In Python, functions and classes are first-class objects. The phrase first-class object is a fancy way of saying that the data values can be accessed, modified, stored, and otherwise manipulated by the program they are a part of. In Python, a function is just as much a data value as a text string is. The same goes for classes.
When a function definition statement is executed, it stores the resulting function in a variable with the name that was specified in the def statement, as shown in the following screenshot:
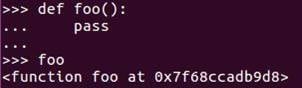
This variable isn't special; it's just like any other variable holding the value. This means that we can use it in expressions, assign the value to other values, or even store a different value in place of the original function.
The function value itself contains quite a few attribute variables, which we can access. More usefully, most of the time, we can add attributes to a function object, allowing us to store custom information about a function as part of the function and access that information later, as shown in the following code example:
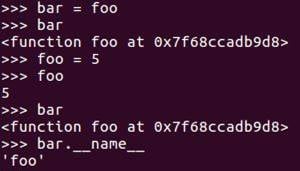
One common task that first-class functions make easy is assigning handlers to events. To bind the handler function to an event in Python, we just pass the function object as a parameter when we call the binding function, as shown here:

That's a significant improvement over the hoops that C++ or Java imposes on us to do something similar. As function definition statements, class definition statements create a class object and store it in a variable. This can be confusing at first. Classes describe the type of object, how can they be objects themselves?
Think of it this way-a blueprint for a house describes the type of building, but the blueprint is still a thing in its own, right? It's the same with class objects. This means that like function objects, class objects can be stored in variables, and otherwise, be treated as data values. Most interestingly, they could be used as parameters to function calls.