Inappropriate or non-existent use of authorization
Authorization is the second of two core security concepts that are crucial in implementing and understanding application security. Authorization uses the information that was validated during authentication to determine whether access should be granted to a particular resource. Built around the authorization model for the application, authorization partitions the application functionality and data so that the availability of these items can be controlled by matching the combination of privileges, functionality, and data to users. Our application's failure at this point of the audit indicates that the application's functionality isn't restricted by the user role. Imagine if you were running an e-commerce site and the ability to view, cancel, or modify orders and customer information was available to any user of the site!
Authorization typically involves the following two separate aspects that combine to describe the accessibility of the secured system:
- The first is the mapping of an authenticated principal to one or more authorities (often called roles). For example, a casual user of your website might be viewed as having visitor authority, while a site administrator might be assigned administrative authority.
- The second is the assignment of authority checks to secured resources of the system. This is typically done at the time a system is developed, either through an explicit declaration in code or through configuration parameters. For example, the screen that allows for the viewing of other users' events should be made available only to those users with administrative authority.
Secured resources of a web-based application could be individual web pages, entire portions of the website, or portions of individual pages. Conversely, secured business resources might be method calls on classes or individual business objects.
You might imagine an authority check that would examine the principal, look up its user account, and determine whether the principal is, in fact, an administrator. If this authority check determines that the principal who is attempting to access the secured area is, in fact, an administrator, then the request will succeed. If, however, the principal does not have the sufficient authority, the request should be denied.
Let's take a closer look at an example of a particular secured resource, the All Events page. The All Events page requires administrative access (after all, we don't want regular users viewing other users' events), and as such, looks for a certain level of authority in the principal accessing it.
If we think about how a decision might be made when a site administrator attempts to access the protected resource, we'd imagine that the examination of the actual authority versus the required authority might be expressed concisely in terms of the set theory. We might then choose to represent this decision as a Venn diagram for the administrative user:
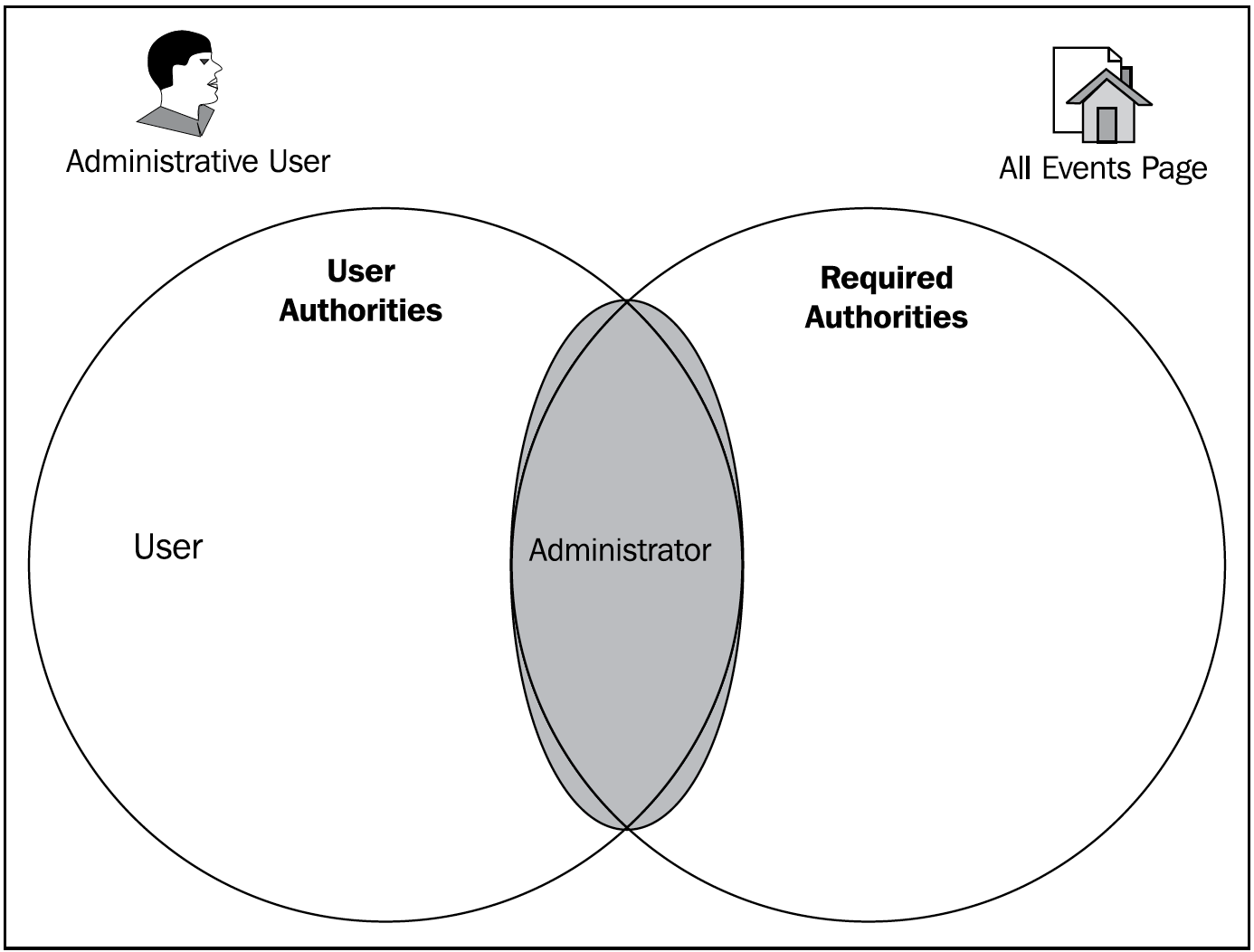
There is an intersection between User Authorities (users and administrators) and Required Authorities (administrators) for the page, so the user is provided with access.
Contrast this with an unauthorized user, as follows:
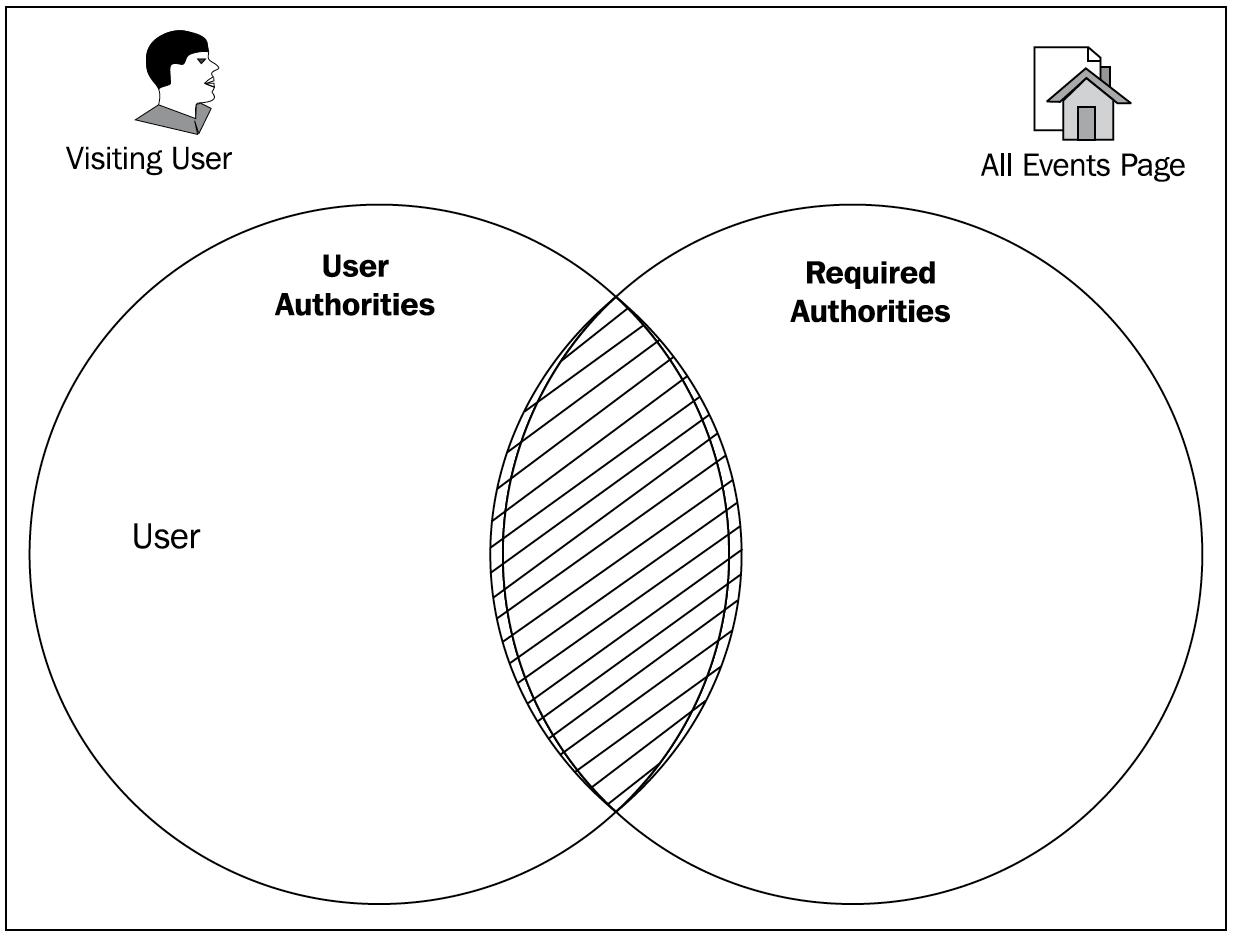
The sets of authorities are disjointed and have no common elements. So, the user is denied access to the page. Thus, we have demonstrated the basic principle of the authorization of access to resources.
In reality, there's real code making this decision, with the consequence that the user is granted or denied access to the requested protected resource. We'll address the basic authorization problem with the authorization infrastructure of Spring Security in Chapter 2, Getting Started with Spring Security, followed by more advanced authorization in Chapter 12, Access Control Lists, and Chapter 13, Custom Authorization.