As a default, Visual Studio compiles all TypeScript files in your project into JavaScript files with the same names, but with the .js extension, and they are placed in the same folder. Moreover, for each .js file, it creates a file with the same name but with a .js.map extension that is called a map file. Map files map locations in the generated JavaScript files to locations in their TypeSctipt sources, thus enabling debugging on the TypeScript sources. This default behavior of the TypeScript compiler may be changed in two ways: either by forcing Visual Studio to invoke the TypeScript compiler with different parameters, or by specifying the desired options in a configuration file.
TypeScript compiler options
Specifying parameters for the TypeScript compiler
All parameters Visual Studio uses to invoke the TypeScript compiler may be edited in the project properties by right-clicking on the project icon and selecting Properties:
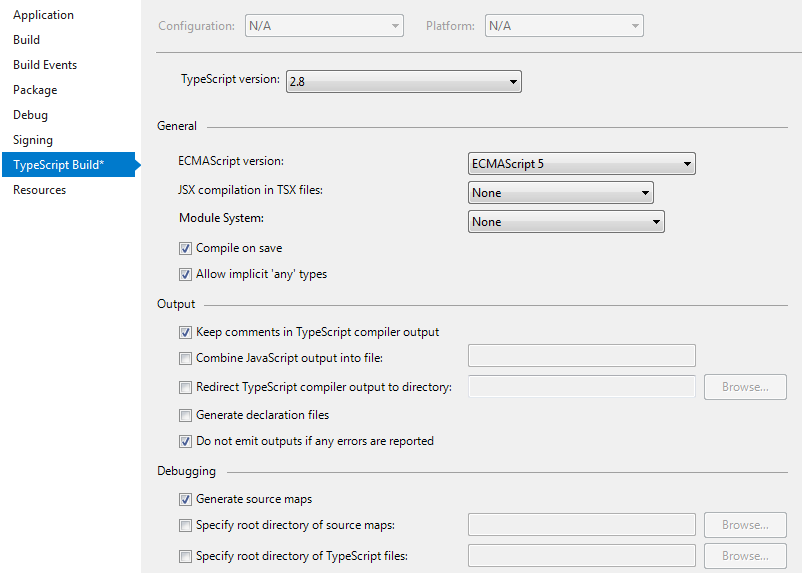
In the window that appears, select TypeScript Build. Here, you may change several options:
- The TypeScript version from the ones installed.
- The ECMAScript version of the JavaScript code generated. At the moment, ECMAScript 5 is supported by all mainstream browsers, while higher versions have incomplete support, so it is the advised choice for most applications.
- Whether to compile on save or not. If this option is deselected, TypeScript files are compiled only when the whole project is built. This possibility may be useful in complex projects in which the TypeScript build is quite slow because after transpilation, large files are first minimized and then bundled into chunks.
- To keep, or not, all TypeScript source comments in the generated JavaScript files.
- To choose a different directory for both the JavaScript and map files created by the compiler.
- To emit JavaScript code, or not, in the case of errors.
- To emit map files or not. If you want to debug TypeScript sources, you should always emit map files.
- The directory in which to look for TypeScript files to compile. If this directory is not provided, the project root is taken.
Let's unselect the Compile on save option, save the changes, modify something in the test.ts file (for instance, change the Hello string to Hello world), and verify that the file is not compiled on save. Have a look at the last modification date of the test.js file. After this test, do not forget to select the Compile on save option again.
Now, let's try to put all the files generated by the TypeScript compiler in the js folder:

After that, if you change something in the test.ts file and save it, test.js and test.js.map will appear in the .js folder:
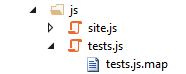
TypeScript configuration file
A TypeScript configuration file allows us to specify several more options. We may specify several input directories instead of just one, we may specify paths/files to be excluded from compilation, or we may specify several more compilations flags.
If you want Visual Studio to invoke the TypeScript compiler automatically, you must give the project TypeScript configuration file its default name, which, tsconfig.json. Otherwise, you must invoke the TypeScript compiler manually and pass it the configuration file name as a command-line parameter.
In order to add a TypeScript configuration file to your project, right-click on the project icon in the project explorer and select Add New Item:
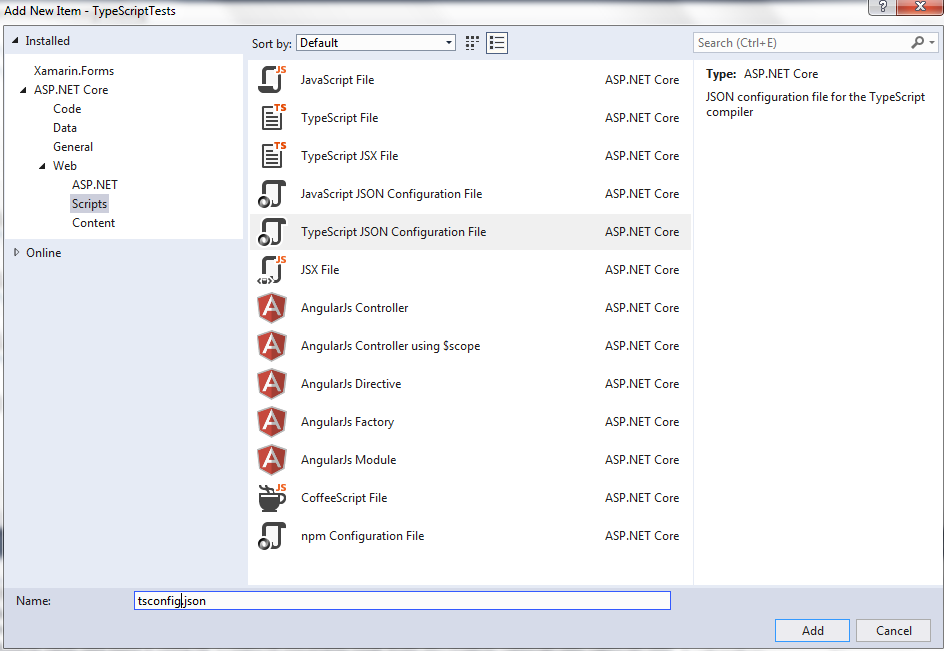
Then select TypeScript JSON Configuration File. The configuration file should appear in the project root. Replace its content with the following code:
{
"compileOnSave": true,
"compilerOptions": {
"noImplicitAny": false,
"noEmitOnError": true,
"removeComments": false,
"sourceMap": true,
"target": "es5",
"outDir": "wwwroot/js"
},
"include": [
"wwwroot/ts/**/*.ts"
],
"exclude": [
"**/node_modules"
]
}
compileOnSave enables compilation on save, while compilerOptions contains all compiler flags. It is easy to recognize the same options we discussed previously:
- noEmitOnError: No code generation in the case of errors.
- removeComments: It removes/keeps comments in JavaScript-emitted code.
- sourceMap: It enables/disables map files.
- target: It specifies the JavaScript version of the emitted code; in our case, ECMAScript 5.
- outDir: It specifies where to place the emitted files. If omitted, this is next to their sources.
- noImplicitAny: It will be discussed later on in this chapter.
Intellisense suggests the allowed options and values, so there is no need to remember their exact names.
include specifies a list of directory or file patterns to include in the compilation. Each entry is a pattern that may match several directories/files, since entries may contain wildcards: * matches a single name, while ** matches any path. In our case, there is a single pattern that selects all files contained in wwwroot/ts and in all its subdirectories (because of the **), whose extension is .ts.
exclude specifies a list of patterns to remove from the ones added within other options (for instance, with the include option). In our case, a unique pattern excludes all files contained in any directory called node_modules located at any depth, since these directories usually include JavaScript packages downloaded with the NPM Package Manager.
There is also a files option to add a list of files (just files, not patterns) to the compilation:
"files":[
"file1.ts",
"dir/file2.ts",
...
]
If no files or include options are specified, the whole project root is taken.
Save tsconfig.json and modify tests.ts. When the file is saved, it should be compiled according to the options contained in tsconfig.json.
Once a tsconfig.json has been added, all options in the TypeScript Build panel of the project properties are disabled:
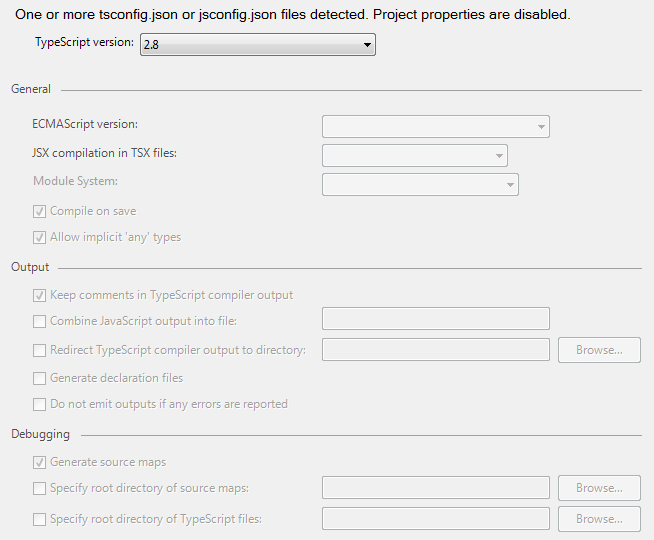
You may use this panel just to select the TypeScript version; that is, the compiler that is automatically invoked by Visual Studio. All other options must be specified in the configuration file you added.