As Qt expanded over time, its structure evolved. At first, it was just a single library, then a set of libraries. When it became harder to maintain and update for the growing number of platforms that it supported, a decision was made to split the framework into much smaller modules contained in two module groups—Qt Essentials and Qt Add-ons. A major decision relating to the split was that each module could now have its own independent release schedule.
Structure of Qt framework
Qt Essentials
The Essentials group contains modules that are mandatory to implement for every supported platform. This implies that if you are implementing your system using modules from this group only, you can be sure that it can be easily ported to any other platform that Qt supports. The most important relations between Qt Essentials modules are shown in the following diagram:
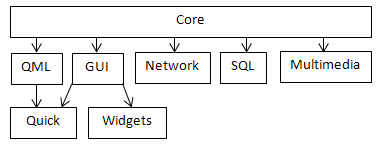
Some of the modules are explained as follows:
- The Qt Core module contains the most basic Qt functionality that all other modules rely on. It provides support for event processing, meta-objects, data I/O, text processing, and threading. It also brings a number of frameworks, such as the Animation framework, the State Machine framework, and the Plugin framework.
- The Qt GUI module provides basic cross-platform support to build user interfaces. It contains the common functionality required by more high-level GUI modules (Qt Widgets and Qt Quick). Qt GUI contains classes that are used to manipulate windows that can be rendered using either the raster engine or OpenGL. Qt supports desktop OpenGL as well as OpenGL ES 1.1 and 2.0.
- Qt Widgets extends the GUI module with the ability to create a user interface using widgets, such as buttons, edit boxes, labels, data views, dialog boxes, menus, and toolbars, which are arranged using a special layout engine. Qt Widgets utilizes Qt's event system to handle input events in a cross-platform way. This module also contains the implementation of an object-oriented 2D graphics canvas called Graphics View.
- Qt Quick is an extension of Qt GUI, which provides a means to create lightweight fluid user interfaces using QML. It is described in more detail later in this chapter, as well as in Chapter 11, Introduction to Qt Quick.
- Qt QML is an implementation of the QML language used in Qt Quick. It also provides API to integrate custom C++ types into QML's JavaScript engine and to integrate QML code with C++.
- Qt Network brings support for IPv4 and IPv6 networking using TCP and UDP. It also contains HTTP, HTTPS, FTP clients, and it extends support for DNS lookups.
- Qt Multimedia allows programmers to access audio and video hardware (including cameras and FM radio) to record and play multimedia content. It also features 3D positional audio support.
- Qt SQL brings a framework that is used to manipulate SQL databases in an abstract way.
Qt Add-ons
This group contains modules that are optional for any platform. This means that if a particular functionality is not available on some platform or there is nobody willing to spend time working on this functionality for a platform, it will not prevent Qt from supporting this platform. We'll mention some of the most important modules here:
- Qt Concurrent: This handles multi-threaded processing
- Qt 3D: This provides high-level OpenGL building blocks
- Qt Gamepad: This enables applications to support gamepad hardware
- Qt D-Bus: This allows your application to communicate with others via the D-Bus mechanism
- Qt XML Patterns: This helps us to access XML data
Many other modules are also available, but we will not cover them here.
qmake
Some Qt features require additional build steps during the compilation and linking of the project. For example, Meta-Object Compiler (moc), User Interface Compiler (uic), and Resource Compiler (rcc) may need to be executed to handle Qt's C++ extensions and features. For convenience, Qt provides the qmake executable that manages your Qt project and generates files required for building it on the current platform (such as Makefile for the make utility). qmake reads the project's configuration from a project file with the .pro extension. Qt Creator (the IDE that comes with Qt) automatically creates and updates that file, but it can be edited manually to alter the build process.
Alternatively, CMake can be used to organize and build the project. Qt provides CMake plugins for performing all the necessary build actions. Qt Creator also has fairly good support for CMake projects. CMake is more advanced and powerful than qmake, but it's probably not needed for projects with a simple build process.
Modern C++ standards
You can use modern C++ in your Qt projects. Qt's build tool (qmake) allows you to specify the C++ standard you want to target. Qt itself introduces an improved and extended API by using new C++ features when possible. For example, it uses ref-qualified member functions and introduces methods accepting initializer lists and rvalue references. It also introduces new macros that help you deal with compilers that may or may not support new standards.
If you use a recent C++ revision, you have to pay attention to the compiler versions you use across the target platforms because older compilers may not support the new standard. In this book, we will assume C++11 support, as it is widely available already. Thus, we'll use C++11 features in our code, such as range-based for loops, scoped enumerations, and lambda expressions.