An exchange of facts
Three different uses or patterns exist that can be called EDA individually or altogether, as follows:
- Event notifications
- Event-carried state transfer
- Event sourcing
In this book, we will be covering each of these patterns, going over their uses and both when to use them and when you might not.
Event notifications
Events can be used to notify something has occurred within your application. A notification event typically carries the absolute minimum state, perhaps even just the identifier (ID) of an entity or the exact time of the occurrence of their payload. Components that are notified of these events may take any action they deem necessary. Events might be recorded locally for auditing purposes, or the component may make calls back to the originating component to fetch additional relevant information about the event.
Let’s see an example of PaymentReceived
as an event notification in Go, as follows:
type PaymentReceived struct {
PaymentID string
}
Here is how that notification might be used:
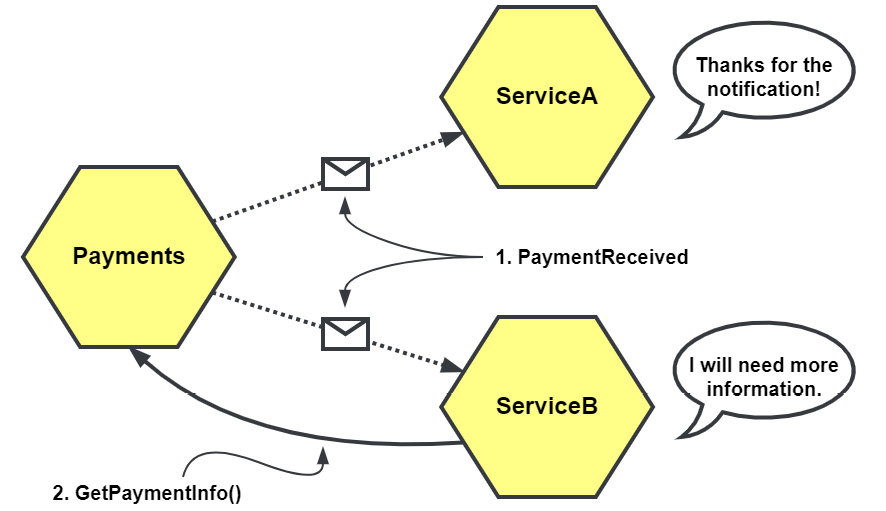
Figure 1.1 – PaymentReceived as an event notification
Figure 1.1 shows the PaymentReceived
notification being received by two different services. While ServiceA only needed to be notified of the event, ServiceB will require additional information and must make a call back to the Payments service to fetch it.
Event-carried state transfer
Event-carried state transfer is an asynchronous cousin to representational state transfer (REST). In contrast with REST’s on-demand pull model, event-carried state transfer is a push model where data changes are sent out to be consumed by any components that might be interested. The components may create their own local cached copies, negating any need to query the originating component to fetch any information to complete their work.
Let’s see an example of PaymentReceived
as an event-carried state transfer, as follows:
type PaymentReceived struct {
PaymentID string
CustomerID string
OrderID string
Amount int
}
In this example for event-carried state transfer, we’ve included some additional IDs and an amount collected, but more detail could be added to provide as much detail as possible, as illustrated in the following diagram:
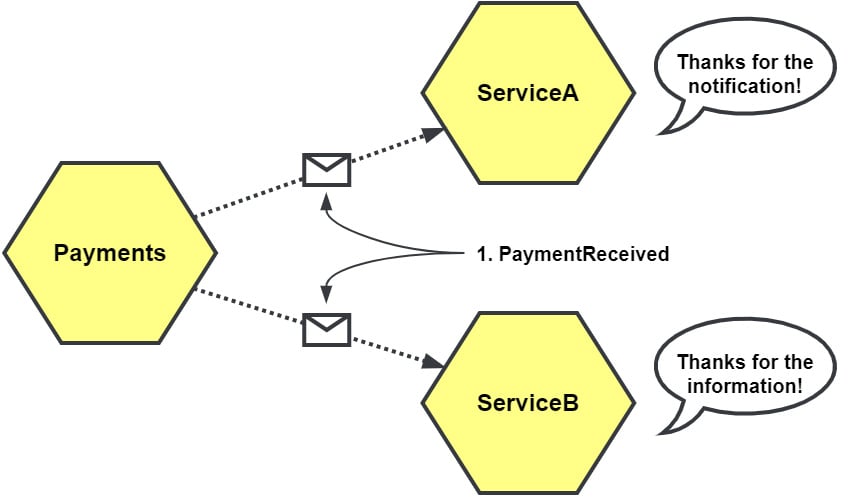
Figure 1.2 – PaymentReceived as an event-carried state change
When the PaymentReceived
event is sent with additional information, it changes how downstream services might react to it. We can see in Figure 1.2 that ServiceB no longer needs to call the Payments service because the event it has received already contains everything it requires.
Event sourcing
Instead of capturing changes as irreversible modifications to a single record, those changes are stored as events. These changes or streams of events can be read and processed to recreate the final state of an entity when it is needed again.
When we use event sourcing, we store the events in an event store rather than communicating them with other services, as illustrated in the following diagram:
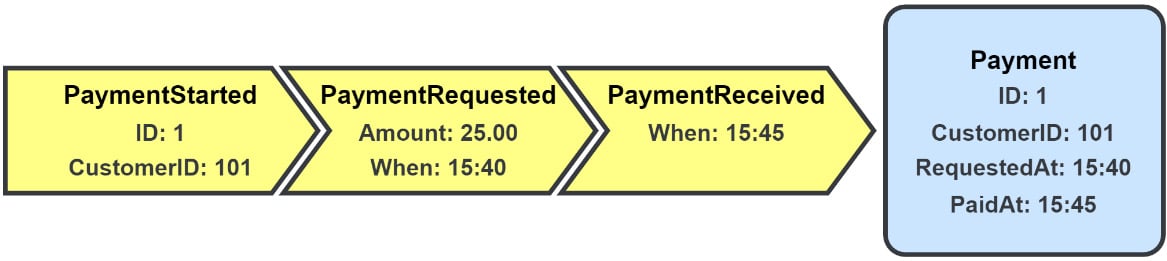
Figure 1.3 – Payment data recorded using event sourcing
In Figure 1.3, we see the entire history of our data is kept as individual entries in the event store. When we need to work with a payment in the application, we would read all the entries associated with that record and then perform a left fold of the entries to recreate the final state.
Core components
You will observe that four components are found at the center of all event patterns, as illustrated in the following diagram:
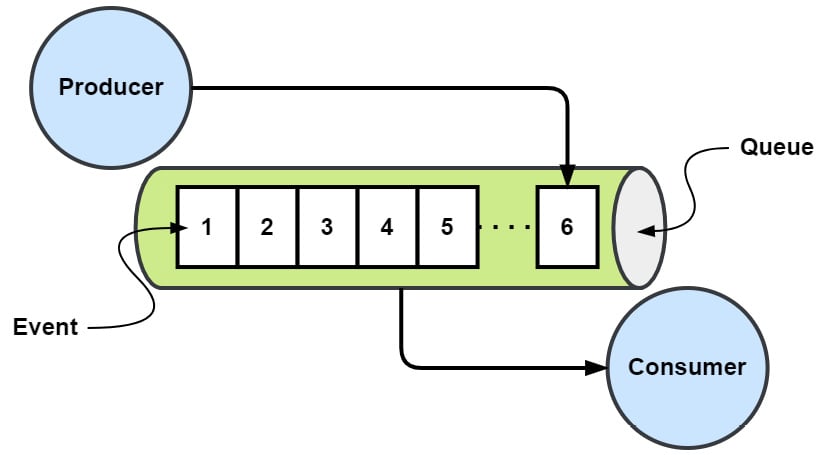
Figure 1.4 – Event, queue, producer, and consumer
Event
At the heart of EDA is the event. In EDA terms, it is an occurrence that has happened in the application. The event itself is in the past and it is an immutable fact. Some examples of events are customers signing up for your services, payments being received for orders, or failed authentication attempts for an account.
With EDA, the consumers of these events may know nothing about what caused the production of these events or have any relationship or connection with them, but only with the event itself.
In most languages, events are simple value objects that contain state. An event is equal to another if all the attributes are the same. In Go, we would represent an event with a simple struct, such as this one for PaymentReceived
:
type PaymentReceived struct {
PaymentID string
OrderID string
Amount int
}
Events should carry enough data to be useful in capturing the change in the application state that they’re meant to communicate. In the preceding example, we might expect that this event is associated with some payment, and the specific payment is identified by the queue name or as some metadata passed along with the event instead of the PaymentID
field in the body of the event being necessary.
The amount of information required to include in an event’s payload matters to all events, the event notification, the event-carried state transfer, and for the changes recorded with event sourcing.
Queues
Queues are referred to by a variety of terms, including bus, channel, stream, topic, and others. The exact term given to a queue will depend on its use, purpose, and sometimes vendor. Because events are frequently—but not always—organized in a first-in, first-out (FIFO) fashion, I will refer to this component as a queue.
Message queues
The defining characteristic of a message queue is its lack of event retention. All events put into a message queue have a limited lifetime. After the events have been consumed or have expired, they are discarded.
You can see an example of a message queue in the following diagram:
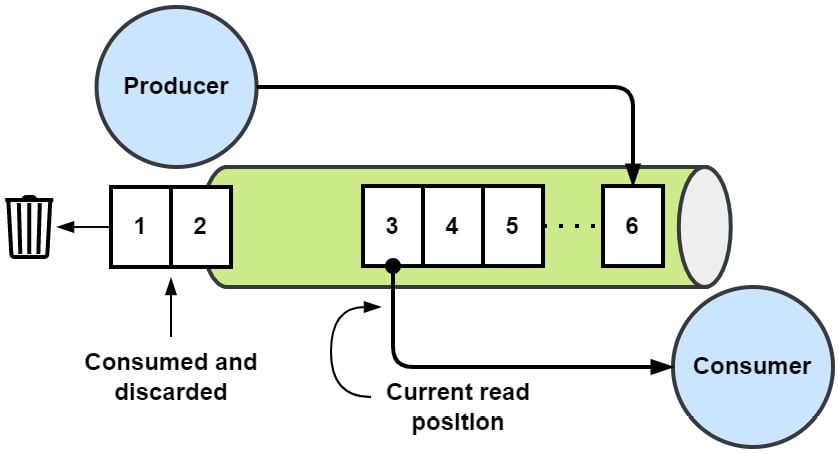
Figure 1.5 – Message queue
A message queue is useful for simple publisher/subscriber (pub/sub) scenarios when the subscribers are actively running or can retrieve the events quickly enough.
Event streams
When you add event retention to a message queue, you get an event stream. This means consumers may now read event streams starting with the earliest event, from a point in the stream representing their last read position, or they can begin consuming new events as they are added. Unlike message queues, which will eventually return to their default empty state, an event stream will continue to grow indefinitely until events are removed by outside forces, such as being configured with a maximum stream length or archived based on their age.
The following diagram provides an example of an event stream:
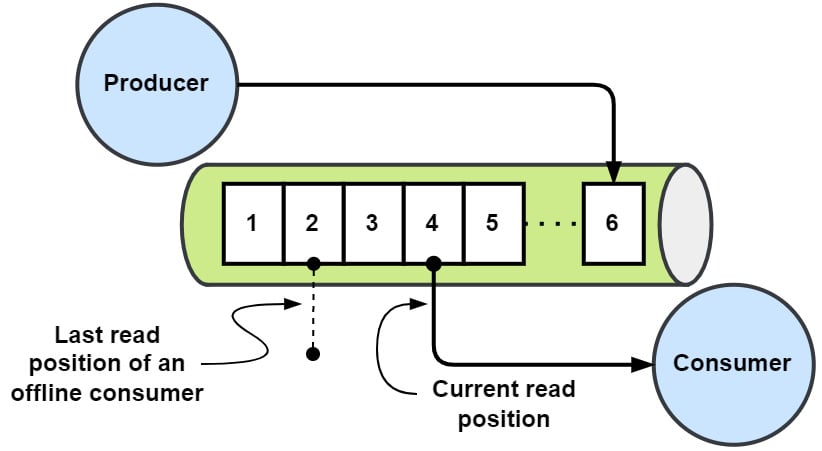
Figure 1.6 – Event stream
When you need retention and the ability to replay events, an event stream should be used instead of a message queue.
Event stores
As the name implies, an event store is an append-only repository for events. Potentially millions of individual event streams will exist within an event store. Event stores provide optimistic concurrency controls to ensure that each event stream maintains strong consistency. In contrast to the last two queue examples, an event store is typically not used for message communication.
You can see an example of an event store in the following screenshot:
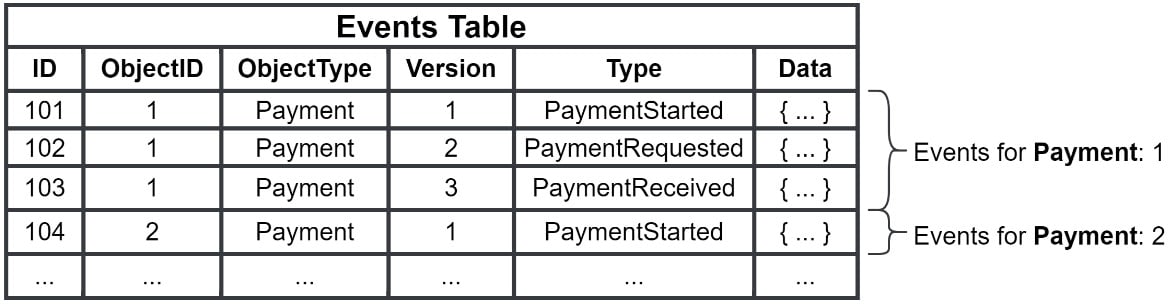
Figure 1.7 – Event store
Event stores are used in conjunction with event sourcing to track changes to entities. The top three rows of Figure 1.7 depict the event-sourcing example events from Figure 1.3.
Producers
When some state in the application has changed, the producer will publish an event representing the change into the appropriate queue. The producer may include additional metadata along with the event that is useful for tracking, performance, or monitoring. The producers of the events will publish it without knowing what the consumers might be listening to. It is essentially a fire-and-forget operation.
Consumers
Consumers subscribe to and read events from queues. Consumers can be organized into groups to share the load or be individuals reading all events as they are published. Consumers reading from streams may choose to read from the beginning of a stream, read new events from the time they started listening, or use a cursor to pick up from where they left the stream.
Wrap-up
Equipped with the types of events we will be using and the knowledge of the components of the patterns involved, let’s now look at how we’ll be using them to build our application.