Let's put our hands on our first code.
Tip
Downloading the example code
You can download the example code files for all Packt books you have purchased from your account at http://www.packtpub.com. If you purchased this book elsewhere, you can visit http://www.packtpub.com/support and register to have the files e-mailed directly to you.
The following is a Hello World program:
1. # File name: hello.py 2. import kivy 3. kivy.require('1.9.0') 4. 5. from kivy.app import App 6. from kivy.uix.button import Label 7. 8. class HelloApp(App): 9. def build(self): 10. return Label(text='Hello World!') 11. 12. if __name__=="__main__": 13. HelloApp().run()
Note
This is merely Python code. Launching a Kivy program is not any different from launching any other Python application.
In order to run the code, you open a terminal (line of commands or console) and specify the following command in Windows or Linux: python hello.py --size=150x100
(--size
is a parameter to specify the screen size).
On a Mac, you must type in kivy
instead of python
after installing Kivy.app
in /Applications
. Lines 2 and 3 verify that we have the appropriate version of Kivy installed on our computer.
Note
If you try to launch our application with an older Kivy version (say 1.8.0) than the specified version, then line 3 will raise an Exception
error. This Exception
is not raised if we have a more recent version.
We omit the call to kivy.require
in most of the examples in the book, but you will find it in the code that you download online (https://www.packtpub.com/), and its use is strongly encouraged in real-life projects. The program uses two classes from the Kivy library (lines 5 and 6) – App
and Label
. The class App
is the starting point of any Kivy application. Consider App
as the empty window where we will add other Kivy components.
We use the App
class through inheritance; the App
class becomes the base class of the HelloApp
subclass or child class (line 8). In practice, this means that the HelloApp
class has all the variables and methods of App
, plus whatever we define in the body (lines 9 and 10) of the HelloApp
class. Most importantly, App
is the starting point of any Kivy application. We can see that line 13 creates an instance of HelloApp
and runs it.
Now the HelloApp
class's body just overrides one of the existing App
class's methods, the build(self)
method. This method has to return the window content. In our case, a Label
that holds the text Hello World!
(line 10). A Label is a widget that allows you to display some text on the screen.
Note
A widget is a Kivy GUI component. Widgets are the minimal graphical units that we put together in order to create user interfaces.
The following screenshot shows the resulting screen after executing the hello.py
code:
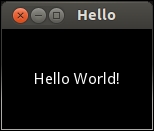
So, is Kivy just another library for Python? Well, yes. But as part of the library, Kivy offers its own language in order to separate the logic from the presentation and to link elements of the interface. Moreover, remember that this library will allow you to port your applications to many platforms.
Let's start to explore the Kivy language. We will separate the previous Python code into two files, one for the presentation (interface), and another for the logic. The first file includes the Python lines:
14. # File name: hello2.py 15. from kivy.app import App 16. from kivy.uix.button import Label 17. 18. class Hello2App(App): 19. def build(self): 20. return Label() 21. 22. if __name__=="__main__": 23. Hello2App().run()
The hello2.py
code is very similar to hello.py
. The difference is that the method build(self)
doesn't have the Hello World! message. Instead, the message has been moved to the text
property in the Kivy language file (hello2.kv
).
Note
A property is an attribute that can be used to change the content, appearance, or behavior of a widget.
The following is the code (rules) of hello2.kv
, which shows how we modify the Label
content with the text
property (line 27):
24. # File name: hello2.kv 25. #:kivy 1.9.0 26. <Label>: 27. text: 'Hello World!'
You might wonder how Python or Kivy knows that these two files (hello2.py
and hello2.kv
) are related. This tends to be confusing at the beginning. The key is in the name of the subclass of App
, which in this case is HelloApp
.
Note
The beginning part of the App
class's subclass name must coincide with the name of the Kivy file. For example, if the definition of the class is class FooApp(App)
, then the name of the file has to be foo.kv
and in the same directory of the main file (the one that executes the run()
method of App
).
Once that consideration is included, this example can be run in the same way we ran the previous one. We just need to be sure we are calling the main file – python hello2.py -–size=150x100
.
This is our first contact with the Kivy language, so we should have an in-depth look at it. Line 25 (hello2.kv
) tells Python the minimal version of Kivy that should be used. It does the same thing as the previous lines 2 and 3 do in hello.py
. The instructions that start with #:
in the header of a Kivy language are called directives. We will also be omitting the version directive throughout the rest of this book, but remember to include it in your own projects.
The <Label>:
rule (line 26) indicates that we are going to modify the Label
class.
Note
The Kivy language is expressed as a sequence of rules. A rule is a piece of code that defines the content, behavior, and appearance of a Kivy widget class. A rule always starts with a widget class name in angle brackets followed by a colon, like this, <Widget Class>:
Inside the rule, we set the text
property with 'Hello World!'
(line 27). The code in this section will generate the same output screen as before. In general, everything in Kivy can be done using pure Python and importing the necessary classes from the Kivy library, as we did in the first example (hello.py
). However, there are many advantages of using the Kivy language and therefore this book explains all the presentation programming in the Kivy language, unless we need to add dynamic components, in which case using Kivy as a traditional Python library is more appropriate.
If you are an experienced programmer, you might have worried that modifying the Label
class affects all the instances we could potentially create from Label
, and therefore they will all contain the same Hello World
text. That is true, and we are going to study a better approach to doing this in the following section.