Android as a platform has certain features built into its architecture that ensure the security of users, applications, and data. Although they help in protecting the data, these security features sometimes prevent investigators from gaining access to necessary data. Hence, from a forensic perspective, it is first important to understand the inherent security features so that a clear idea is established about what can or cannot be accessed under normal circumstances. The security features and offerings that are incorporated aim to achieve three things:
To protect user data
To protect system resources
To make sure that one application cannot access the data of another application
The next sections provide an overview of the key security features in the Android operating system.
The Android operating system is built on top of the Linux kernel. Over the past few years, Linux has evolved into a secure operating system trusted by many corporations across the world for its security. Today, most of the mission critical systems and servers run on Linux because of its security. By having the Linux kernel at the heart of its platform, Android tries to ensure security at the OS level. Also, Android has built a lot of specific code into Linux to include certain features related to mobile environment. With each Android release the kernel version also has changed. The following table shows Android versions and the corresponding Linux kernel version:
Android Version |
Linux Kernel Version |
---|---|
1.0 |
2.6.25 |
1.5 |
2.6.27 |
1.6 |
2.6.29 |
2.2 |
2.6.32 |
2.3 |
2.6.35 |
3.0 |
2.6.36 |
4.0 |
3.0.1 |
4.1 |
3.0.31 |
4.2 |
3.4.0 |
4.3 |
3.4.39 |
4.4 |
3.8 |
Linux kernel used in various Android versions
The Linux kernel provides Android with the below key security features:
A user-based permissions model
Process isolation
Extensible mechanism for secure IPC
Android implements a permission model for individual apps. Applications must declare which permissions (in the manifest file) they require. When the application is installed, as shown in the following screenshot, Android will present the list to the user so that they can view the list to allow installation or not:
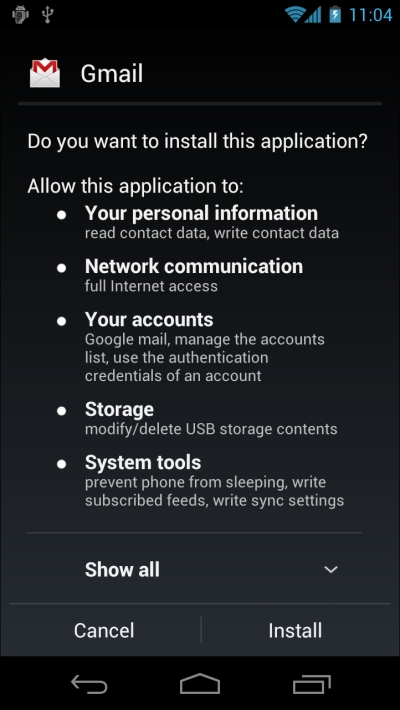
Sample permission model in Android
Unlike a desktop environment, this provides an opportunity for the user to know in advance which resources the application is seeking access to. In other words, user permission is a must to access any kind of critical resource on the device. By looking at the requested permission, the user is more aware of the risks involved in installing the application. But most users do not read these and just give away a lot of permissions, exposing the device to malicious activities.
Note
It is not possible to install an Android app with a few or reduced permissions. You can either install the app with all the permissions or decline it.
As mentioned earlier, developers have to mention permissions in a file named AndroidManifest.xml
. For example, if the application needs to access the Internet, the permission INTERNET
is specified using the following code in the AndroidManifest.xml
file:
<manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.example.rohit"> <uses-permission android:name="android.permission.INTERNET" /> </manifest>
Android permissions are categorized into four levels which are as follows:
Permission Type |
Description |
---|---|
Normal |
This is the default value. These are low risk permissions and do not pose a risk to other applications, system or user. This permission is automatically granted to the app without asking for user approval during installation. |
Dangerous |
These are the permissions that can cause harm to the system and other applications. Hence, user approval is necessary during installation. |
Signature |
These are automatically granted to a requesting app if that app is signed by the same certificate as the one that declared/created the permission. This level is designed to allow apps that are part of a suite, or otherwise related, to share data. |
Signature/System |
A permission that the system grants only to the applications that are in the Android system image, or that are signed with the same certificate as the application that declared the permission. |
In order to isolate applications from each other, Android takes advantage of the Linux user-based protection model. In Linux systems, each user is assigned a unique user ID (UID) and users are segregated so that one user does not access the data of another user. All resources under a particular user are run with the same privileges. Similarly, each Android application is assigned a UID and is run as a separate process. What this means is that even if an installed application tries to do something malicious, it can do it only within its context and with the permissions it has.
This application sandboxing is done at the kernel level. The security between applications and the system at the process level is ensured through standard Linux facilities such as user and group IDs that are assigned to applications. This is shown in the following screenshot, referenced from http://www.ibm.com/developerworks/library/x-androidsecurity/.
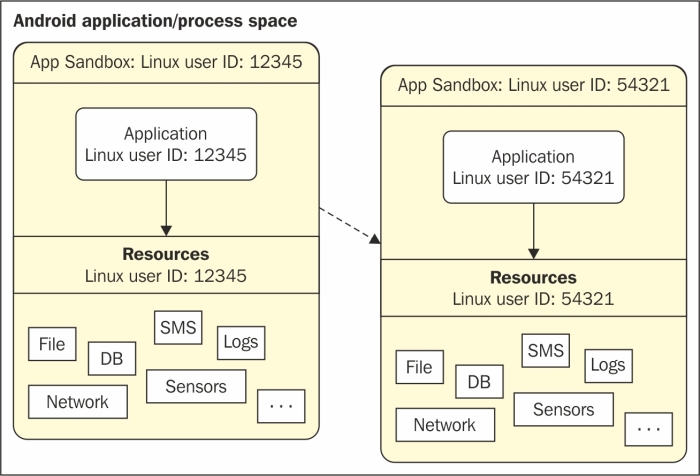
Two applications on different processes on with different UID's
By default, applications cannot read or access the data of other applications and have limited access to the operating system. If application A tries to read application B's data, for example, then the operating system protects against this because application A does not have the appropriate privileges. Since the application sandbox mechanism is implemented at the kernel level, it applies to both native applications and OS applications. Thus the operating system libraries, application framework, application runtime, and all applications run within the Application Sandbox. Bypassing this sandbox mechanism would require compromising the security of Linux kernel.
Starting with Android 4.3, Security-Enhanced Linux (SELinux) is supported by the Android security model. Android security is based on discretionary access control, which means that applications can ask for permissions, and users can grant or deny those permissions. Thus, malware can create havoc on phones by gaining permissions. But SE Android uses mandatory access control (MAC) which ensures that applications work in isolated environments. Hence, even if a user installs a malware app, the malware cannot access the OS and corrupt the device. SELinux is used to enforce MAC over all processes, including the ones running with root privileges.
SELinux operates on the principle of default denial. Anything that is not explicitly allowed is denied. SELinux can operate in one of two global modes:
permissive mode, in which permission denials are logged but not enforced, and
enforcing mode, in which denials are both logged and enforced. As per Google's documentation, in the Android 5.0 Lollipop release, Android moves to full enforcement of SELinux. This builds upon the permissive release of 4.3 and the partial enforcement of 4.4. In short, Android is shifting from enforcement on a limited set of crucial domains (installd
, netd
, vold
and zygote
) to everything (more than 60 domains).
All Android apps need to be digitally signed with a certificate before they can be installed on a device. The main purpose of using certificates is to identify the author of an app. These certificates do not need to be signed by a certificate authority and Android apps often use self-signed certificates. The app developer holds the certificate's private key. Using the same private key, the developer can provide updates to his applications and share data between applications. In debug mode, developers can sign the app with a debug certificate generated by the Android SDK tools. You can run and debug an app signed in debug mode but the app cannot be distributed. To distribute an app, the app needs to be signed with your own certificate. The key store and the private key which are used during this process need to be secured by the developer as they are essential to push updates. The following screenshot shows the key store selection option that is displayed while exporting the application:
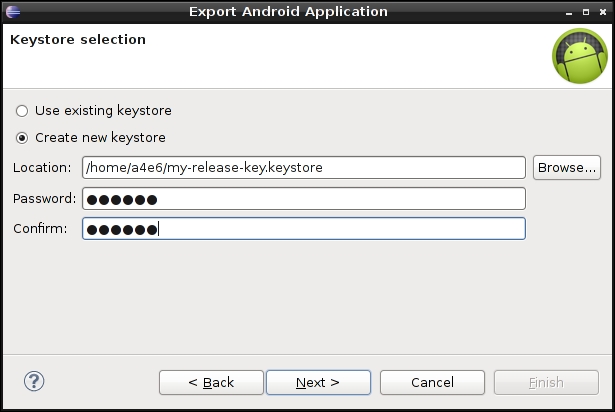
Keystore selection while exporting Android app
As discussed in the above sections, sandboxing of the apps is achieved by running apps in different processes with different Linux identities. System services run in separate processes and have more privileges. Thus, in order to organize data and signals between these processes, an interprocess communication (IPC) framework is needed. In Android, this is achieved with the use of the Binder mechanism.
The Binder framework in Android provides the capabilities required to organize all types of communication between various processes. Android application components, such as intents and content providers, are also built on top of this Binder framework. Using this framework, it is possible to perform a variety of actions such as invoking methods on remote objects as if they were local, synchronous and asynchronous method invocation, sending file descriptors across processes, and so on. Let us suppose the application in Process 'A' wants to use certain behavior exposed by a service which runs in Process 'B'. In this case, Process 'A' is the client and Process 'B' is the service. The communication model using Binder is shown in the following diagram:
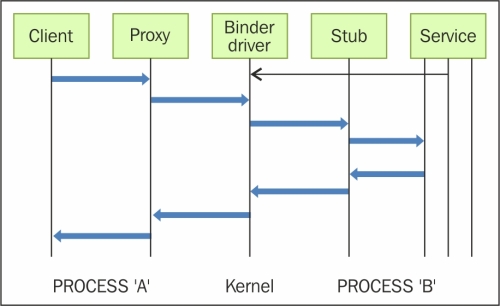
Binder Communication Model
All communication between the processes using the Binder framework occurs through the /dev/binder
Linux kernel driver. The permissions to this device driver are set to world readable and writable. Hence, any application may write to and read from this device driver. All communications between the client and server happen through proxies on the client side and stubs on the server side. The proxies and the stubs are responsible for sending and receiving the data, and the commands, sent over the Binder driver.
Each service (also called a Binder service) exposed using the Binder mechanism is assigned with a token. This token is a 32-bit value and is unique across all processes in the system. A client can start interacting with the service after discovering this value. This is possible with the help of Binder's context manager. Basically, the context manager acts as a name service, providing the handle of a service using the name of this service. In order to get this process working, each service must be registered with the context manager. Thus, a client needs to know only the name of a service to communicate. The name is resolved by the context manager and the client receives the token that is later used for communicating with the service. The Binder driver adds the UID and the PID value of the sender process to each transaction. As discussed earlier, each application in the system has its own UID and this value is used to identify the calling party. The receiver of the call may check the obtained values and decide if the transaction should be completed. Thus, the security is enforced, with the Binder token acting as a security token as it is unique across all processes.
Android is compatible with a wide range of hardware components. Having a Linux kernel made this easy, as Linux supports large variety of hardware. This gives manufacturers a lot of flexibility as they can design based on their requirement, without worrying about compatibility. This poses a significant challenge for forensic analysts during investigations. Thus, understanding the hardware components and device types would greatly help in understanding Android forensics.
The components present in a device change from one manufacturer to another and also from one model to another. However, there are some components which are found in most mobile devices. The following sections provide an overview of the commonly-found components of an Android device.
The central processing unit (CPU), also known as processor, is responsible for executing everything that happens on a mobile device. It tells the device what to do and how to do it. Its performance is measured based on the number of tasks it can complete per second, known as a cycle. For example, 1 GHz processor can process one billion cycles per second. The higher the capacity of the processor, the smoother the performance of the phone will be.
When dealing with smart phones, we come across the following terminologies—ARM, x86 (Intel), MIPS, Cortex, A5, A7, or A9. ARM is the name of a company that licenses their architectures (branded Cortex) with different models coming up each year such as the aforementioned A series (A5, A7, and A9). Based on these architectures, chip makers release their own series of chipsets (Snapdragon, Exynos, and so on) which are used in mobile devices. The latest smartphones are powered by dual core, quad core and even octa core processors.
Smartphones today support a variety of cellular protocols, including GSM, 3G, 4G and 4G LTE. These protocols are complicated and require a large amount of CPU power to process data, generate packets and transmit them to the network provider. To handle this process, smartphones now use a baseband modem which is a separate chip included in smartphones that communicates with the main processor. These baseband modems have their own processor called the baseband processor and run their own operating system. The baseband processor manages several radio control functions such as signal generation, modulation, encoding, as well as frequency shifting. It can also manage the transmission of signals.
The baseband processor is generally located on the same circuit board as the CPU but consists of a separate radio electronics component.
Android phones, just like normal computers, use two primary types of memory–random access memory (RAM) and read-only memory (ROM). Although most users are familiar with these concepts, there is some confusion when it comes to mobile devices.
RAM is volatile, which means its contents are erased when the power is removed. RAM is very fast to access and is used primarily for the runtime memory of software applications (including the device's operating system and any applications). In other words, it is used by the system to load and execute the OS and other applications. So the number of applications and processes that can be run simultaneously depends on this RAM size.
ROM (commonly referred to as Android ROM) is non-volatile, which means it retains the contents even when the power is off. Android ROM contains the boot loader, OS, all downloaded applications and their data, settings and, so on.
Note that the part of memory that is used for the boot loader is normally locked and can only be changed through a firmware upgrade. The remaining part of the memory is termed by some manufacturers as user memory. The data of each application stored here will not be accessible to other applications. Once this memory gets filled up, the device slows down. Both RAM and Android ROM are manufactured into a single component called as Multichip Package (MCP).
The SD card has great significance with respect to mobile forensics because, quite often, data that is stored in these can be vital evidence. Many Android devices have a removable memory card commonly referred to as their Secure Digital (SD) card. This is in contrast to Apple's iPhone which does not have any provision for SD cards. SD cards are non-volatile, which means data is stored in it even when it is powered off. SD cards use flash memory, a type of Electrically Erasable Programmable Read-Only Memory (EEPROM) that is erased and written in large blocks instead of individual bytes. Most of the multimedia data and large files are stored by the apps in SD card. In order to interoperate with other devices, SD cards implement certain communication protocols and specifications.
In some mobile devices, although an SD card interface is present, some portion of the on-board NAND memory (non-volatile) is carved out for creating an emulated SD card. This essentially means the SD card is not removable. Hence, forensic analysts need to check whether they are dealing with the actual SD card or an emulated SD card. SD memory cards come in several different sizes. Mini-SD card and micro-SD card contain the same underlying technology as the original SD memory card, but are smaller in size.
Mobile phone screens have progressed dramatically over the last few years. Below is a brief description of some of the widely used types of mobile screens as described at http://www.in.techradar.com/news/phone-and-communications/mobile-phones/Best-phone-screen-display-tech-explained/articleshow/38997644.cms.
The thin film transistor liquid crystal display (TFT LCD) is the most common type of screen found in mobile phones. These screens have a light underneath them which shines through the pixels to make them visible.
The active-matrix organic light-emitting diode (AMOLED) is a technology based on organic compounds and known for its best image quality while consuming low power. Unlike LCD screens, AMOLED displays don't need a backlight; each pixel produces its own light, so phones using them can potentially be thinner.
Battery is the lifeblood of a mobile phone and is one of the major concerns with modern smartphones. The more you use the device and its components, the more battery is consumed. The following different types of batteries are used in mobile phones:
Lithium Ion (Li-ion): These batteries are the most popular batteries used in cell phones as they are light and portable. They are well known for their high energy density and low maintenance. However, they are expensive to manufacture compared to other battery types.
Lithium Polymer (Li-Polymer): These batteries have all the attributes of a Lithium Ion battery but with ultra slim geometry and simplified packaging. They are the very latest and found only in few mobile devices.
Nickel Cadmium (NiCd): These batteries are old technology batteries and suffer from memory effect. As a result, the overall capacity and the lifespan of the battery are reduced. In addition to this, NiCd batteries are made from toxic materials that are not environment-friendly.
Nickel Metal Hydrid (NiMH): These batteries are same as the NiCd batteries, but can contain higher energy and run longer, between 30 and 40 percent. They still suffer from memory effect, but comparatively less than the NiCd batteries. They are widely used in mobile phones and are affordable too.
The battery type can be found by looking at the details present on its body. For example, the following is an image of a Li-ion battery:
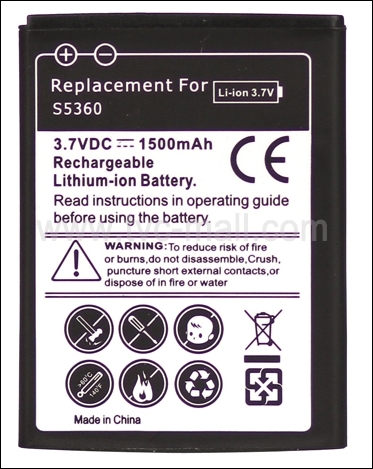
Lithium-ion battery
Most SD cards are located behind the battery. During forensic analysis, accessing an SD card would require removing the battery which would power off the device. This can have certain implications which will be discussed in detail in later chapters.
Apart from the components described above, here are some of the other components that are well known:
Global Positioning System
Wi-Fi
Near field communication
Bluetooth
Camera
Keypad
USB
Accelerometer and gyroscope
Speaker
Microphone
Understanding the boot process of an Android device will help us to understand other forensic techniques which involve interacting with the device at various levels. When an Android device is first powered on, there is a sequence of steps that are executed, helping the device to load necessary firmware, OS, application data, and so on into memory. The following information is compiled from the original post published at http://www.androidenea.com/2009/06/android-boot-process-from-power-on.html.
The sequence of steps involved in Android boot process is as follows:
Boot ROM code execution
The boot loader
The Linux kernel
The init process
Zygote and Dalvik
The system server
We will examine each of these steps in detail.
Before the device is powered on, the device CPU will be in a state where no initializations will have taken place. Once the Android device is powered on, execution starts with the boot ROM code. This boot ROM code is specific to the CPU the device is using. As shown in the the following diagram, this phase includes two steps:
When, boot ROM code is executed, it initializes the device hardware and tries to detect the boot media. Thus, the boot ROM code scans till it finds the boot media. This is similar to the BIOS function in the boot process of a computer.
Once the boot sequence is established, the initial boot loader is copied to the internal RAM. After this, the execution shifts to the code loaded into the RAM.
Android boot process: Boot ROM code execution
The boot loader is a piece of program that is executed before the operating system starts to function. Boot loaders are present in desktop computers, laptops and mobile devices as well. In an Android boot loader, there are two stages—initial program load (IPL) and second program load (SPL). As shown in the following diagram, this involves three steps explained as follows:
IPL deals with detecting and setting up external RAM.
Once external RAM is available, the SPL is copied into the RAM and execution is transferred to it. The SPL is responsible for loading the Android operating system. It also provides access to other boot modes such as fastboot, recovery, and so on. It initiates several hardware components such as console, display, keyboard and file systems, virtual memory, and other features.
After this, the SPL tries to look for the Linux kernel. It will load this from the boot media and copy it to the RAM. Once the boot loader is done with this process, it transfers the execution to the kernel.
Android boot process: The boot loader
The Linux kernel is the heart of the Android operating system and is responsible for process management, memory management, and enforcing security on the device. After the kernel is loaded, it mounts the root file system (rootfs) and provides access to system and user data, as described in the following steps:
The init is the very first process that starts and is the root process of all other processes.
The init process will look for a script named
init.rc
that describes the system services, file system, and any other parameters that need to be set up.The
init
process can be found at:<android source>/system/core/init
..The
init.rc
file can be found in source tree at<android source>/system/core/rootdir/init.rc
.Tip
More details about the Android file hierarchy will be covered in Chapter 3, Understanding Data Storage on Android Devices.
The
init
process will parse theinit.rc
script and launch the system service processes. At this stage, you will see the Android logo on the device screen.Android boot process: The init process
Zygote is one of the first init processes created after the device boots. It initializes the Dalvik virtual machine and tries to create multiple instances to support each android process. As discussed in earlier sections, the Dalvik virtual machine is the virtual machine which executes Android applications written in Java.
Zygote facilitates using a shared code across the VM, thus helping to save the memory and reduce the burden on the system. After this, applications can run by requesting new Dalvik virtual machines that each one runs in. Zygote registers a server socket for zygote connections, and also preloads certain classes and resources. This Zygote loading process has been more clearly explained at http://www.kpbird.com/2012/11/in-depth-android-boot-sequence-process.html. This is also explained as follows:
Load ZygoteInitclass
: This class loads theZygoteInit
class. Source Code:<Android Source>/frameworks/base/core/java/com/android/internal/os/ZygoteInit.java
.registerZygoteSocket()
: This registers a server socket for zygote command connections.preloadClasses()
: This is a simple text file containing the list of classes that need to be preloaded will be executed here. This file can be seen at<Android Source>/frameworks/base
.preloadResources()
: This deals with native themes and layouts. Everything that includes theandroid.R
file will be loaded using this method.Android boot process: The Zygote
All the core features of the device such as telephony, network, and other important functions, are started by the system server, as shown in the following diagram:
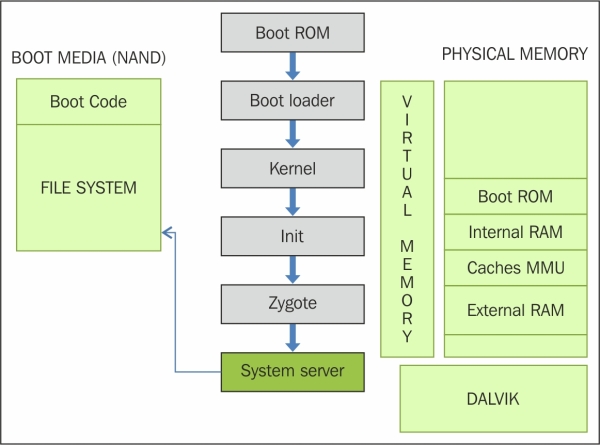
Android boot process: System server
The following core services are started in this process:
Start Power Manager
Create Activity Manager
Start Telephony Registry
Start Package Manager
Set Activity Manager Service as System Process
Start Context Manager
Start System Context Providers
Start Battery Service
Start Alarm Manager
Start Sensor Service
Start Window Manager
Start Bluetooth Service
Start Mount Service
The system sends a broadcast action called ACTION_BOOT_COMPLETED
which informs all the dependent processes that the boot process is complete. After this, the device displays the home screen and is ready to interact with the user. The Android system is now fully operational and is ready to interact with the user.
As explained earlier, several manufacturers use the Android operating system on their devices. Most of these device manufacturers customize the OS based on their hardware and other requirements. Hence, when a new version of Android is released, these device manufacturers have to port their custom software and tweaks to the latest version.
Understanding Android architecture and its security model is crucial to having a proper understanding of Android forensics. The inherent security features in Android OS, such as application sandboxing, permission model, and so on, safeguard Android devices from various threats and also act as an obstacle for forensic experts during investigation. Having gained this knowledge of Android internals, we will discuss more about what type of data is stored on the device and how it is stored, in the next chapter.