a default, Visual Studio 2017 installers automatically install TypeScript SDK, and as long as you keep Visual Studio updated, you should always have a recent version of the TypeScript SDK. Anyway, if for some reason TypeScript was not installed, or if you want to be sure you have the latest version of the TypeScript SDK, proceed as follows.
Go to Program and Functionalities in your computer's Control Panel and verify that TypeScript SDK is installed, and which version is installed:
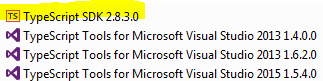
In this case, TypeScript is already installed and its version is 2.8.3.0.
Open Visual Studio 2017 and go to Tools | Extensions and Updates:
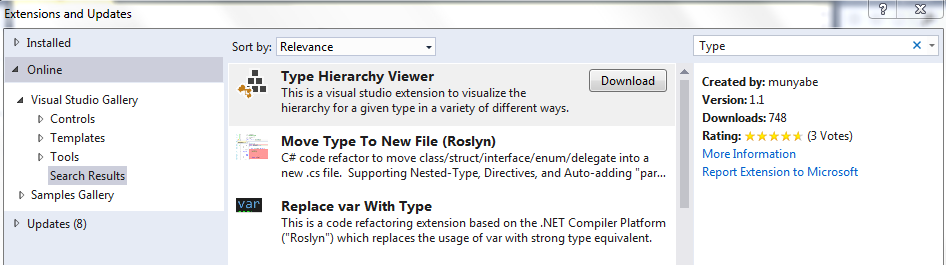
When the Extensions and Updates window opens, in the left-hand menu, select Online | Visual Studio Gallery | Tools, and then type TypeScript in the text box in the upper-right corner.
A few seconds after you finish typing TypeScript, you should see all available versions of the TypeScript SDK. Select the most recent version:
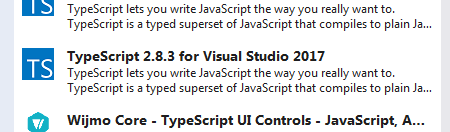
In this case, the most recent version is 2.8.3.
If the version you found is more recent than the one already installed, click on the list item to select it, and then click on the Download button that appears to download the TypeScript SDK installer. When the download completes, double-click on the installer to install the SDK.
Installation of Node.js-based TypeScript compiler
In the Using VS Code section of Chapter 8, Building Typescript Libraries, we will learn TypeScript development without Visual Studio. In this case, we need the Node.js-based TypeScript compiler that is independent of Visual Studio.
As a first step, go to the Node.js website at https://nodejs.org/en/ and download the recommended version of Node.js.
Once installation is complete, open a Windows Command Prompt and type node -v to verify Node.js has been installed properly, and to verify its version.
We also need npm, the Node.js packages handler (a kind of NuGet for Node.js packages). npm is automatically installed with Node.js. Type npm -v in the Windows Command Prompt to verify its proper installation and its version.
Here is the result of typing these commands in a Windows Command Prompt:
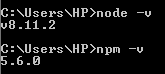
Now, installing TypeScript compiler is quite easy; just type npm install -g typescript. This will install the last version of the TypeScript compiler globally on your computer.
If you need a different version of the TypeScript compiler for a specific project, you may install it locally for that project folder, while all other projects will continue using the version installed globally. This can be done as follows:
- Open Command Prompt in the project folder.
- Suppose the version you would like to install is 2.7.1, then type the following command:
npm install --save-dev [email protected]