Once you have the TypeScript SDK installed, adding a TypeScript code file to an ASP.NET Core project is straightforward. Your TypeScript file will be compiled in a JavaScript file that you may call, as usual, in any View. However, you may debug your code and put breakpoints directly in the TypeScript source.
Adding TypeScript to your web projects
Your first TypeScript file
Let's open Visual Studio and create an ASP.NET Core project 2.x named TypeScriptTests:
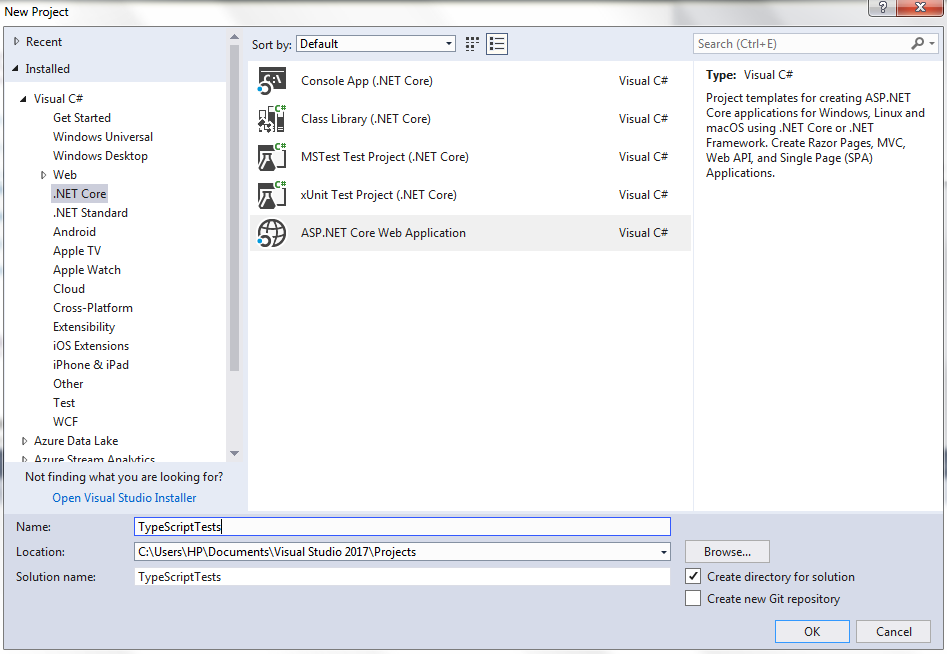
Click OK, and in the new window that appears, select an MVC 2.x application with No Authentication (we need just a View for where to call our code):
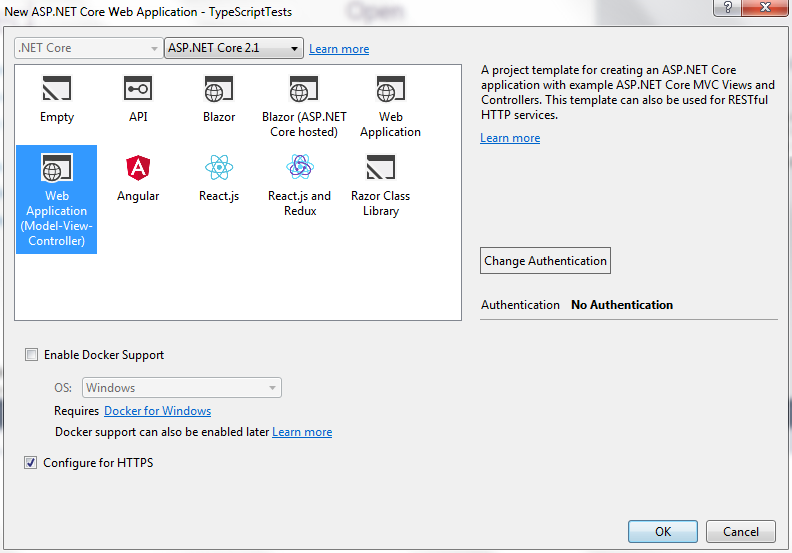
Once the project finishes loading, run it to verify that the project has been scaffolded properly. Then, in the solution explorer, right-click on the wwwroot node and select Add | New Folder to add a new folder named ts:
Finally, right-click on the ts folder and select Add New Item. The following window opens:
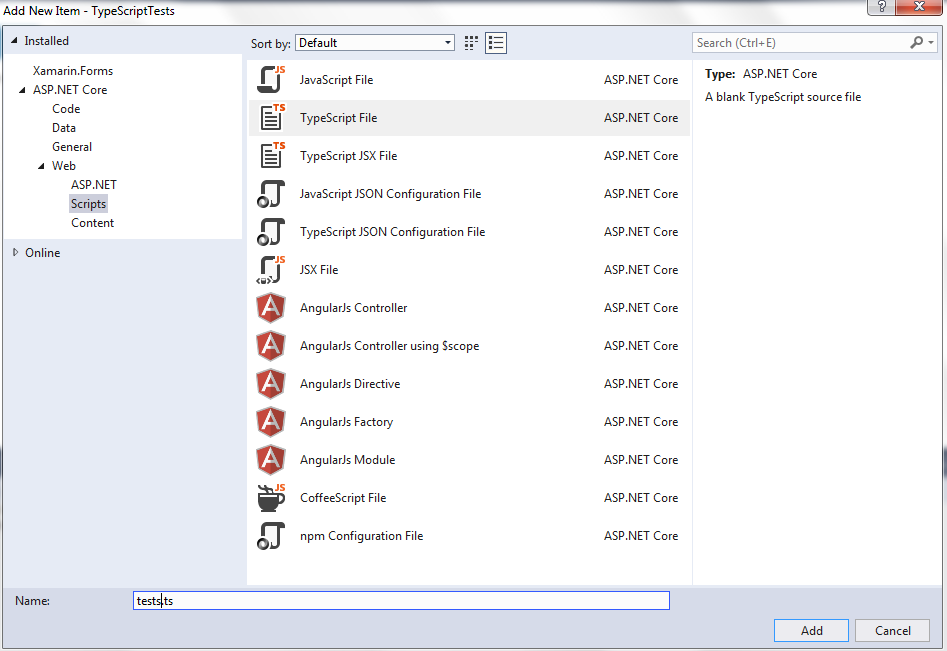
In the left-hand menu, select ASP.NET Core | Web | Scripts. Then select the TypeScript File and name it tests.ts.
Let's add some test code to the newly added test.ts file:
var firstName: string = "Francesco";
var surName: string = "Abbruzzese";
function fullName(x: string, y: string, spaces: number): string {
return x + Array(spaces+1).join(' ') + y;
}
alert(fullName(firstName, surName, 3)+" Hello");
Thanks to declarations and strong typing, the Visual Studio editor helps us with IntelliSense:
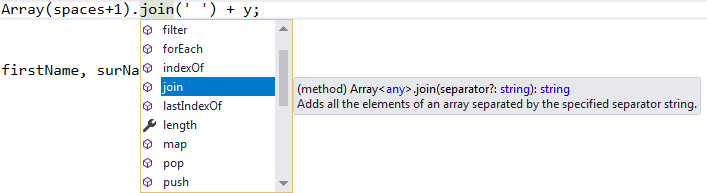
As soon as we save the file, Visual Studio invokes the TypeScript compiler to transpile our file into a test.js JavaScript file:
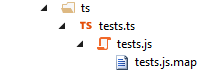
In the case of errors, the JavaScript file is not generated and all errors are displayed in the editor as soon as we save the file. Let's try this behavior by misspelling the join method:

When we build the project, all TypeScript errors are also added to the Error List panel, as follows:
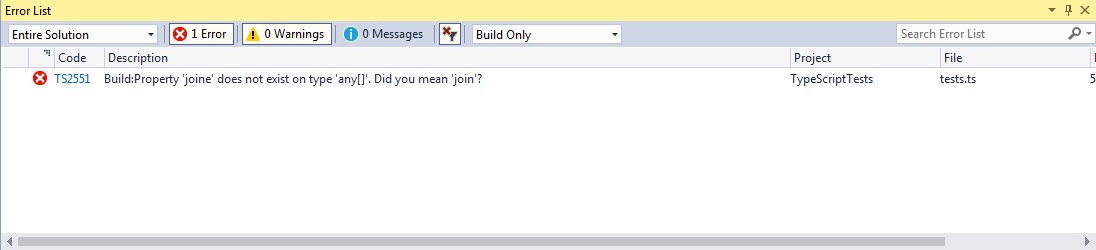
Running and debugging TypeScript code
Running and debugging TypeScript code is straightforward. It is enough to add the transpiled JavaScript file to a view. Let's add our previous test.js file to the bottom of the Home/Index.cshtml view:
@section Scripts{
<script src="~/ts/tests.js"></script>
}
It is worth remembering that Script is a section defined in the Layout view of the ASP.NET Core MVC template. It allows all views to place the JavaScript code they need in the right place in the Layout view. When you run the project, an alert box should appear as soon as the website's default page is loaded in the browser, as shown in the following screenshot:
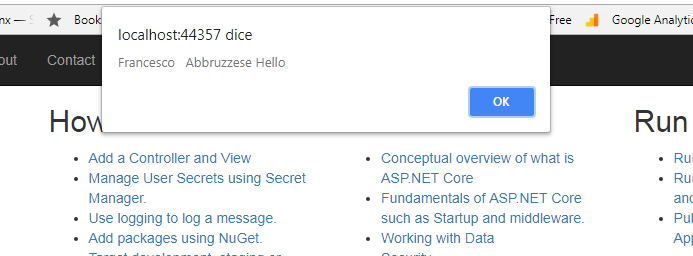
Thanks to the test.js.map map file generated by the TypeScript compiler, it is possible to debug the TypeScript source instead of the JavaScript transpiled code. In fact, map files contain all the information needed to map each position in the transpiled file into a position in the source file. Map files are not a peculiarity of TypeScript but a well-recognized standard, since they are also used for mapping minimized JavaScript files to their sources.
Let's place a breakpoint on the last instruction of test.ts and run the project:

Once the breakpoint is hit, you may benefit from all the Visual Studio debugging features you are used to when debugging C# code.
You may see values by hovering over variables with the mouse:

Or, you can use a Watch 1 window:

You also have access to the calls stack, to an immediate window, to the intellitrace, and to all other C# code features you are used to.