In this recipe we will learn how to use the features of the Gyroscope events of the iOS device.
We have looked at how to use the iOS devices accelerometer and modify the views background color based on the orientation of the axes. In this recipe we will look at how to incorporate the gyroscope features.
To begin, follow these simple steps as outlined:
Open the
AccelGyroExample.xcodeproj
project file.Open the
ViewController.m
implementation file and modify theviewDidLoad
method as shown in the following highlighted code snippet:- (void)viewDidLoad { [super viewDidLoad]; // Set up the accelerometer UIAccelerometer *accelerometer = [UIAccelerometer sharedAccelerometer]; accelerometer.updateInterval = 0.5; accelerometer.delegate = self; // Perform a check to see if the device // supports the Gyroscope feature if ([self isGyroscopeAvailable] == YES) { motionManager = [[CMMotionManager alloc] init]; [motionManager startGyroUpdatesToQueue:[NSOperationQueue currentQueue] withHandler:^(CMGyroData *gyroData, NSError *error) { [self doGyroRotation:gyroData.rotationRate]; }]; } else { // Device does not support the gyroscope feature NSLog(@"No Gyroscope detected."); } }
Next, create the following code sections, as specified in the following code snippet:
// Handles rotation of the Gyroscope - (void)doGyroRotation:(CMRotationRate)rotation { double value = (fabs(rotation.x)+fabs(rotation.y) + fabs(rotation.z)) / 8.0; if (value > 1.0) { value = 1.0;} self.view.alpha = value; } // Checks to see if Gyroscope is available on the device - (BOOL) isGyroscopeAvailable { #ifdef __IPHONE_4_0 CMMotionManager *gyroManager = [[CMMotionManager alloc] init]; gyroManager.gyroUpdateInterval = 1.0 / 60.0; BOOL gyroAvailable = gyroManager.gyroAvailable; return gyroAvailable; #else return NO; #endif }
Build and run the application by choosing Product | Run from the Product menu or alternatively by pressing Command + R.
When the compilation completes, the application will be displayed onto your iOS device. Try moving your device in all directions to see the background color start cycling through the various colors.
What we have done in this recipe is added code to determine by using the #Ifdef __IPHONE_4_0
directive if the device currently in use is an iPhone 4. If this is the case, it then checks to see if the device supports the gyroscope feature and a Boolean status YES
is returned; otherwise NO
is returned.
Next, we set up our UIAccelerometer
delegate and update intervals to be twice per second in order to request updates. We then make a call to our isGyroscopeAvailable
function to check to see if the gyroscope feature is supported.
Finally, we call the
startGyroUpdatesToQueue
function and add a handler to call our doGryroRotation
function that then updates the alpha blend color of our view. If no gyroscope feature is supported, this
is logged out to the debug window.
Note
To start receiving and handling rotation-rate data for the gyroscope feature, you need to create an instance of the CMMotionManager
class and call one of the following methods to it.
The following image shows how the iPhone responds to changes on its three axes when the iPhone is tilted. Under normal gravity, each of these values will be between -1 and +1 with a value of 0 being the middle center point. Moving the phone in a rapid motion will increase these values:
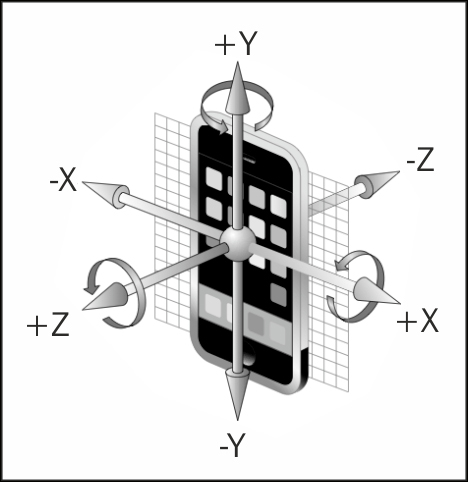
The following table explains each of the
method calls relating to the CMMotionManager
class:
Note
If you would like to find
out more information about the CoreMotion
class, you can refer to the Apple Developer documentation located on the link http://developer.apple.com/library/ios/#documentation/CoreMotion/Reference/CoreMotion_Reference/_index.html.
The Using the shake gesture with the touch interface recipe
The Sensing movement with the accelerometer input recipe
The Using Xcode to create an iOS project recipe in Chapter 1, Getting and Installing the iOS SDK Development Tools