Grunt is a simple-to-use task runner written with Node.js, a scalable JavaScript software platform. A task runner is defined as a software tool used to automate predefined tasks to ease the development and integration of large-scale projects that are in production.
The following is a look into the official website for Grunt:
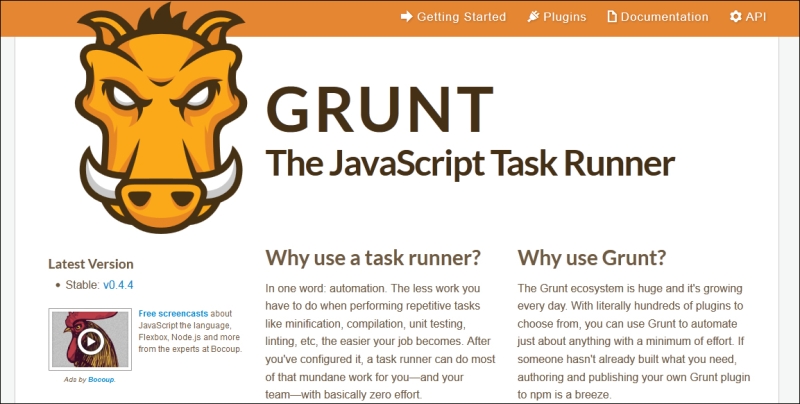
Source: http://gruntjs.com/
Grunt is currently used by large organizations such as Twitter and Adobe, and by technologies such as jQuery. Through consistent updates, it has recently become one of the most stable automation tools since its creation. The open source community has embraced its simplicity compared to alternatives such as Ant or Maven. Lastly, it is praised for its use of the ubiquitous language, JavaScript, thereby easing the training among development teams. In this book, Grunt will be used to automate the compilation of Sass to CSS and CoffeeScript to JavaScript. Minification will be used to optimize page load times. Obfuscation, the mangling of information, will also be automated to deter code theft and manipulation from the client side. Grunt will be used to automate testing via the Mocha engine.
So, why should you use Grunt? In today's world of modern web applications, you may find yourself using various tools that require compilation or preprocessing. Likewise, you may wish to obfuscate code and minimize the size of your public files in order to optimize the load time of our website. At other times, you could manually call executables to perform these tasks. This would only waste more time that would otherwise have been spent in the development of your app. With a simple, customizable configuration file, Grunt allows you to set up a build script to automate these activities via its community-curated plugins.
Plugins are installed via npm, which will be explained later in this chapter. Certain plugins are deemed contrib packages (these are labeled with a contrib indicator such as grunt-contrib-jshint
) and are branded as officially maintained and stable. These will be used throughout the book to ensure consistency.
Every Gruntfile will be typically aligned with the following format:
// constants and functions module.exports = function (grunt) { grunt.initConfig({ // configuration }); // user-defined tasks }
Note
It is important to note that Grunt follows the CommonJS spec, a project developed to normalize JavaScript styles and conventions. As such, Grunt exports itself as a module that contains its configuration and tasks.
In order to ease the configuration process, Grunt users should ideally store any ports, functions, and other constants, which are used at the top of the file, as global variables. This ensures that if a constant or function suddenly changes, editing the global variable at the top would be a lot simpler than changing its value at every location in the file.
Also, constants help to provide information by allocating a variable name to each unknown value. For instance, look at the following command line:
var LIVERELOAD_PORT = 35729;
In this case, any instance of the port within the configuration can easily be traced back to the LiveReload plugin.
The configuration section contains the settings required for each Grunt plugin that is included. As a result, these will be plugin-specific and will be documented by the maintainers of each plugin respectively.
For instance, the grunt-contrib-uglify
plugin is used to minify various files. By default, the plugin will also obfuscate the existing code. Users can optionally turn the mangle
option off in the configuration section to prevent this from happening.
Lastly, the Gruntfile will end with a list of user-defined tasks. By default, every Grunt plugin has a respective task that may be called to achieve its desired output. User-defined tasks may be defined at the end of the Gruntfile to synchronously chain multiple Grunt plugin tasks together.
For instance, you may wish to define a task for sysadmins that will deploy a production-ready version of your web application by combining minification, concatenation, and compilation tasks. You may also wish to define a watch task for web developers to auto-compile CoffeeScript or Sass files that require compilation to be used. For a team of interns, a task that combines validation plugins for various coding languages may be used to prevent errors and aid their learning process.