In order to extract a meaningful amount of information from the images, we need to make sure our feature extractor extracts features from all the parts of a given image. Consider the following image:
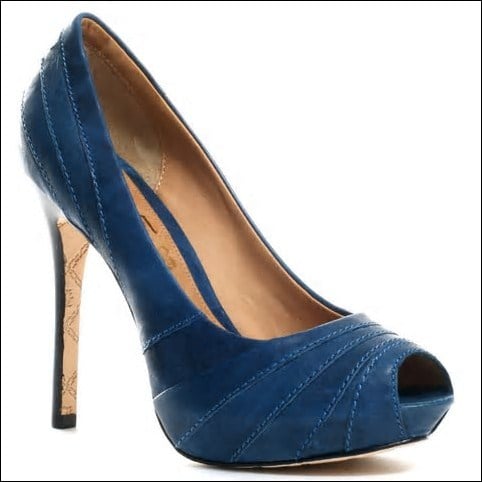
If you extract features using a feature extractor, it will look like this:
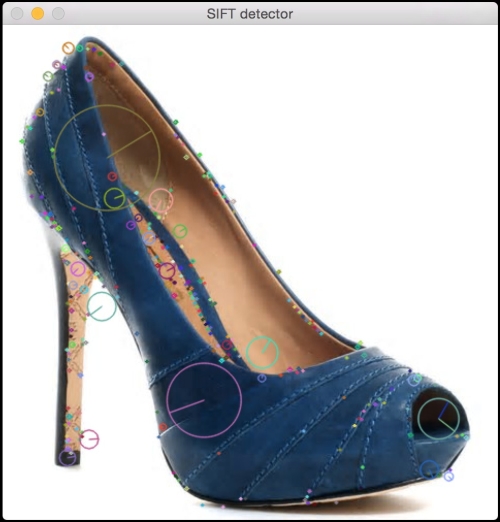
If you use Dense
detector, it will look like this:
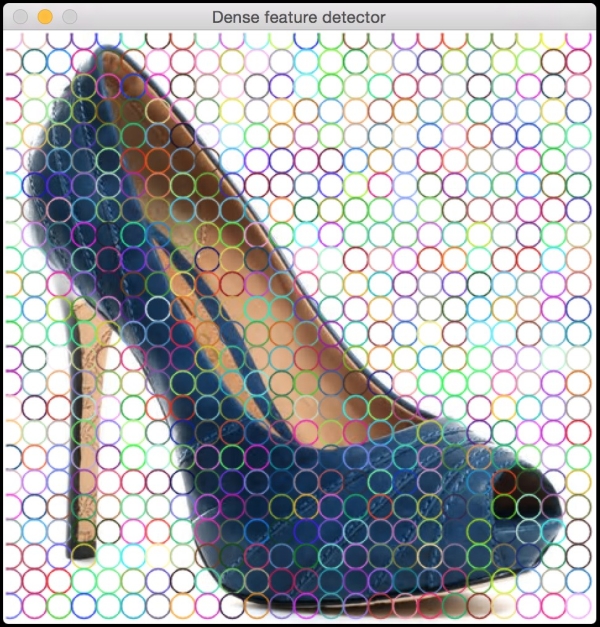
We can control the density as well. Let's make it sparse:
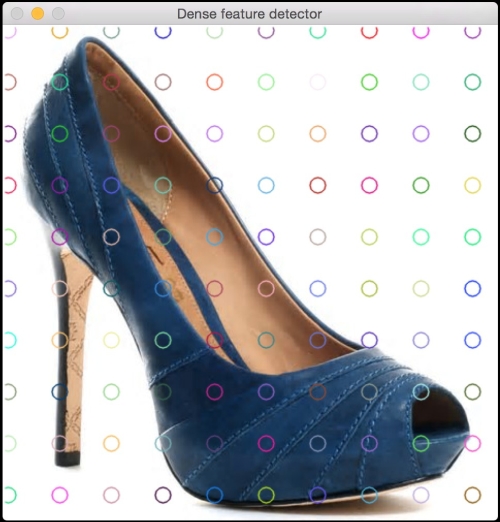
By doing this, we can make sure that every single part in the image is processed. Here is the code to do it:
import cv2 import numpy as np class DenseDetector(object): def __init__(self, step_size=20, feature_scale=40, img_bound=20): # Create a dense feature detector self.detector = cv2.FeatureDetector_create("Dense") # Initialize it with all the required parameters self.detector.setInt("initXyStep", step_size) self.detector.setInt("initFeatureScale", feature_scale) self.detector.setInt("initImgBound", img_bound) def detect...