As mentioned earlier, Julia is open source and is available for free. It can be downloaded from the website at http://julialang.org/downloads/.
The website has links to documentation, tutorials, learning, videos, and examples. The documentation can be downloaded in popular formats, as shown in the following screenshot:
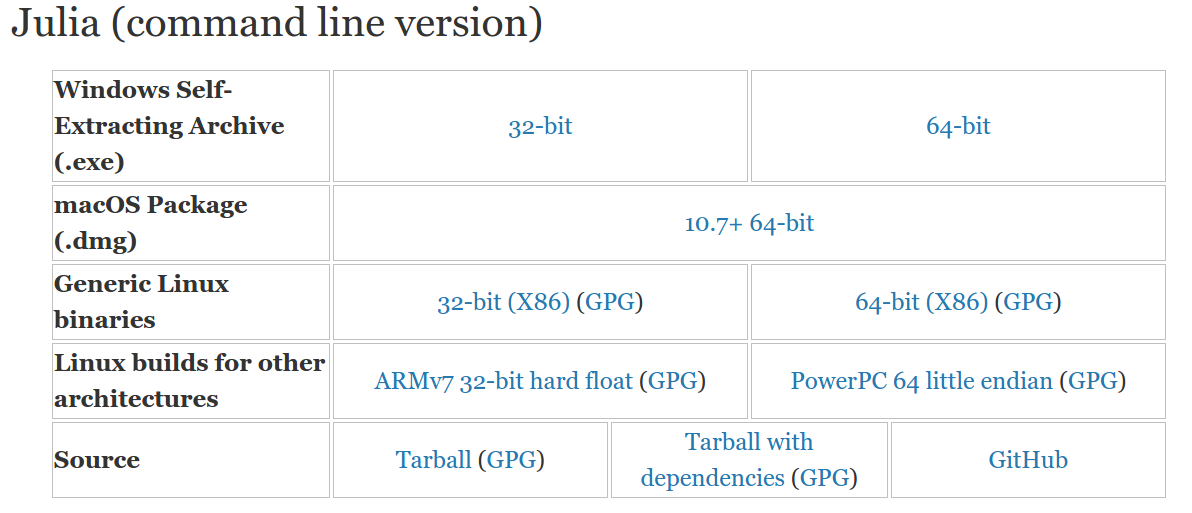
It is highly recommended to use the generic binaries for Linux provided on the julialang.org website.
As Ubuntu and Fedora are widely used Linux distributions, a few developers were kind enough to make the installation on these distributions easier by providing it through package manager. We will go through them in the following sections.
Ubuntu and its derived distributions are one of the most famous Linux distributions. Julia's deb packages (self-extracting binaries) are available on the website of JuliaLang, mentioned earlier. These are available for both 32-bit and 64-bit distributions. One can also add Personal Package Archive (PPA), which is treated as an apt repository to build and publish Ubuntu source packages. In the Terminal, type the following commands:
$ sudo apt-get add-repository ppa:staticfloat/juliareleases $ sudo apt-get update
This adds the PPA and updates the package index in the repository. Now install Julia using the following command:
$ sudo apt-get install Julia
The installation is complete. To check whether the installation is successful in the Terminal, type the following:
$ julia --version
This gives the installed Julia's version:
$ julia version 0.5.0
To uninstall Julia, simply use apt
to remove it:
$ sudo apt-get remove julia
For Fedora/RHEL/CentOS or distributions based on them, enable the EPEL repository for your distribution version. Then, click on the link provided. Enable Julia's repository using the following:
$ dnf copr enable nalimilan/julia
Or copy the relevant .repo
file available at:
/etc/yum.repos.d/
Finally, in the Terminal type the following:
$ yum install julia
Go to the Julia download page (https://julialang.org/downloads/) and get the .exe
file provided according to your system's architecture (32-bit/64-bit). The architecture can be found on the property settings of the computer. If it is amd64 or x86_64, then go for 64-bit binary (.exe
), otherwise go for 32-bit binary. Julia is installed on Windows by running the downloaded .exe
file, which will extract Julia into a folder. Inside this folder is a batch file called julia.exe
, which can be used to start the Julia console.
Users with macOS need to click on the downloaded .dmg
file to run the disk image. After that, drag the app icon into the Applications
folder. It may prompt you to ask if you want to continue, as the source has been downloaded from the internet and so is not considered secure. Click on Continue
if it was downloaded from the official Julia language website. Julia can also be installed using Homebrew
on a Mac, as follows:
$ brew update $ brew tap staticfloat/julia $ brew install julia
The installation is complete. To check whether the installation is successful in the Terminal, type the following:
$ julia --version
This gives you the Julia version installed.
Building from source could be challenging for beginners. We assume that you are on Linux (Ubuntu) right now and are building from source. This provides the latest build of Julia, which may not be completely stable. Perform the following steps to build Julia from source:
- On the downloads page of the Julia website, download the source. You can choose Tarball or GitHub. It is recommended to use GitHub. To clone the repo, GitHub must be installed on the machine. Otherwise, choose
Download as ZIP
. Here is the link: https://github.com/JuliaLang/julia.git. - To build Julia, it requires various compilers: g++, gfortran, and m4. We need to install them first, if not installed already, using the
$ sudo apt-get install gfortran g++ m4
command. - Traverse inside the Julia directory and start the make process, using the following command:
$ cd julia $ make
- On a successful build, Julia can be started up with the
./julia
command. - If you used GitHub to download the source, you can stay up to date by compiling the newest version using the following commands:
$ git pull $ make clean $ make
Building from source on Windows and macOS is also straightforward. It can be found at https://github.com/juliaLang/julia/.
Let's have a look at the directories and their content:
Directory | Contents |
| Julia's standard library |
| Miscellaneous set of scripts, configuration files |
| External dependencies |
| Source for user manual |
| Source for standard library function help text |
| Example Julia programs |
| Source for Julia's language core |
| Test suits |
| Benchmark suits |
| Source for various frontends |
A brief explanation about the directories mentioned earlier:
- The
base/
directory consists of most of the standard library. - The
src/
directory contains the core of the language. - There is also an
examples
directory containing some good code examples, which can be helpful when learning Julia. It is highly recommended to use these in parallel.
On the successful build on Linux, these directories can be found in the Julia's folder. These are usually present in the build directory.