Even though books, videos, and the Internet can be helpful in your efforts to learn PowerShell, you will find that your greatest ally in this quest is PowerShell itself. In this chapter, we will look at two fundamental weapons in any PowerShell scripter's arsenal, the Get-Command
and Get-Help
cmdlets. The topics covered in this chapter include the following:
- Finding commands using
Get-Command
- Finding commands using tab completion
- Using
Get-Help
to understand cmdlets - Interpreting the command syntax
You saw in the previous chapter that you are able to run standard command-line programs in PowerShell and that there are aliases defined for some cmdlets that allow you to use the names of commands that you are used to from other shells. Other than these, what can you use? How do you know which commands, cmdlets, and aliases are available?
The answer is the first of the big three cmdlets, the Get-Command
cmdlet. Simply executing Get-Command
with no parameters displays a list of all the entities that PowerShell considers to be executable. This includes programs in the path (the environment variable), cmdlets, functions, scripts, and aliases.
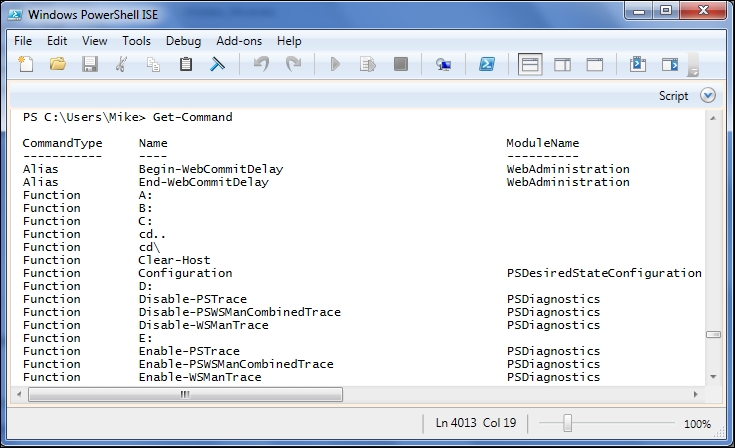
This list of commands is long and gets longer with each new operating system and PowerShell release. To count the output, we can use this command:
Get-Command | Measure-Object
The output of this command is as follows:
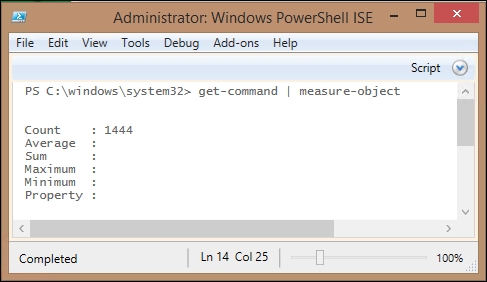
The Measure-Object
cmdlet can be used to count the number of items in the output from a command. In this case, it tells us that there are 1444 different commands available to us. In the output, we can see that there is a CommandType
column. There is a corresponding parameter to the Get-Command
cmdlet that allows us to filter the output and only shows us certain kinds of commands. Limiting the output to the cmdlets shows that there are 706 different cmdlets available:
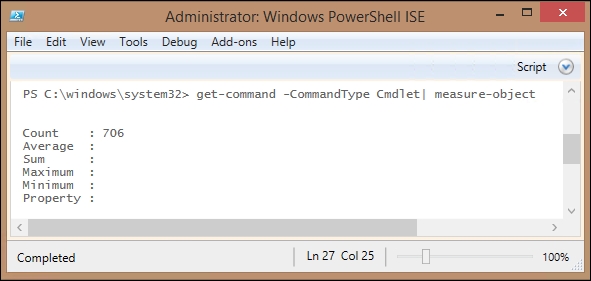
Getting a big list such as this isn't very useful though. In reality, you are usually going to use Get-Command
to find commands that pertain to a component you want to work with. For instance, if you need to work with Windows services, you could issue a command such as Get-Command *Service*
using wildcards to include any command that includes the word service
in its name. The output would look something similar to the following:
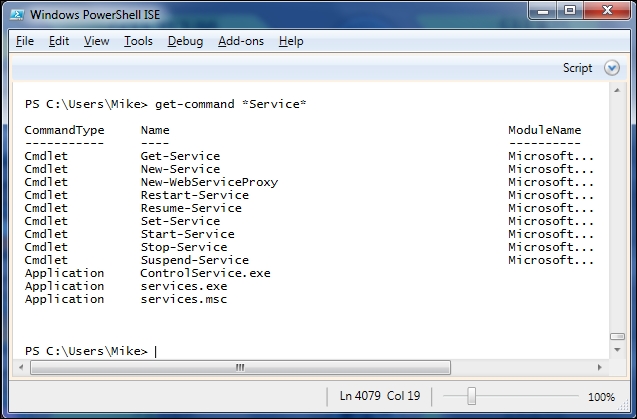
You can see in the output that applications found in the current path and the PowerShell cmdlets are both included. Unfortunately, there's also a cmdlet called New-WebServiceProxy
, which clearly doesn't have anything to do with Windows services. To better control the matching of cmdlets with your subject, you can use the –Noun
parameter to filter the commands. Note that by naming a noun, you are implicitly limiting the results to PowerShell objects because nouns are a PowerShell concept. Using this approach gives us a smaller list:
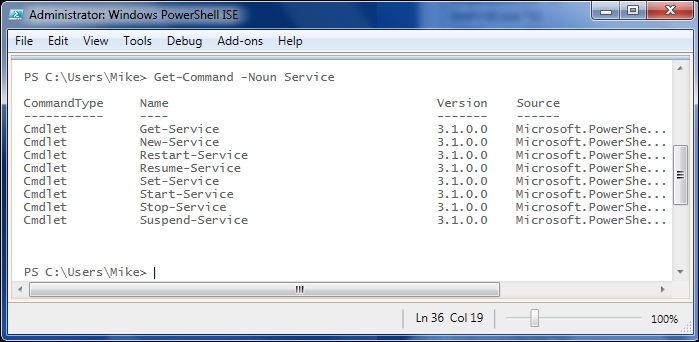
Although Get-Command
is a great way to find cmdlets, the truth is that the PowerShell cmdlet names are very predictable. In fact, they're so predictable that after you've been using PowerShell for a while you won't probably turn to Get-Command
very often. After you've found the noun or the set of nouns that you're working with, the powerful tab completion found in both the PowerShell console and the ISE will allow you to enter just a part of the command and press tab to cycle through the list of commands that match what you have entered. For instance, in keeping with our examples dealing with services, you could enter *-Service
at the command line and press tab. You would first see
Get-Service, followed by the rest of the items in the previous screenshot as you hit tab. Tab completion is a huge benefit for your scripting productivity for several reasons, such as:
- You get the suggestions where you need them
- The suggestions are all valid command names
- The suggestions are consistently capitalized
In addition to being able to complete the command names, tab completion also gives suggestions for parameter names, property, and method names, and in PowerShell 3.0 and above, it gives the parameter values. The combination of tab completion improvements and IntelliSense in the ISE is so impressive that I recommend that people upgrade their development workstation to at least PowerShell 3.0, even if they are developing PowerShell code that will run in a 2.0 environment.
Knowing what commands you can execute is a big step, but it doesn't help much if you don't know how you can use them. Again, PowerShell is here to help you. To see a quick hint of how to use a cmdlet, write the cmdlet name followed by -?
. The beginning of this output for Get-Service
is shown as follows:
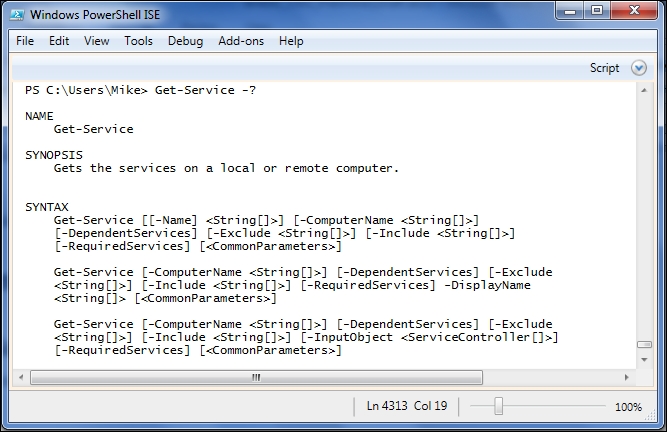
Even this brief help, which was truncated to fit on the page, shows a brief synopsis of the cmdlet and the syntax to call it, including the possible parameters and their types. The rest of the display shows a longer description of the cmdlet, a list of the related topics, and some instructions about getting more help about Get-Service
:
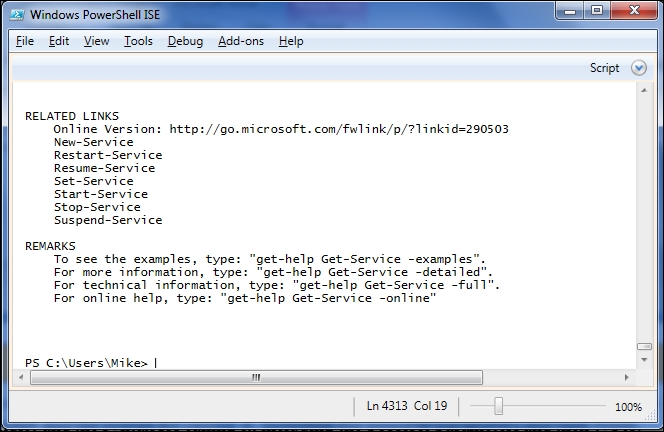
In the Remarks section, we can see that there's a cmdlet called Get-Help
(the second of the "big 3" cmdlets) that allows us to view more extensive help in PowerShell. The first type of extra help we can see is the examples. The example output begins with the name and synopsis of the cmdlet and is followed, in the case of Get-Service
, by 11 examples that range from simple to complex. The help for each cmdlet is different, but in general you will find these examples to be a treasure trove providing an insight into not only how the cmdlets behave in isolation, but also in combination with other commands in real scenarios:
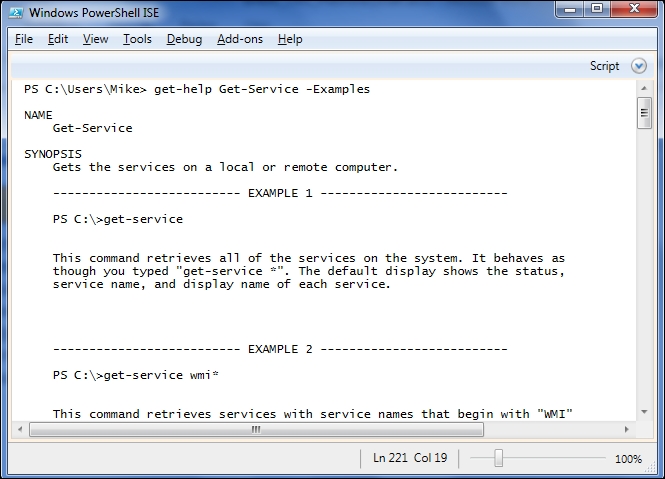
Also, mentioned in this are the ways to display more information about the cmdlet using the –Detailed
or –Full
switches with Get-Help
. The –Detailed
switch shows the examples as well as the basic descriptions of each parameter. The –Full
switch adds sections on inputs, outputs, and detailed parameter information to the –Detailed
output.
If you're running PowerShell 3.0 or above, instead of getting a complete help entry, you probably received a message like this:

This is because in PowerShell 3.0, the PowerShell team switched to the concept of update-able help. In order to deal with time constraints around shipping and the complexity of releasing updates to installed software, the help content for the PowerShell modules is now updated via a cmdlet called Update-Help
. Using this mechanism, the team can revise, expand, and correct the help content on a continual basis, and users can be sure to have the most recent content at all times:
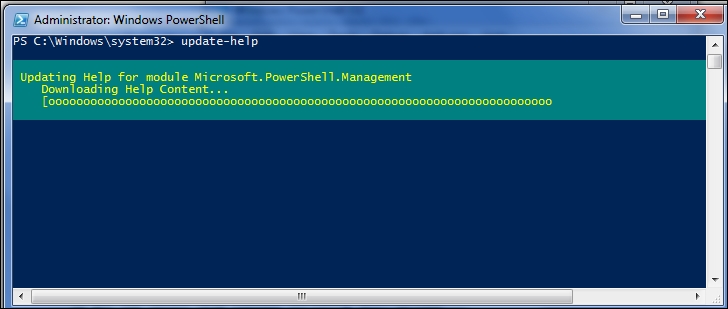
In addition to the help with individual cmdlets, PowerShell includes help content about all aspects of the PowerShell environment. These help topics are named beginning with about_
and can also be viewed with the Get-Help
cmdlet. The list of topics can be retrieved using get-Help
about_*
:
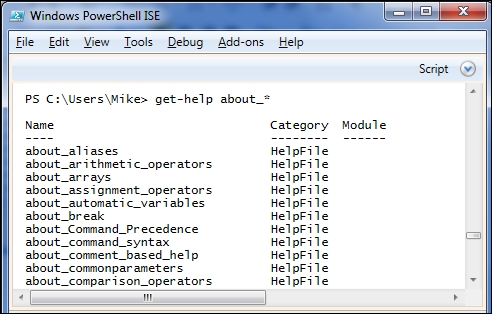
Using measure-object
, as we saw in a previous section, we can see that there are 124 topics listed in my installation:
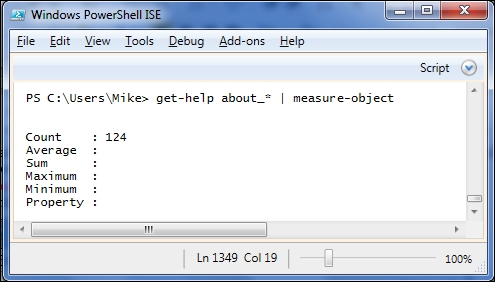
These help topics are often extremely long and contain some of the best documentation on the working of PowerShell you will ever find. For that reason, I will end each chapter in Module 1 with a list of help topics to read about the topics covered in the chapter.
In addition to reading the help content in the output area of the ISE and the text console, PowerShell 3.0 added a –ShowWindow
switch to get-help
that allows viewing in a separate window. All help content is shown in the window, but sections can be hidden using the settings button:
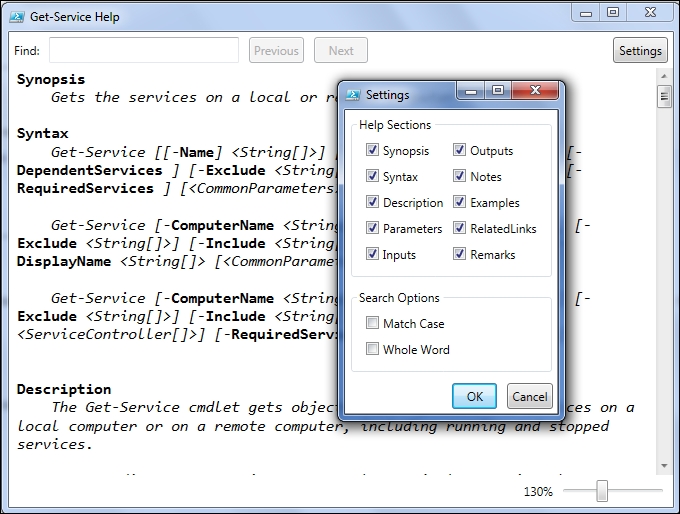
The syntax section of the cmdlet help can be overwhelming at first, so let's drill into it a bit and try to understand it in detail. I will use the get-service
cmdlet for an example, but the principles are same for any cmdlet:
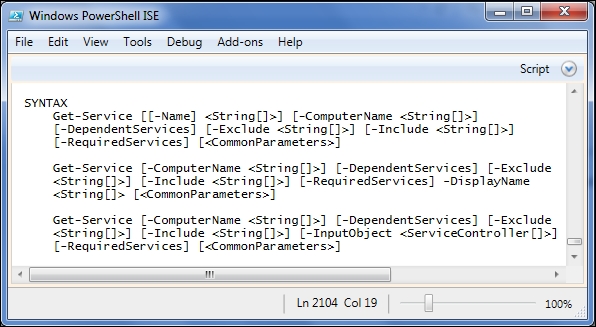
The first thing to notice is that there are three different get-service
calls illustrated here. They correspond to the PowerShell concept of ParameterSets, but you can think of them as different use cases for the cmdlet. Each ParameterSet, or use case will have at least one unique parameter. In this case, the first includes the –Name
parameter, the second includes –DisplayName
, and the third has the –InputObject
parameter.
Each ParameterSet lists the parameters that can be used in a particular scenario. The way the parameter is shown in the listing, tells you how the parameter can be used. For instance, [[-Name] <String[]>]
means that the name parameter has the following attributes:
- It is optional (because the whole definition is in brackets)
- The parameter name can be omitted (because the name is in brackets)
- The parameter values can be an array (list) of strings (String[])
In general, a parameter will be shown in one of the following forms:
Form |
Meaning |
---|---|
|
Optional parameter of type |
|
Optional parameter of type |
|
Required parameter of type |
|
A switch (flag) |
Applying this to the Get-Service
syntax shown previously, we see that some valid calls of the cmdlet could be:
This chapter dealt with the first two of the "big three" cmdlets for learning PowerShell, get-Command
and get-Help
. These two cmdlets allow you to find out which commands are available and how to use them. In the next chapter, we will finish the "big 3" with the get-member
cmdlet that will help us to figure out what to do with the output we receive.