The last demo of basic LED programming is to work with an RGB LED. This LED can emit monochromatic light, which could be one of the three primary colors—red, green, and blue, known as RGB.
The RGB LED connection is shown in the following figure:
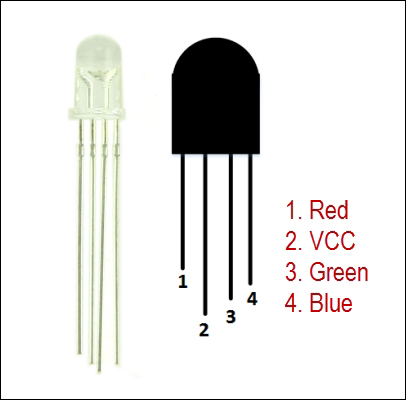
In this section, we will build a simple program to display red, green, and blue colors through the RGB LED.
The following hardware components are needed:
A Raspberry Pi 2 board
An RGB LED (https://www.sparkfun.com/products/9264).
Our hardware wiring can be implemented as follows:
RGB LED pin 1 is connected to Raspberry Pi GPIO18
RGB LED pin 2 is connected to Raspberry Pi VCC +3 V
RGB LED pin 3 is connected to Raspberry Pi GPIO23
RGB LED pin 4 is connected to Raspberry Pi GPIO24
The complete hardware wiring can be seen in the following figure:
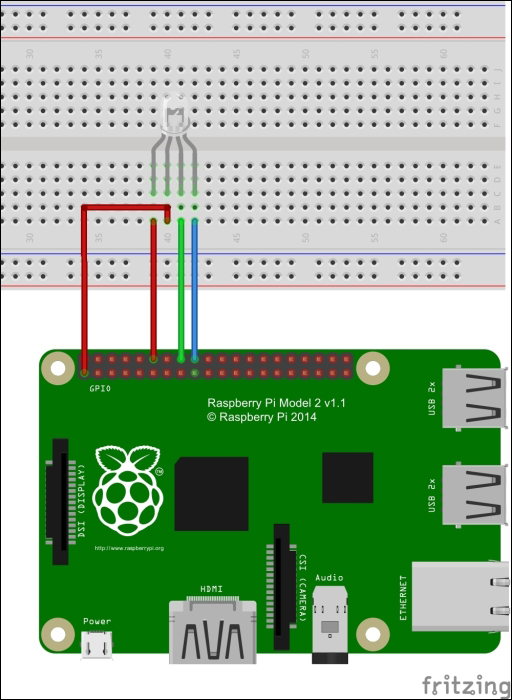
Returning to the Raspberry Pi terminal, you could write a Python program to display color through RGB LED. Let's create a file named ch01_03.py
and write this script as follows:
# ch01_03.py file import wiringpi2 as wiringpi import time # initialize wiringpi.wiringPiSetup() # define GPIO mode GPIO18 = 1 # red GPIO23 = 4 # green GPIO24 = 5 # blue LOW = 0 HIGH = 1 OUTPUT = 1 wiringpi.pinMode(GPIO18, OUTPUT) wiringpi.pinMode(GPIO23, OUTPUT) wiringpi.pinMode(GPIO24, OUTPUT) # make all LEDs off def clear_all(): wiringpi.digitalWrite(GPIO18, HIGH) wiringpi.digitalWrite(GPIO23, HIGH) wiringpi.digitalWrite(GPIO24, HIGH) def display(red, green, blue): wiringpi.digitalWrite(GPIO18, red) wiringpi.digitalWrite(GPIO23, green) wiringpi.digitalWrite(GPIO24, blue) try: while 1: clear_all() print("red") display(0, 1, 1) time.sleep(2) clear_all() print("green") display(1, 0, 1) time.sleep(2) clear_all() print("blue") display(1, 1, 0) time.sleep(2) clear_all() print("white") display(0, 0, 0) time.sleep(2) clear_all() print("110") display(1, 1, 0) time.sleep(2) clear_all() print("101") display(1, 0, 1) time.sleep(2) clear_all() print("011") display(0, 1, 1) time.sleep(2) except KeyboardInterrupt: clear_all() print("done")
Save this script. You can run this file by typing the following command:
$ sudo python ch01_03.py
Then, you should see that the RGB LED displays a certain color every second. The program output can also write a message indicating which color is currently on the RGB LED:
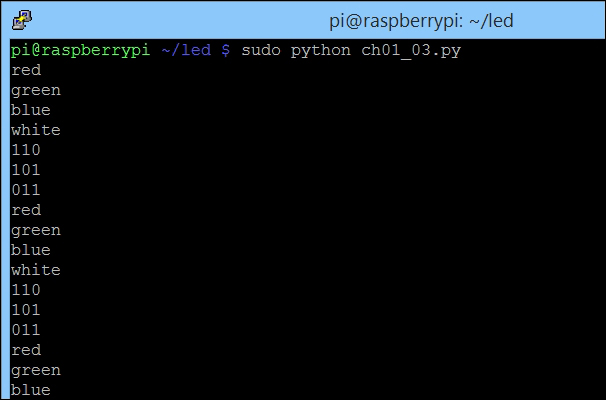
The RGB LED can display a color by combining three basic colors: red, green, and blue. First, we initialize Raspberry Pi GPIO and define our GPIO usage:
# initialize wiringpi.wiringPiSetup() # define GPIO mode GPIO18 = 1 # red GPIO23 = 4 # green GPIO24 = 5 # blue LOW = 0 HIGH = 1 OUTPUT = 1 wiringpi.pinMode(GPIO18, OUTPUT) wiringpi.pinMode(GPIO23, OUTPUT) wiringpi.pinMode(GPIO24, OUTPUT)
For instance, to set a red color, we should set LOW
on the red pin and HIGH
on both green and blue pins. We define the display()
function to display a certain color on the RGB LED with the red, green, and blue values as parameters as follows:
def display(red, green, blue): wiringpi.digitalWrite(GPIO18, red) wiringpi.digitalWrite(GPIO23, green) wiringpi.digitalWrite(GPIO24, blue)
In the main program, we display a color via the display()
function by passing red, green, and blue values, as shown in the following code:
clear_all() print("red") display(0, 1, 1) time.sleep(2) clear_all() print("green") display(1, 0, 1) time.sleep(2) clear_all() print("blue") display(1, 1, 0) time.sleep(2) clear_all() print("white") display(0, 0, 0) time.sleep(2) clear_all() print("110") display(1, 1, 0) time.sleep(2) clear_all() print("101") display(1, 0, 1) time.sleep(2) clear_all() print("011") display(0, 1, 1) time.sleep(2)