In this section, you will see how to implement your first Highcharts graph. Assuming that we want to run Highcharts from our local web server, first download the latest version from the Highcharts website: http://www.highcharts.com/download.
Alternatively, we can load the library via the Content Delivery Network (CDN), as follows:
<script type="text/javascript" src="http://code.highcharts.com/highcharts.js"></script>
For debugging, highcharts.src.js
is also available from the CDN.
When you unpack the downloaded ZIP file, you should see the following directory structure under the Highcharts-4.x.x
top-level directory:
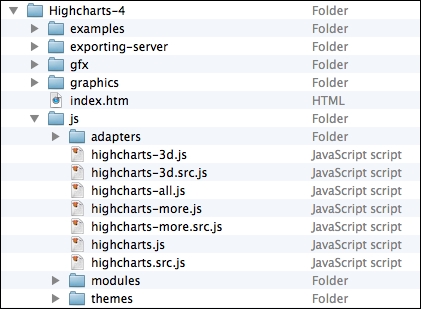
The following is what each directory contains and is used for:
index.html
: This is the demo HTML page, which is the same as the demo page on the Highcharts website, so that you can experiment with Highcharts offline.examples
: This contains all the source files for the examples.gfx
: This contains an image file required to produce the radial gradient effect for browsers that only support VML.exporting-server
: This directory contains three main components: a Java implementation of the online export server, a PhantomJs toolkit to run Highcharts on the service side, and server script to service the chart export function using Batik. This directory is useful for users who need to set up their own internal export service or run Highcharts as a server process. See Chapter 14, Server-side Highcharts and Chapter 15, Highcharts Online Services and Plugin.js
: This is the main directory for Highcharts code. Each JavaScript filename has two suffixes:.src.js
, which contains the source code with comments in it, and.js
, which is the minified version of the JavaScript source file. Thehighcharts.js
file contains the core functionality and the basic chart implementations,highcharts-3d.js
is the 3D charts extension, andhighcharts.more.js
contains additional series such as polar, gauge, bubble, range, waterfall, errobar, and boxplot.Highcharts-all.js
lets users provide all the chart series in their application.adapters
: This contains the default adapter standalone-framework in source and compressed format.modules
: Since the last edition, Highcharts has created a number of plugin modules such as solid-gauge, funnel, exporting, drilldown, canvas-tools and so on. This directory contains these plugins.themes
: This has a set of JavaScript files prebuilt with settings such as background colors, font styles, axis layouts, and so on. Users can load one of these files in their charts for different styles.
All you need to do is move the top-level Highcharts-4.x.x/js
directory inside your web server document's root directory.
To use Highcharts, you need to include Highcharts-4.x.x/js/highcharts.js
library in your HTML file. The following is an example showing the percentage of web browser usage for a public website. The example uses the minimal configuration settings for getting you started quickly. The following is the top half of the example:
<!DOCTYPE HTML> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8"> <title>Highcharts First Example</title> <script src="http://ajax.googleapis.com/ajax/libs/jquery/1.8.2 /jquery.min.js"></script> <script type="text/javascript" src="Highcharts-4.0.4/js/highcharts.js"></script>
We use the Google public library service to load the jQuery library version 1.8.2 before loading the Highcharts library. At the time of writing, the latest jQuery version is 2.1.1 and the Highcharts system requirement for jQuery is 1.8.2.
The second half of the example is the main Highcharts code, as follows:
<script type="text/javascript"> var chart; $(document).ready(function() { Chart = new Highcharts.Chart({ chart: { renderTo: 'container', type: 'spline' }, title: { text: 'Web browsers statistics' }, subtitle: { text: 'From 2008 to present' }, xAxis: { categories: [ 'Jan 2008', 'Feb', .... ], tickInterval: 3 }, yAxis: { title: { text: 'Percentage %' }, min: 0 }, plotOptions: { series: { lineWidth: 2 } }, series: [{ name: 'Internet Explorer', data: [54.7, 54.7, 53.9, 54.8, 54.4, ... ] }, { name: 'FireFox', data: [36.4, 36.5, 37.0, 39.1, 39.8, ... ] }, { // Chrome started until late 2008 name: 'Chrome', data: [ null, null, null, null, null, null, null, null, 3.1, 3.0, 3.1, 3.6, ... ] }, { name: 'Safari', data: [ 1.9, 2.0, 2.1, 2.2, 2.4, 2.6, ... ] }, { name: 'Opera', data: [ 1.4, 1.4, 1.4, 1.4, 1.5, 1.7, ... ] }] }); }); </script> </head> <body> <div> <!-- Highcharts rendering takes place inside this DIV --> <div id="container"></div> </div> </body> </html>
The spline graph is created via an object specifier that contains all the properties and series data required. Once the chart
object is created, the graph is displayed in the browser. Within this object specifier, there are major components corresponding to the structure of the chart:
var chart = new HighCharts.Chart({ chart: { ... }, title: '...' ... });
The renderTo
option instructs Highcharts to display the graph onto the HTML <div>
element with 'container'
as the ID value, which is defined in the HTML <body>
section. The type
option is set to the default presentation type as 'spline'
for any series data, as follows:
chart: { renderTo: 'container', type: 'spline' }
Next is to set title
and subtitle
, which appear in the center at the top of the chart:
title: { text: 'Web browsers ... ' }, subtitle: { text: 'From 2008 to present' },
The categories
option in the xAxis
property contains an array of x-axis labels for each data point. Since the graph has at least 50 data points, printing each x-axis label will make the text overlap. Rotating the labels still results in the axis looking very packed. The best compromise is to print every third label, (tickIntervals: 3
), which makes the labels nicely spaced out from each other.
For the sake of simplicity, we use 50 entries in xAxis.categories
to represent the time. However, we will see a more optimal and logical way to display date and time data in the next chapter:
xAxis: { categories: [ 'Jan 2008', 'Feb', .... ], tickInterval: 3 },
The options in yAxis
are to assign the title of the y-axis and set the minimum possible value to zero; otherwise, Highcharts will display a negative percentage range along the y-axis, which is not wanted for this data set:
yAxis: { title: { text: 'Percentage %' }, min: 0 },
The plotOptions
property is to control how each series is displayed, according to its type (line, pie, bar, and so on). The plotOptions.series
option is the general configuration applied to all the series types instead of defining each setting inside the series
array. In this example, the default linewidth
for every series is set to 2
-pixels wide, as follows:
plotOptions: { series: { lineWidth: 2 } },
The series
property is the heart of the whole configuration object that defines all the series data. It is an array of the series
object. The series
object can be specified in multiple ways. For this example, the name is the name of the series that appears in the chart legend and tool tip. The data is an array of y-axis values that have the same length as the xAxis.categories
array, to form (x,y) data points:
series: [{ name: 'Internet Explorer', data: [54.7, 54.7, 53.9, 54.8, 54.4, ... ] }, { name: 'FireFox', data: [36.4, 36.5, 37.0, 39.1, 39.8, ... ] }, {
Tip
Downloading the example code
You can download the example code files from your account at http://www.packtpub.com for all the Packt Publishing books you have purchased. If you purchased this book elsewhere, you can visit http://www.packtpub.com/support and register to have the files e-mailed directly to you.
The following screenshot shows how the final Highcharts should look on the Safari browser:
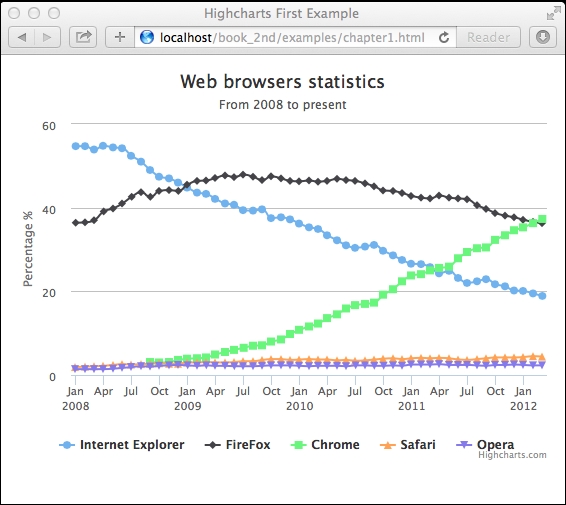
The following screenshot shows how it should look on the Internet Explorer 11 browser:
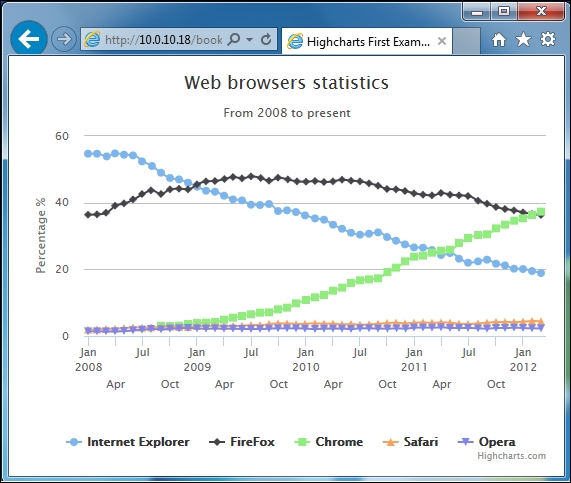
The following screenshot shows how it should look on the Chrome browser:
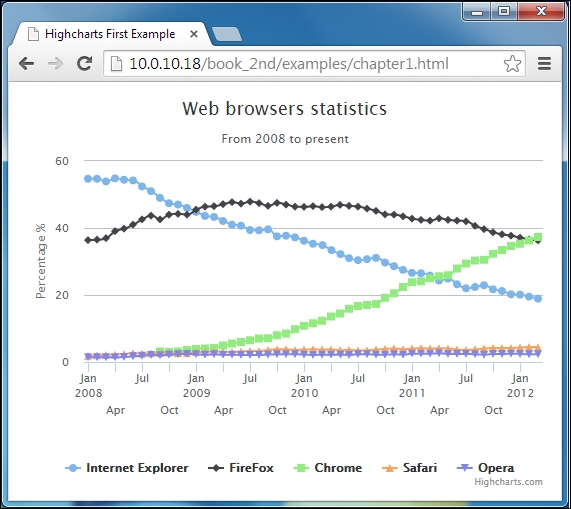
The following screenshot shows how it should look on the Firefox browser:
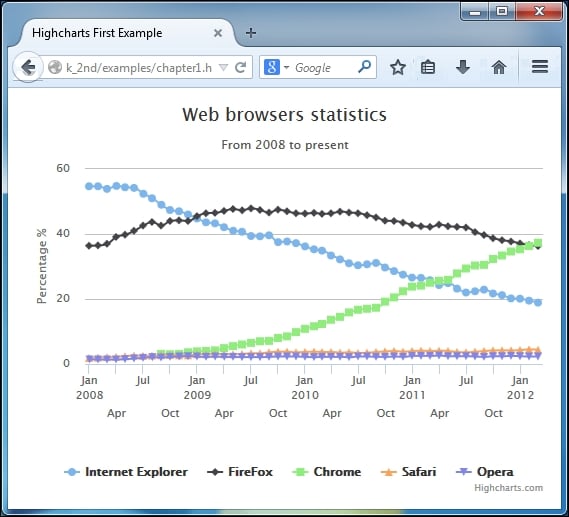