Let's make sure that we have installed the prerequisites. Here is a table that summarizes the basic tools we need for this chapter and beyond; more verbose installation instructions follow in the next section:
Tool |
Notes |
---|---|
Python 3.5 |
The code illustrated in this book is compatible with version 3.5. See the next table for available Python distributions. Supporting code bundles also provide 2.7.9 compatible files. |
pip (package manager for Python) |
The pip is already available in the official distribution for versions 3.5 and 2.7.9. |
IPython |
Optional installation. IPython is an enhanced Python interpreter. |
Integrated development environment (IDE) |
Use the Python editor or any IDE of your choice. Some good IDEs are listed in a table later in this chapter. |
In subsequent chapters, we will need to install some additional dependencies. The Python package manager (pip) will makes this a trivial task.
Tip
Have you already set up the required Python environment or know how to do it? Just skip the setup instructions that follow and move on to the The theme of the book section, where the real action begins!
There are two options to install Python. You can either use the official Python version or one of the freely available bundled distributions.
For Linux or Mac users, Python is probably already installed on your system. If not, you can install it using the package manager of your operating system. Windows OS users can install Python 3.5 by downloading the Python installer from the official Python website:
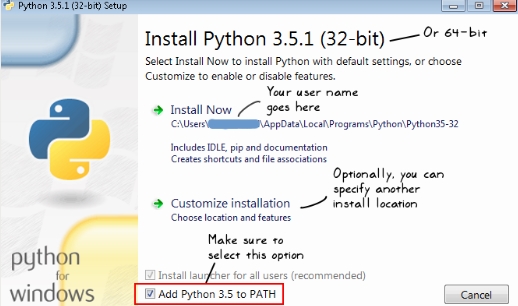
During the installation process, just make sure to select the option that adds Python 3.5 to the system environment variable, PATH
, as shown in the preceding screenshot. You can also visit the official Python website, https://www.python.org/downloads, to get the platform-specific distribution.
Alternatively, there are several freely available Python distributions that bundle together useful Python packages, including pip and IPython. The following table summarizes some of the most popular Python distributions, including the official one:
Distribution |
Supported platforms |
Notes |
---|---|---|
| ||
Anaconda | ||
Windows, Linux, Mac | ||
Python(x, y) |
Windows |
Let's briefly talk about the path where Python is installed, and how to make sure python
is available as a command in your terminal window. Of course, things will widely vary, depending on where you install it and which Python distribution you choose.
Tip
The official Python documentation page has comprehensive information on setting up the Python environment on different platforms. Here is a link, in case you need further help beyond what we have covered: https://docs.python.org/3/using/index.html.
On a Unix-like operating system such as Linux, the default location is typically /usr/bin/python
or /usr/local/bin/python
.
If you used your operating system's package manager to install Python, the command python
or python3
should be available in the terminal window. If it isn't, you need to update the PATH
system environment variable to include the directory path to the Python executable. For example, if you have a
Bash shell, add the following to the .bashrc
file in your user home directory:
export PATH=$PATH:/usr/bin/
Specify the actual path to your Python installation in place of /usr/bin
.
On Windows OS, the default Python installation path is typically the following directory: C:\Users\name\AppData\Local\Programs\Python\Python35-32\python.exe
. Replace name
with your Windows username. Depending on your installer and system, the Python directory can also be Python35-64
. As mentioned earlier, at the time of installation, you should select the option Add Python 3.5 to PATH to make sure python
or python.exe
are automatically recognized as commands. Alternatively, you can rerun the installer with just this option checked.
Open a terminal window (or command prompt on Windows OS) and type the following command to verify the Python version. This command will work if Python is installed and is available as a command in the terminal window. Otherwise, specify the full path to the Python executable. For instance, on Linux you can specify it as /usr/bin/python
, if Python is installed in /usr/bin
:
$ python -V
Tip
Note that the $
sign in the previous command line belongs to the terminal window and is not part of the command itself! Put another way, the actual command is just python -V
. The $
or %
sign in the terminal window is a prompt for a normal user on Linux. For a root (admin) user, the sign is #
. Likewise, on Windows OS, the corresponding symbol is >
. You will type the actual command after this symbol.
The following is just a sample output, if we run the preceding command:
[user@hostname ~]$ python -V Python 3.5.1 :: Anaconda 4.0.0 (64-bit)
The pip is a software package manager that makes it trivial to install Python packages from the official third party software repository, PyPI. The pip is already installed for Python-2 version 2.7.9 or higher and Python-3 version 3.4 or higher. If you are using a different Python version, check out https://pip.pypa.io/en/stable/installing for the installation instructions.
On Linux OS, the default location for the pip is same as that of the Python executable. For example, if you have /usr/bin/python
, then pip should be available as /usr/bin/pip
. On Windows OS, the default pip.exe
is typically the following: C:\Users\name\AppData\Local\Programs\Python\Python35-32\Scripts\pip.exe
. As mentioned earlier, replace name
with your Windows username. Depending on your installer and the system, the Python directory can also be Python35-64
.
This is an optional installation. IPython is an enhanced version of the Python interpreter. If it is not already bundled in your Python distribution, you can install it with:
$ pip install ipython
After the installation, just type ipython
in the terminal to start the IPython interactive shell. Here is a screenshot of the IPython shell using the Anaconda Python 3.5 distribution:
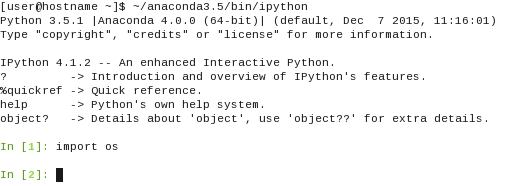
Tip
It is often very convenient to use the Jupyter Notebook to write and share interactive programs. It is a web application that enables an interactive environment for writing Python code alongside rich text, images, plots, and so on. For further details, check out the project homepage at http://jupyter.org/. The Jupyter Notebook can be installed with:
$ pip install "ipython[notebook]"
Using an IDE for development is a matter of personal preference. Simply put, an IDE is a tool intended to accelerate application development. It enables developers to write efficient code quickly by integrating the most common tools they need. The Python installation comes with a program called IDLE. It is a basic IDE for Python, which should get you started. For advanced development, you can choose from a number of freely or commercially available tools. Any good Python IDE has the following minimum features:
A source code editor with code completion and syntax highlighting features
A code browser to browse through files, projects, functions, and classes
A debugger to interactively identify problems
A version control system integration such as Git
You can get started by trying out one of the freely available IDEs. Here is a partial list of popular IDEs. If you are just interested in a simple source code editor, you can check out https://wiki.python.org/moin/PythonEditors, for a list of available choices.