This book is about Selenium WebDriver, also known as Selenium 2, which is a UI automation tool used by software developers and QA engineers to test their web application on different web browsers. The reader is expected to have a basic idea of programming, preferably using Java, because we take the reader through several features of WebDriver using code examples. This book can be used as a reference for your day-to-day usage of WebDriver.
Chapter 1, Introducing WebDriver and WebElements, will start off by briefly discussing the history of Selenium and the differences between Selenium 1 and Selenium 2. Then, we quickly jump into WebDriver by describing how it perceives a web page. We will also look at what a WebDriver's WebElement is. Then, we talk about locating WebElements on a web page and performing some basic actions on them.
Chapter 2, Exploring Advanced Interactions of WebDriver, will dive deeply into more advanced actions that WebDriver can perform on the WebElements of a web page, such as the dragging-and-dropping of elements from one frame of a page to another and right/context-clicking on WebElements. We're sure you will find this chapter interesting to read.
Chapter 3, Exploring the Features of WebDriver, will talk about some advanced features of WebDriver, such as taking screenshots of web pages, executing JavaScript, and handling cookies and proxies.
Chapter 4, Different Available WebDrivers, will talk about various implementations of WebDriver, such as FirefoxDriver, IEDriver, and ChromeDriver. When we discuss WebDriver in Chapter 1, Introducing WebDriver and WebElements, we will see that WebDriver has specific implementations for most of the popular browsers available on the market.
Chapter 5, Understanding WebDriver Events, will deal with the event-handling aspect of WebDriver. To state a few, events can be a value change on a WebElement, a browser back-navigation invocation, script execution completion, and so on.
Chapter 6, Dealing with I/O, will introduce you to the file-handling features of WebDriver. Concepts such as copying files, uploading files, and deleting files will be discussed in this chapter.
Chapter 7, Exploring RemoteWebDriver and WebDriverBackedSelenium, will deal with two very important topics of WebDriver: RemoteWebDriver and WebDriverBackedSelenium. If you want to execute a WebDriver installed on a different machine from your machine, you can use the RemoteWebDriver class to handle all your commands for that remote machine. One of its popular use cases is browser compatibility testing. The other class we talk about in this chapter is WebDriverBackedSelenium. This is useful for people who want to use WebDriver, but still have many of their existing tests using Selenium 1. Finally, we will migrate some code using Selenium1 APIs to use WebDriver APIs.
Chapter 8 , Understanding Selenium Grid, will talk about one important and interesting feature of Selenium named Selenium Grid. Using this, you can submit your developed automation scenarios to a server and specify there the target platform, that is, the OS, browser type, and version, upon which you want these scenarios to be executed. If a node with such a configuration is registered and available, the server will dispatch your job to that node, and it will take care of executing your automation scenarios in its environment and publish the results back to the server.
Chapter 9, Understanding PageObject Pattern, will talk about a well-known design pattern named the PageObject pattern. This is a proven pattern that will give you a better handle on your automation framework and scenarios.
Chapter 10, Testing iOS and Android Apps, we will take you through how WebDriver can be used to automate your test scripts for iOS and Android applications. We will also discuss a recently developed software tool called Appium.
By the end of this book, we are sure you will be one of the world's advanced WebDriver users.
The following sections describe the installation of components required to work with the code in this book.
In this book, all the code examples that we show covering various features of WebDriver will be in Java. To follow these examples and write your own code, you need Java Development Kit installed on your computer. The latest version of JDK can be downloaded from the following link:
http://docs.oracle.com/javase/7/docs/webnotes/install/windows/jdk-installation-windows.html
A step-by-step installation guide is available at the following link:
http://docs.oracle.com/javase/7/docs/webnotes/install/windows/jdk-installation-windows.html
This book is a practical guide that expects the user to write and execute WebDriver examples. For this, it would be handy to install a Java IDE. You can install your favorite IDE. Here, I am installing Eclipse. It can be downloaded from the following link:
http://www.eclipse.org/downloads/packages/eclipse-ide-java-developers/junosr2
Most of the work in this book will be done using Firefox. However, we do talk about other browsers and their respective drivers in Chapter 4, Different Available WebDrivers. We will work with Firefox 17.0.1, which has been tested and tried against WebDriver 2.33.0. It can be downloaded from the following link:
https://ftp.mozilla.org/pub/mozilla.org/firefox/releases/17.0.1/
Firebug is one of the add-ons of Firefox. It is widely used to inspect HTML elements on a web page. You can get Firebug from the following link:
After installation, when you open the Firefox browser, you should see the firebug icon on the top-right corner of the browser, as shown highlighted in red in the following screenshot:
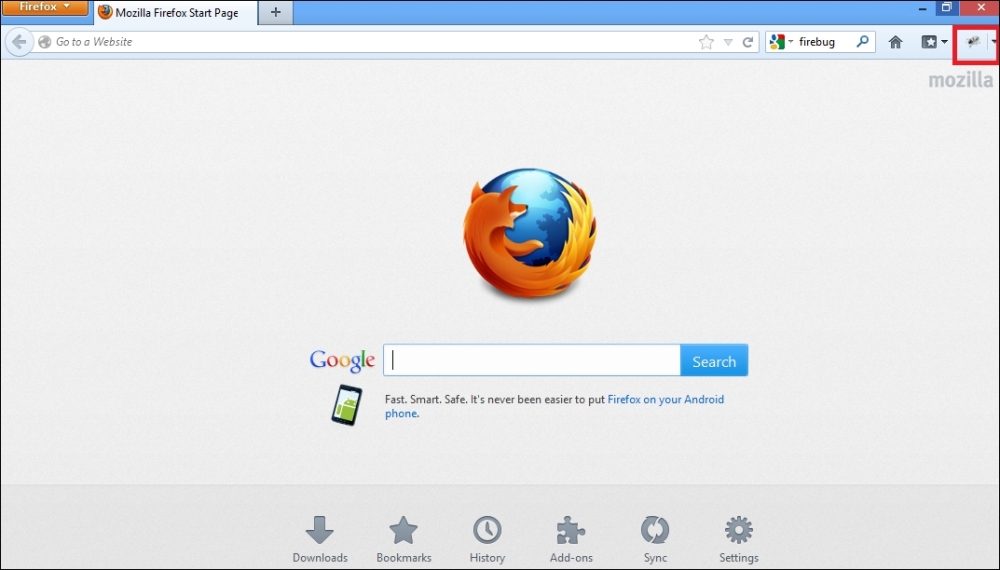
Now, click on the Firebug icon to load the Firebug UI, as shown in the following screenshot:
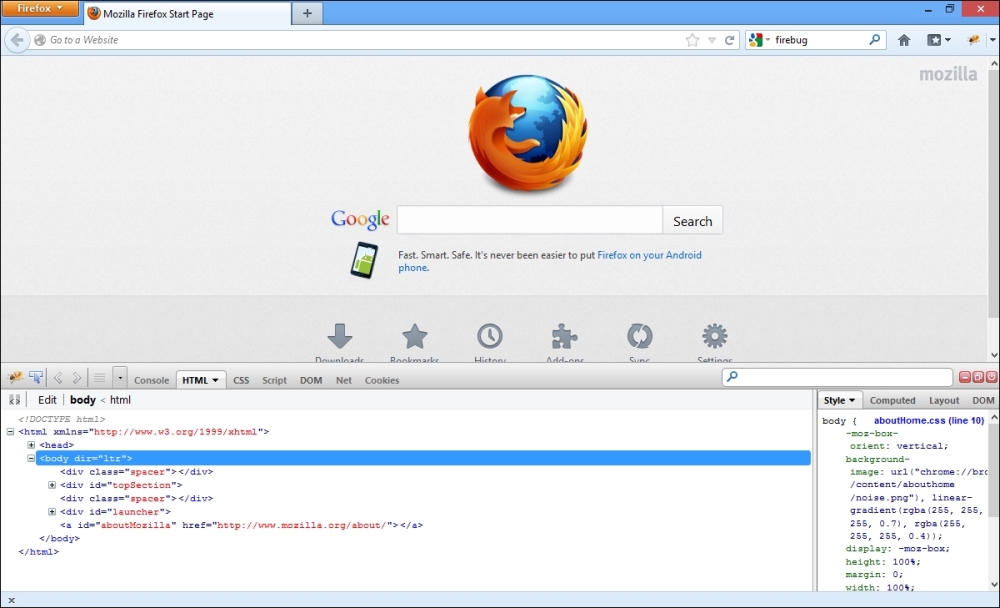
After you have installed the Firebug add-on to Firefox, it's time to extend Firebug to have something named FirePath. FirePath is used to get XPath and CSS values of an HTML element on a web page. You can download FirePath from the following location:
https://addons.mozilla.org/en-US/firefox/addon/FirePath/
After installation, you should see a new tab in the Firebug UI for FirePath, as shown in the following screenshot:
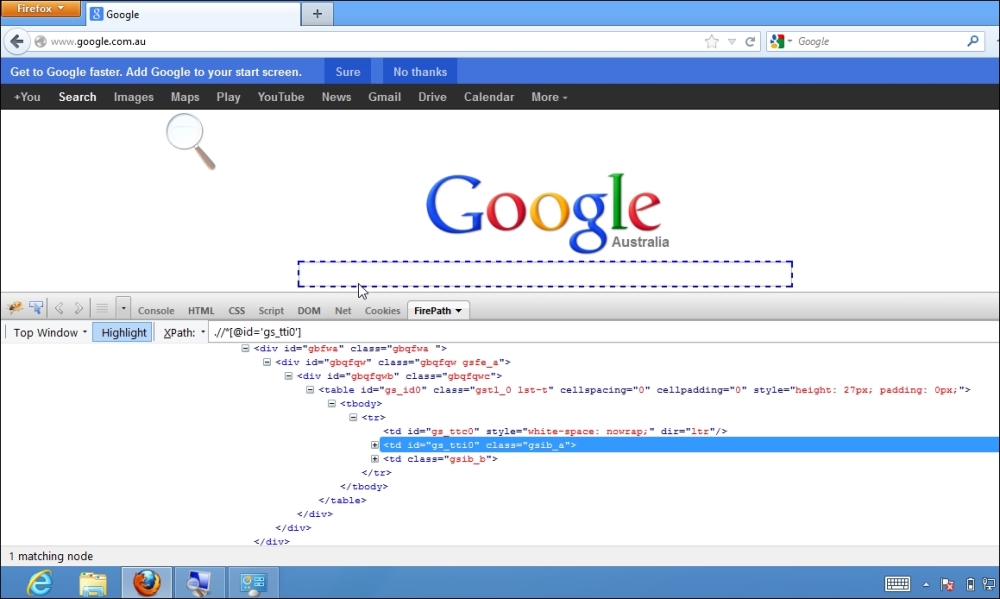
As discussed earlier, test scripts need a client library with which to interact, or command WebDriver to execute specific user events against a web application being tested on a browser. For this, you need to download the WebDriver client library. In this book, we use Java language bindings to create and execute our automation scripts.
At the time of writing this book, all the code examples are written based on Selenium Java Version 2.33.0. It is recommended that you download that version from the following location:
https://code.google.com/p/selenium/downloads/detail?name=selenium-java-2.33.0.zip&can=2&q=
The good news is that you have already downloaded the Firefox Driver. Yes, the Firefox Driver comes along with client libraries. But, for other drivers, such as the IE Driver, Safari Driver, Chrome Driver, and so on, you have to download them explicitly from the following link:
http://docs.seleniumhq.org/download/
We will download them when we need to in Chapter 4, Different Available WebDrivers.
If you are a quality assurance/testing professional, software developer, or web application developer looking to create automation test scripts for your web applications, this is the perfect guide for you! As a prerequisite, this book expects you to have a basic understanding of Java programming, although any previous knowledge of WebDriver or Selenium 1 is not needed. By the end of this book, you will have acquired a comprehensive knowledge of WebDriver, which will help you in writing your automation tests.
In this book, you will find a number of styles of text that distinguish among different kinds of information. Here are some examples of these styles, and an explanation of their meaning.
Code words in text, database table names, folder names, filenames, file extensions, pathnames, dummy URLs, user input, and Twitter handles are shown as follows: "The moveByOffset()
method is used to move the mouse from its current position to another point on the web page."
A block of code is set as follows:
public class NavigateToAUrl { public static void main(String[] args){ WebDriver driver = new FirefoxDriver(); driver.get("http://www.google.com"); } }
When we wish to draw your attention to a particular part of a code block, the relevant lines or items are set in bold:
public class GoogleSearchButtonByName {
public static void main(String[] args){
WebDriver driver = new FirefoxDriver();
driver.get("http://www.google.com");
WebElement searchBox = driver.findElement(By.name("btnK"));
searchBox.submit();
}
}
Any command-line input or output is written as follows:
java -jar selenium-server-standalone-2.33.0.jar -role node -hub http://172.16.87.131:1111/grid/register -registerCycle 10000
New terms and important words are shown in bold. Words that you see on the screen, in menus or dialog boxes for example, appear in the text like this: "Open Eclipse from the directory you have installed it in earlier. Navigate to File | New | Java Project".
Feedback from our readers is always welcome. Let us know what you think about this book—what you liked or may have disliked. Reader feedback is important for us to develop titles that you really get the most out of.
To send us general feedback, simply send an e-mail to <[email protected]>
, and mention the book title via the subject of your message.
If there is a topic that you have expertise in and you are interested in either writing or contributing to a book, see our author guide on www.packtpub.com/authors.
Now that you are the proud owner of a Packt book, we have a number of things to help you to get the most from your purchase.
You can download the example code files for all Packt books you have purchased from your account at http://www.packtpub.com. If you purchased this book elsewhere, you can visit http://www.packtpub.com/support and register to have the files e-mailed directly to you.
Although we have taken every care to ensure the accuracy of our content, mistakes do happen. If you find a mistake in one of our books—maybe a mistake in the text or the code—we would be grateful if you would report this to us. By doing so, you can save other readers from frustration and help us improve subsequent versions of this book. If you find any errata, please report them by visiting http://www.packtpub.com/submit-errata, selecting your book, clicking on the errata submission form link, and entering the details of your errata. Once your errata are verified, your submission will be accepted and the errata will be uploaded on our website, or added to any list of existing errata, under the Errata section of that title. Any existing errata can be viewed by selecting your title from http://www.packtpub.com/support.
Piracy of copyright material on the Internet is an ongoing problem across all media. At Packt, we take the protection of our copyright and licenses very seriously. If you come across any illegal copies of our works, in any form, on the Internet, please provide us with the location address or website name immediately so that we can pursue a remedy.
Please contact us at <[email protected]>
with a link to the suspected pirated material.
We appreciate your help in protecting our authors, and our ability to bring you valuable content.
You can contact us at <[email protected]>
if you are having a problem with any aspect of the book, and we will do our best to address it.