Introduction
Application Programming Interface (API) is not a new buzz word in the programming world. If we take a history tour of all programming languages ever developed, we will notice that any language that allowed software components to communicate and exchange information supports the notion of an API. An API can be as simple as defining a function in the procedural language such as C, or can be as complex as defining a protocol standard; while the structure and complexity of an API may be varied, the intent of an API mostly remains the same. Simply put, an API is a composition of a set of behaviors that perform some specific and deterministic tasks. Clients can then consume this API placing requests on any of the behaviors and expect appropriately formatted responses.
For example, consider the following code snippet that leverages the System.IO.File
type in the .NET framework to read from a text file and print its contents in a console window:
static void Main(string[] args) { string text = System.IO.File.ReadAllText(@"C:\MySample.txt"); System.Console.WriteLine("Contents of MySample.txt = {0}", text); }
In the preceding example, the System.IO.File
type exposes a set of specific behaviors that a client can consume. The client invokes a request by providing the required input and gets back an expected response. The System.IO.File
type acts like a third-party system that takes input and provides the desired response. Primarily, it relieves the client from writing the same logic and also relieves the client from worrying about the management of the System.IO.File
source code. On the other hand, the developers of System.IO.File
can protect their source from manipulations or access; well, not in this case because the .NET Framework source code is available under Microsoft Reference Source License. The bits for the .NET Framework source can be accessed at https://github.com/Microsoft/dotnet.
If we now take the preceding API definition and stitch it with a Web standard-like HTTP, we can say that:
A Web API is a composition of a set of behaviors that perform concrete and deterministic tasks in a stateless and distributed environment.
If this feels like a philosophical statement, we will look at a more technical definition when we delve in the ASP.NET Web API in the coming sections.
Note
Note that the semantics of a Web API do not necessarily require it to leverage HTTP as a protocol. However, since HTTP is the most widely used protocol for Web communication and unless some brilliant mind is working on a garage project to come up with an alternative, it is safe to assume that Web APIs are based on HTTP standards. In fact, Web APIs are also referred as HTTP Services.
Before we get into the details of writing our own Web APIs, let's try consuming one; we will use the Bing Map API for our example:
Bing Maps provide a simple trial version of their Map Web API (http://msdn.microsoft.com/en-us/library/ff701713.aspx) that can be used for scenarios such as getting real-time traffic incident data, location, routes, and elevations. To access the API, we need a key that can be obtained by registering at the Bing Maps Portal (https://www.bingmapsportal.com/). We can then access information such as location details based on geo coordinates, address, and other parameters.
In the following example, we fetch the address location for the Microsoft headquarters in Redmond:

The response should look as follows:
{ "authenticationResultCode":"ValidCredentials","brandLogoUri":"http:\/\/dev.virtualearth.net\/Branding\/logo_powered_by.png","copyright":"Copyright © 2015 Microsoft and its suppliers. All rights reserved. This API cannot be accessed and the content and any results may not be used, reproduced or transmitted in any manner without express written permission from Microsoft Corporation.","resourceSets":[{"estimatedTotal":1,"resources":[{"__type":"Location:http:\/\/schemas.microsoft.com\/search\/local\/ws\/rest\/v1","bbox":[47.636677282429325,-122.13698331308882,47.644402717570678,-122.12169668691118],"name":"Microsoft Way, Redmond, WA 98052","point":{"type":"Point","coordinates":[47.64054,-122.12934]},"address":{"addressLine":"Microsoft Way","adminDistrict":"WA","adminDistrict2":"King Co.","countryRegion":"United States","formattedAddress":"Microsoft Way, Redmond, WA 98052","locality":"Redmond","postalCode":"98052"},"confidence":"Medium","entityType":"Address","geocodePoints":[{"type":"Point","coordinates":[47.64054,-122.12934],"calculationMethod":"Interpolation","usageTypes":["Display","Route"]}],"matchCodes":["Good"]}]}],"statusCode":200,"statusDescription":"OK","traceId":"a54a3e7f071b44e498af17b2b1dc596d|CH10020545|02.00.152.3000|CH1SCH050110320, CH1SCH060052346" }
Note
To run the preceding example, we use a Chrome browser extension called Postman (https://chrome.google.com/webstore/detail/postman-rest-client/fdmmgilgnpjigdojojpjoooidkmcomcm?hl=en). It provides a good GUI interface to allow executing HTTP Services from within the browser without writing any code. We will use Postman for examples in this book. However, other tools such as Fiddler (http://www.telerik.com/fiddler) can also be used.
We notice a few things here:
We did not write a single line of code; in fact, we did not even open an IDE to make to call to the Web API. Yes, it is that easy!
The Bing API presented the caller with a mechanism to uniquely access a resource through a URI and parameters. The client requested the resource and the API processed the request to produce a well-defined response.
The example is simple but it is still accessed in a secure manner, the Bing API mandates that an API key be passed with the request and throws an unauthorized failure code if the key cannot validate the authenticity of the caller.
We talk in greater detail about how this request was processed earlier. However, there are two key technologies that enabled the execution of the preceding request, namely, HTTP and REST. We discuss these in greater details in this section.
To explore more free and premium API(s) you can take a look at http://www.programmableweb.com/. Programmable web is one of the largest directories of HTTP-based APIs and provides a comfortable and convenient way to discover and search APIs for Web and mobile application consumption.
Getting to know HTTP
Since we concluded in the previous section that Web APIs are based on HTTP, it is important to understand some of the fundamental constructs of HTTP before we delve into other details.
THE RFC 26163 specification published in June 1999 defines the Hypertext Transfer Protocol (HTTP) Version 1.1. The specification categorizes HTTP as a generic, stateless, application-level protocol for distributed, collaborative, and hypermedia information systems. The role of HTTP, for years, has been to access and handle especially when serving the Web documents over HTML. However, the specification allows the protocol to be used for building powerful APIs that can provide business and data services at hyperscale.
Note
A recent update to the HTTP 1.1 specification has divided the RFC 2616 specification into multiple RFCs. The list is available at http://evertpot.com/http-11-updated/.
An HTTP request/response
HTTP relies on a client-server exchange and defines a structure and definition for each request and response through a set of protocol parameters, message formats, and protocol method definitions.
A typical HTTP request/response interaction looks as follows:
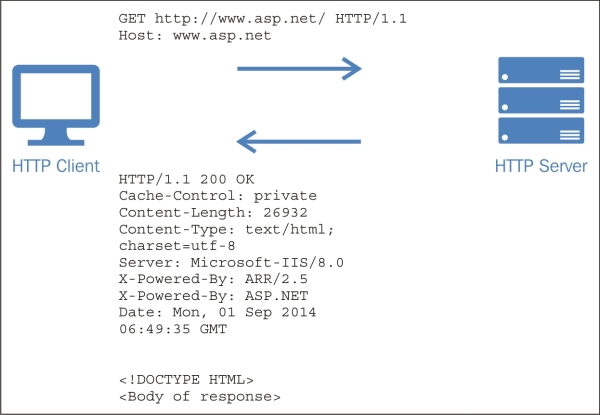
The client made a request to fetch the contents of a resource located at www.asp.net, specifying to use HTTP Version 1.1 as the protocol. The server accepts the request and determines more information about the request from its headers, such as the type of request, media formatting, and resource identifier. The server then uses the input to process the results appropriately and returns a response. The response is accompanied by the content and a status code to denote completion of the request. The interaction between the client and server might involve intermediaries such as a proxy or gateway for message translation. However, the message request and response structures remain the same.
Note
HTTP is a stateless protocol. Hence, if we attempt to make a request for the resource at the same address n times, the server will receive n unique requests and process them separately each time.
HTTP methods
In the preceding request, the first thing a server needs to determine is the type of request so that it can validate if the resource supports this request and can then serve it. The HTTP protocol provides a set of tokens called methods that indicate the operations performed on the resource.
Further, the protocol also attempts to designate these methods as safe and idempotent; the idea here is to make the request execution more predictable and standardized:
A method is safe if the method execution does not result in an action that modifies the underlying resource; examples of such methods are
GET
andHEAD
. On the other hand, actions such asPUT
,POST
, andDELETE
are considered unsafe since they can modify the underlying resource.A method is idempotent if the side effects of any number of identical requests is the same as for a single request. For example
GET
,PUT
, andDELETE
share this property.
The following table summarizes the standard method definitions supported by HTTP Version 1.1 protocol specification:
Method |
Description |
Safe |
Idempotent |
---|---|---|---|
|
This represents the communication options for the target resource. |
Yes |
Yes |
|
This requests data from a specified resource. |
Yes |
Yes |
|
This is the same as |
Yes |
Yes |
|
This requests to send data to the server, typically evaluates a create request. |
No |
No |
|
This requests to replace all current representation of the target resource, typically, generally evaluates an |
No |
Yes |
|
This applies a partial update to an object in an incremental way. |
Yes |
No |
|
This requests to remove all current representation of the target resource. |
No |
Yes |
|
This performs a message loop-back test (echo) along the path to the targeted resource. |
Yes |
Yes |
|
This establishes a tunnel to the server identified by a given URI. This, for example, may allow the client to use the Web server as a proxy. |
Yes |
Yes |
HTTP status codes
HTTP status codes are unique codes that a server returns based on how the request is processed. Status codes play a fundamental role in determining response success and failure especially when dealing with Web APIs. For example, 200 OK
means a generic success whereas 500
indicates an internal server error.
Status codes play a pivotal role while developing and debugging the Web API. It is important to understand the meaning of each status code and then define our responses appropriately. A list of all HTTP status codes is available at http://en.wikipedia.org/wiki/List_of_HTTP_status_codes.
Other HTTP goodies
From a Web API perspective, there are some other aspects of HTTP that we should consider.
Header field definitions
Header fields in HTTP allow the client and server to transfer metadata information to understand the request and response better; some key header definitions include:
Field |
Description |
---|---|
|
This specifies certain attributes that are acceptable for a response. These headers can be used to provide specifications to the server on what type of response is expected, for example, |
|
The header indicates a list of all valid methods supported by a requested resource ( |
|
This is the authorization header for the request. |
|
The header acts as a modifier to a content type. It indicates the additional encodings that are applied to the body, for example, Gzip, deflate. |
|
This indicates a cache directive that is followed by all caching mechanisms throughout the request/response chain. The header plays an important role when dealing with server-side cache and CDN. |
|
This indicates the media type of the entity-body sent to the server. |
|
The header allows clients or firewalls that don't support HTTP methods such as |
Content negotiation
Content negotiation is a technique to identify the "best available" response for a request when multiple responses may be found on the server. HTTP 1.1 supports two types of negotiations:
Pre-emptive or server-driven negotiation: In this case, the server negotiates with the client (based on the
Accept
headers) to determine the type of response.Reactive or client-driven negotiation: The server presents the client with the representations available and lets the client choose based on their purpose and goals.
More information about content negotiation can be found at http://www.w3.org/Protocols/rfc2616/rfc2616-sec12.html.
HTTP 2.0
HTTP 2.0 is the next planned version of the HTTP protocol specification and is an attempt to optimize the usage of network resources and reduce latency through header compressions and pooling multiple connections over the same channels. The initiative is spearheaded by Google and Mozilla research teams and as of today, is in a working draft state. The good news is that HTTP 2.0 is going to retain all the goodness of HTTP 1.1 while making it more performant over the wire through efficient processing of messages. Since HTTP 2.0 is still in the making, we will refrain from using it for the scope of this book. However, if you are enthusiastic to know more about HTTP 2.0, you can take a look at the current draft of the specification at http://http2.github.io/http2-spec/.
For the scope of this book, HTTP always refers to HTTP 1.1 specification of the protocol.
HTTP and .NET
Starting from .NET 4.5, a new namespace and assembly was added as part of the framework, System.Net.Http
. The resemblance of the types in this namespace may look behaviorally similar to those defined in ASP.NET System.Web.Http
namespace; however, the addition of this namespace marks a big difference in the development of HTTP services.
Firstly, it enables unified communication over HTTP by providing a set of abstract types. These types allow any .NET application to access HTTP services in a consistent way, for example, now both the client and server can use the same HTTP programming model for better development experience. Secondly, it has been written to target modern HTTP apps such as Web API and mobile development, this signifies the investment of Microsoft in making HTTP services a first-class citizen.
Some of the key types defined in this namespace are:
Type |
Description |
---|---|
|
This enables primitive operations for sending HTTP requests and receiving HTTP responses from a resource identified by a URI. |
|
This represents the HTTP request message from a client. |
|
This represents the HTTP response message received from an HTTP request. |
|
This is a base class that represents an HTTP entity body and any content headers. |
|
This is the base type for all message handlers and this will be used to define all message handlers. Message handlers may be used to create server-side or client-side handlers. The server-side handler works with the hosted model chain and handles incoming client requests ( The We may, of course, hook our custom controllers in the pipelines, for example, to validate, modify, or log the requests. |
Note
For a complete list of all types available in the System.Net.Http
namespace, please visit http://msdn.microsoft.com/en-us/library/system.net.http(v=vs.110).aspx.
We will see in the later sections that the ASP.NET Web API also leverages some of the System.Net.Http
types for communication over HTTP.
The rise of REST
When talking about Web APIs, it is imperative to mention the REST framework. Over the years, REST has proven to be a much simplified and efficient web service architecture style as compared to other architectures like RPC-based SOAP. The key reason for this popularity is because of its modern web design patterns and utilization of HTTP transport layer features to the fullest. REST has enabled many modern-world scenarios such as Mobile apps and Internet of Things (IoT), which would be challenging with protocols like SOAP because of its rigid schema-based WSDL structure and bulky XML standards.
Note
A good comparison of REST and SOAP protocols can be found at http://blog.smartbear.com/apis/understanding-soap-and-rest-basics/.
The REST style of services
REST stands for Representational State Transfer and perhaps the most important thing to realize about REST is that it is an architecture style and not a standard (like SOAP). What do we mean by this?
An architectural style is an accumulation of a set of design elements and constraints that can be tailored to define communication and interaction for a system. The elements in an architecture style are abstracted to ignore the implementation and protocol syntax and focus more on the role of the component. Additionally, constraints are applied to define the communication and extensibility patterns for these elements.
The focus of the REST architectural style is to improve the performance, portability, simplicity, reliability, and scalability of the system. The REST architecture style assumes the system as a whole in its initial state. It then evolves the system by incrementally applying constraints; these constraints allow components to scale efficiently while still maintaining a uniform set of interfaces. It also reduces deployment and maintenance considerations by layering out these components. Note that REST itself is a composition of many other architecture styles. For example, REST utilizes a set of other network architecture styles such data styles, hierarchical styles, and mobile code styles system to define its core architectural constraints. For more information on network architecture styles, please visit http://www.ics.uci.edu/~fielding/pubs/dissertation/net_arch_styles.htm.
For a Web API to be RESTful, most or all of the following architectural constraints must be satisfied:
Constraint |
Description |
---|---|
Client-server |
This constraint enforces a separation of concern between the client and server components. The core of this constraint is to promote a distributed architecture where the client can issue requests to a server, and the server responds back with status and the actual response. It enables multiple clients to interact with the server and allows the server to evolve independently. |
Stateless |
The stateless constraint evolves from the client server but mandates that no session state be allowed on the server component. The client must send all information required to understand the request and should not assume any available state on the server. This pattern is the backbone for enabling scalable Web APIs in a cloud-based environment. |
Cache |
Cache constraint is applied on top of client-server and stateless constraints and allows the requests to be implicitly or explicitly categorized as cacheable and noncacheable. The idea is to introduce a cache intermediator component that can improve latency by caching responses and minimizing interactions over the network. The cache can be employed as a consumer cache or a service side cache. |
Uniform interface |
The uniform interface constraint is the most critical constraint of a REST architecture style and distinguishes REST from other network architecture styles. The constraint emphasizes a uniform interface or contract between components. REST provides the following set of interface constraints: identification of resources, manipulation of resources through representation, self-descriptive messages, and hypermedia as the engine of application state. A typical uniform interface may be a combination of HTTP methods, media types (JSON, XML), and the resource URI. These provide a consistent interface for consumers to perform the desired operation on the resource. |
Layered system |
A layered system enables the architecture to be composed of hierarchical layers. These layers expose components to achieve specific behavior or functionality and these components only interact with components within their layer. Having a layered approach promotes extensibility and loose coupling between components. |
Code on demand |
The code on demand constraint is an optional constraint, and the main idea is to allow clients to be independently updated based on the browser add-on or client scripts. |
For a more detailed understanding of REST-based architecture, the one source of truth is Roy Thomas Fielding's dissertation, Architectural Styles and the Design of Network-based Software Architectures (http://www.ics.uci.edu/~fielding/pubs/dissertation/top.htm).
Web API and Microsoft Azure
Microsoft Azure is a scalable cloud platform that enables organizations to rapidly build, deploy, and manage their applications in a Platform as a Service (PaaS) or Infrastructure as a Service (IaaS) model. It also provides an array of Software as a Service (SaaS) offerings built on Microsoft Azure that further improve the productivity of an organization. Microsoft Azure treats Web APIs as a first-class citizen and all the services and features exposed by Microsoft Azure expose REST-based Web APIs that can be consumed by clients to manage their hosted application and underlying environments.
Some of these APIs are listed here:
Service |
API |
---|---|
Service Management API |
This is the API for managing Azure subscriptions and environment programmatically. For more information, visit https://msdn.microsoft.com/en-us/library/azure/ee460799.aspx. |
Azure AD |
This is the graph API for accessing and managing an Azure AD tenant. For more information, visit https://msdn.microsoft.com/en-us/library/azure/hh974478.aspx. |
Service Bus |
This is the API for managing and accessing Service Bus entities, such as Topics, Queues, and EventHub. For more information, visit https://msdn.microsoft.com/en-us/library/azure/hh780717.aspx. |
Notification Hubs |
This is the API for managing Notification Hubs. For more information, visit https://msdn.microsoft.com/en-us/library/azure/dn223264.aspx. |
BizTalk Services |
This is the API for performing management operations on BizTalk Services. For more information, visit https://msdn.microsoft.com/en-us/library/azure/dn232347.aspx. |
Azure SQL Database |
This is the API for managing SQL databases, server configuration, and firewall associations for the servers. For more information, visit https://msdn.microsoft.com/en-us/library/azure/gg715283.aspx. |
Mobile Services |
This is the API for managing authentication and underlying database CRUD operations. For more information, visit https://msdn.microsoft.com/en-us/library/azure/jj710108.aspx. |
Storage Services |
This is the API for managing storage entities, such as tables, blobs, and file services. For more information, visit https://msdn.microsoft.com/en-us/library/azure/dd179355.aspx. |
API Management |
This is the API for managing the API Management service provided by Microsoft Azure. This allows for management of users, tenants, certificates, authorization servers, products, and reporting capabilities. For more information, visit https://msdn.microsoft.com/en-us/library/azure/dn776326.aspx. |
Azure Web Sites |
This has a REST API but is not currently exposed publicly. |
Note
For a list of all Microsoft Azure Web APIs, please refer to Microsoft Azure documentation at https://msdn.microsoft.com/en.
Apart from providing a comprehensive set of REST-based Web APIs, Microsoft Azure provides the tools and environment for customers to build and host their own Web APIs. Chapter 1, Getting Started with the ASP.NET Web API to Chapter 4, Developing a Web API for Mobile Apps discuss these tools and techniques in detail.
Summary
The Web API market has seen exponential growth since its inception in 2005. Over the last couple of years, the published APIs have increased to more than 12,000 and the number has been increasing each month. Web APIs have given organizations new opportunities to collaborate with partners in a secure and easy way and, at the same time, allowed for rapid development of consumer-based mobile and web-based applications. HTTP and REST provide the backbone for the Web API development, making it widely available and easy to consume. Microsoft Azure has taken special measures to expose services and provide support to easily incorporate the REST-based programming model into customer environments.
In the next chapters, we will dive into the details of the tools and techniques provided by Microsoft Azure and the ASP.NET Web API framework to rapidly build, deploy, and manage scalable Web API services.