You have been tasked with upgrading your Fibonacci sequence test code to use the Mocha test framework. Take the Fibonacci sequence code and test code you created for Activity 1 and upgrade it to use the Mocha test framework to test the code. You should write tests for the n=0 condition, then implement the n=0 condition, then write tests for and implement the n=1 condition, then write tests for and implement the n=2 condition, and finally do so for the n=5, n=7, and n=9 conditions. If the it()
test passes, call the done
callback with no argument. Otherwise, call the test done
callback with an error or other truthy value.
To utilize the Mocha test framework to write and run tests, follow these steps:
Set up the project directory with
npm run init.
Install mocha globally withnpm install mocha -s -g
.Create
index.js
to hold the code andtest.js
to hold the tests.Add the test script to
package.json
in thescripts
field. The test should call themocha
module and pass in thetest.js
file.Add the recursive Fibonacci sequence code to
index.js
. You can use the code built inExercise 24
.Export the function with
module.exports = { fibonacci }.
Import the Fibonacci function into the test file using the following command:
const { fibonacci } = require( './index.js' )
;Write a
describe
block for the tests. Pass in the stringfibonacci
and a callback functionCalculate the expected value by hand for each item in the fibonacci sequence (you can also look up the sequence on Google to avoid doing too much math by hand).
For each test condition (n=0, n=1, n=2, n=5, n=7, and n=9) do the following:
Create a mocha test with the
it()
function and pass in a description of the test as the first parameter.Pass a callback as the second parameter. The callback should take in one argument,
done
.Inside the callback call the fibonacci sequence and compare its result to the expected value with a not equal comparison.
Call the
done()
function and pass in the test comparison result.If the test fails, return
done( error )
. Otherwise, returndone(null)
ordone(false)
.Run the tests from the command line with
npm run test
.
Code:
test.js
'use strict'; const { fibonacci } = require( './index.js' ); describe( 'fibonacci', () => { it( 'n=0 should equal 0', ( done ) => { done( fibonacci( 0 ) !== 0 ); } ); it( 'n=1 should equal 1', ( done ) => { done( fibonacci( 1 ) !== 1 ); } ); it( 'n=2 should equal 1', ( done ) => { done( fibonacci( 2 ) !== 1 ); } ); it( 'n=5 should equal 5', ( done ) => { done( fibonacci( 5 ) !== 5 ); } ); it( 'n=7 should equal 13, ( done ) => { done( fibonacci( 7 ) !== 13 ); } ); it( 'n=9 should equal 34, ( done ) => { done( fibonacci( 9 ) !== 34 ); } ); } );
Snippet 4.9: Utilizing test environments
Take a look at the following output screenshot below:
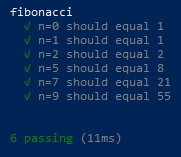
Figure 4.8 : Displaying the Fibonacci series
Outcome
You have successfully utilized the Mocha test framework to write and run tests.