ASP.NET Core is a complete rewrite of the ASP.NET framework, rather than a new version built on top of the existing one. It is much smaller and more modular, and is the first version of ASP.NET to have official cross-platform support. Covering everything that has changed in ASP.NET Core would require a whole book in itself, so we'll focus on the main parts that we need to know a little bit about to get us started.
ASP.NET Core – what's new?
Middleware pipeline
ASP.NET Core apps are built on the principle of a middleware pipeline. But what exactly do we mean by this? Web applications are all about HTTP requests and responses; a middleware pipeline is simply a set of instructions for how the application should handle each HTTP request.
Ultimately, an ASP.NET Core middleware is just a function which is invoked as a result of a HTTP request. If we need multiple middleware functions, they can be chained together in a specific order to form a pipeline to process the request. After each function finishes processing, they may decide that the request should be terminated. In this instance, it sends a response back to the previous middleware, which in turn passes it back to the middleware before that, and so on until it eventually ends up back with the client that started the request. Alternatively, if everything is successful during processing, it can simply pass the request on to the next piece of middleware defined in the chain. Each middleware can essentially perform some kind of custom logic before the request, after the request, or both. As an example, you could easily create a request timing middleware that records a timestamp before and after every request, so that the total processing time of each HTTP request can be logged somewhere.
The following diagram shows how this works in an ASP.NET Core web application:
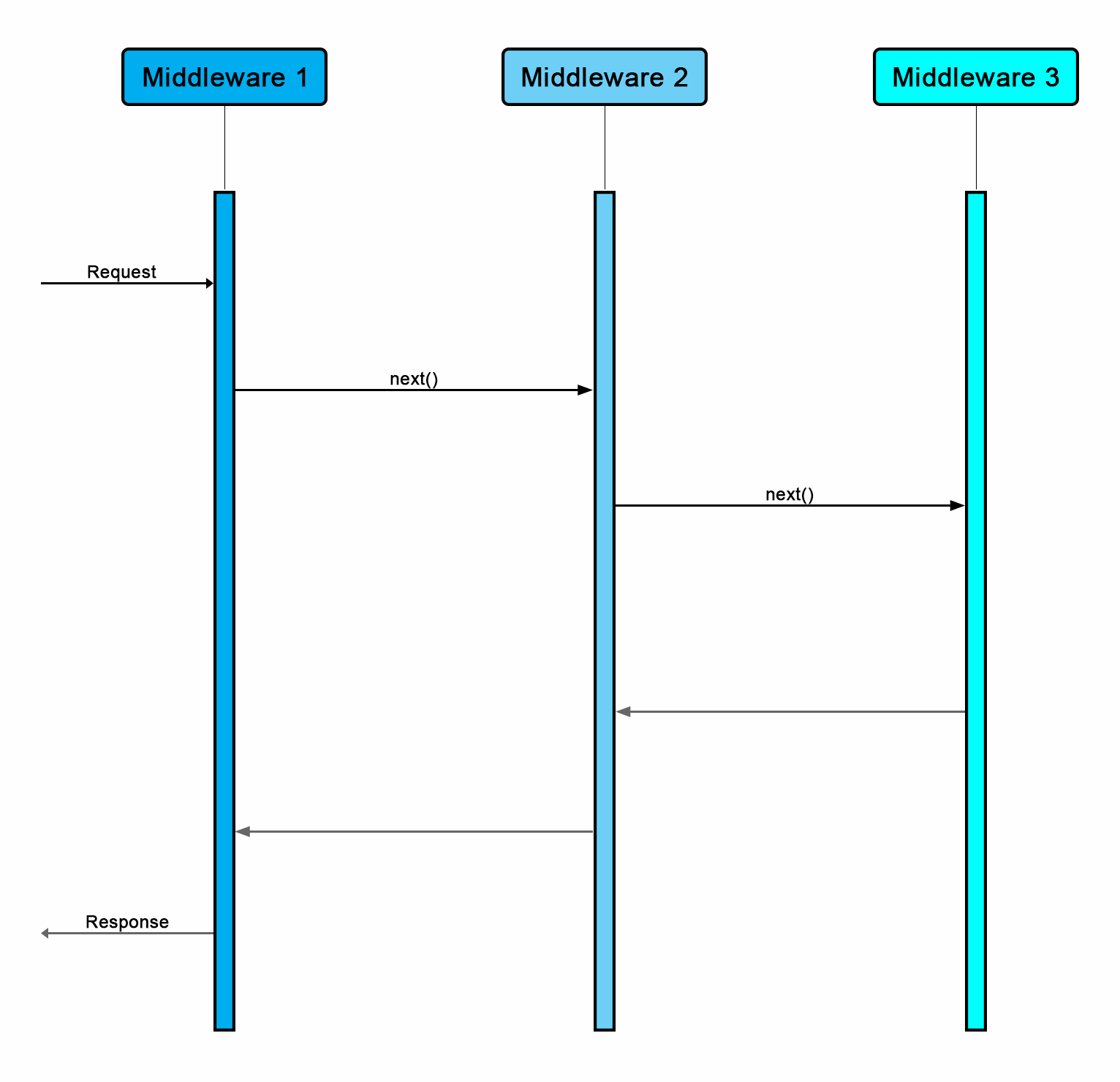
ASP.NET Core provides a number of middleware components for us to optionally make use of, and we'll see how we go about registering these with our application in the next section. The only other thing to note is that the order that we register our middleware in is extremely important. If one middleware depends on another one having processed the request before it receives it, we need to make sure that the dependent middleware is configured after the middleware it depends upon. Otherwise, our requests are likely to fail, or at least display some very strange results depending on what the middleware are designed to do.
Later in this book, we'll look at how we can build our own custom middleware and register them with the pipeline.
Application startup
All ASP.NET Core applications must have a Startup.cs file that contains a class named Startup. There are two methods we need to be aware of, and these are Configure and ConfigureServices.
Configure is a required method that is used to, as the name suggests, configure the application, specifically by setting up the request pipeline, including any optional middleware that we may need. The following sample shows how we would configure a simple ASP.NET application that uses the StaticFiles, Authentication, RequestLocalization, and MVC middleware:
public void Configure(IApplicationBuilder app, IHostingEnvironment env)
{
app.UseStaticFiles();
app.UseAuthentication();
app.UseRequestLocalization();
app.UseMvc(routes =>
{
routes.MapRoute(
name: "default",
template: "{controller=Home}/{action=Index}/{id?}");
});
}
ConfigureServices is optional, although I've yet to find an application that doesn't implement it. It is used to configure the application's services and register them with the built-in dependency injection (DI) container. Most non-trivial applications will need to configure services such as Entity Framework (EF), ASP.NET Identity, RequestLocalization, and MVC. The following code shows a sample configuration of these services:
public void ConfigureServices(IServiceCollection services)
{
services.AddDbContext<ApplicationDbContext>(options =>
options.UseNpgsql(Configuration["ConnectionString"]));
services.AddIdentity<ApplicationUser, IdentityRole>()
.AddEntityFrameworkStores<ApplicationDbContext>()
.AddDefaultTokenProviders();
services.Configure<RequestLocalizationOptions>(options =>
{
options.DefaultRequestCulture = new RequestCulture("en-GB");
});
services.AddMvc();
}
You'll probably spend a fair bit of time in this class, tweaking configurations and such, so let's look into these two sections in a little more detail.
DI is a first-class citizen
ASP.NET Core has been built from the ground up with DI in mind, and in fact DI is now a pattern that is strongly encouraged for use in every application. As such, it is no longer required to use an external library for DI, as there is a simple built-in container included out of the box. On top of this, the vast majority of official supporting libraries are registered as DI services when we install them, such as EF and ASP.NET Identity. That being said, the built-in container is fairly limited in functionality, and for more advanced requirements, Microsoft is still recommending that we bring in a more fully-featured container instead.
DI is not a technique that all ASP.NET developers will be familiar with if they've only ever built fairly simple applications. As it's a core technique used in ASP.NET Core apps, if you aren't particularly comfortable with it, then I strongly recommend you go and read up on it. The Microsoft documentation for ASP.NET Core is a great place to start! We've already seen how to configure some of the built-in framework services using the extension methods provided by each package, but how do we register our own services? The answer is via a set of extension methods that provide a way of registering dependencies with different lifetimes:
services.AddTransient<ICartService, CartService>();
The services.AddSingleton() extension method is fairly self-explanatory, and is used for registering services that have a single instance which is shared by all dependent classes. The other two are slightly less obvious: services.AddScoped() is used for registering dependencies that are scoped to the lifetime of a request, that is, they are created once per request and each dependent class receives the same copy until the request terminates; services.AddTransient() is probably the most common, and simply means that each service is instantiated every time it is requested.
This is pretty much the limit of what we can do with the built-in container, and as previously mentioned, if you need any more complicated features, such as property injection and/or convention-based registrations, you'll need to look at a more complete container such as StructureMap.