Different types of APIs
There are several types of APIs commonly used on the internet. Before you dive too deep into the details of using Postman, it is worth knowing a bit about the different kinds of APIs and how to recognize and test them. In the following sections, I will provide brief explanations of the three most common types of APIs that you will see on the internet.
REST APIs
We'll start with what is probably the most common type of API you'll come across on the modern web, the RESTful API. REST stands for Representational State Transfer, and refers to an architectural style that guides how in terms of how you should create APIs. I won't go into the details of the properties that a RESTful API should have (you can look them up on Wikipedia if you want, at https://en.wikipedia.org/wiki/Representational_state_transfer), but there are a few clues that can let you know that you are probably testing a RESTful API.
Since RESTful APIs are based on a set of guidelines, they do not all look the same. There is no official standard that defines the exact specifications that a response must conform to. This means that many APIs that are considered to be RESTful do not strictly follow all the REST guidelines. REST in general has more flexibility than a standards-based protocol such as SOAP (covered in the next section), but this means that there can be a lot of diversity in the way REST APIs are defined and used.
So how do you know whether the API that you are looking at is RESTful?
Well, in the first place, what kind of requests are typically defined? Most REST APIs have GET, POST, PUT, and DELETE calls, with perhaps a few others. Depending on the needs of the API, it may not use all these actions, but those are the common ones that you will be likely to see if the API you are looking at is RESTful.
Another clue is in the types of requests or responses that are allowed by the API. Often, REST APIs will use JSON data in their responses (although they could use text or even XML). Generally speaking, if the data in the responses and requests of the API is not XML, there is a good chance you are dealing with a REST-based API of some sort. There are many examples of REST APIs on the web and, in fact, all the APIs that we have looked at so far in this book have all been RESTful.
SOAP APIs
Before REST, there was SOAP. SOAP stands for Simple Object Access Protocol. SOAP has been around since long before Roy Fielding came up with the concept of REST APIs. It is not as widely used on the web now (especially for smaller applications), but for many years it was the default way to make APIs and so there are still many SOAP APIs around.
SOAP is an actual protocol with a W3C standards definition. This means that its usage is much more strictly defined than REST, which is an architectural guideline as opposed to a strictly defined protocol.
If you want a little light reading, check out the w3 primer on the SOAP protocol (https://www.w3.org/TR/soap12-part0/). It claims to be a "non-normative document intended to provide an easily understandable tutorial on the features of SOAP Version 1.2.
I'll be honest, I'm not sure how well it delivers on the "easily understandable tutorial" part of that statement, but looking at some of the examples in there may help you understand why REST APIs have become so popular. SOAP APIs require a highly structured XML message to be sent with the request. Being built in XML, these requests are not that easy to read for humans and require a lot of complexity to build up. There are, of course, many tools (an example is SoapUI) that can help with this, but in general, SOAP APIs tend to be a bit more complex to get started with. You need to know more information (such as the envelope structure) in order to get started.
But how do you know whether the API you are looking at is a SOAP API?
The most important rule of thumb here is: does it require you to specify structured XML in order to work? If it does, it's a SOAP API. Since these kinds of APIs are required to follow the W3C specification, they must use XML, and they must specify things such as env:Envelope
nodes inside the XML. If the API you are looking at requires XML to be specified, and that XML includes the Envelope
node, you are almost certainly dealing with a SOAP API.
SOAP API example
Let's look at an example of what it would look like to call a SOAP API. This is a little bit harder than just sending a GET request to an endpoint, but Postman can still help us out with this. For this example, I will use the country info service to get a list of continents by name. The base page for that service is here: http://webservices.oorsprong.org/websamples.countryinfo/CountryInfoService.wso. In order to call this API in Postman, we will need to set up a few things. You will, of course, need to create a request in Postman. In this case though, instead of having the request method as a GET request, you will need to set the request method to POST and then put in the URL specified above. SOAP requests are usually sent with the POST method rather than the GET method because they have to send so much XML data. It is more difficult to send that data via a GET request, and so most SOAP services require the requests to be sent using the POST protocol.
Don't click Send yet though. Since this is a SOAP API, we need to send some XML information as well. We want to get the list of continents by name, so if you go to the CountryInfoServices web page, you can click on that first link in the list, which will show you the XML definitions for that operation. Use the SOAP 1.2 example on that page and copy the XML for it.
In Postman, you will need to set the input body type to raw and choose XML from the dropdown and then paste in the Envelope data that you copied from the documentation page. It should look something like this:
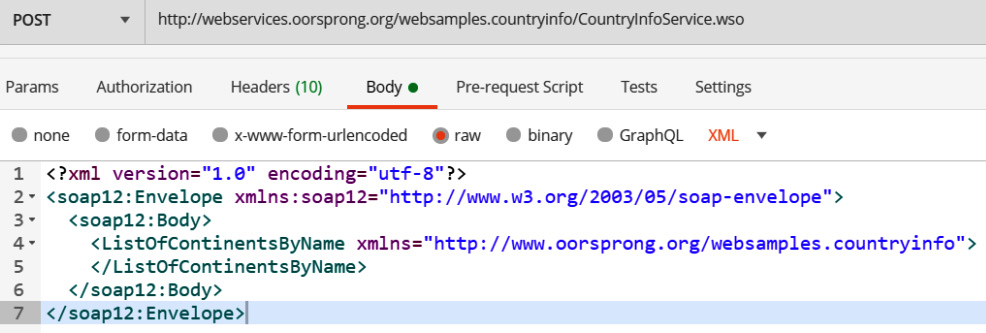
Figure 1.8 – SOAP API information
For this particular API, we would also need to modify the Content-Type
header (by adding a new one) at the bottom, so that it is set to application/soap+xml
:
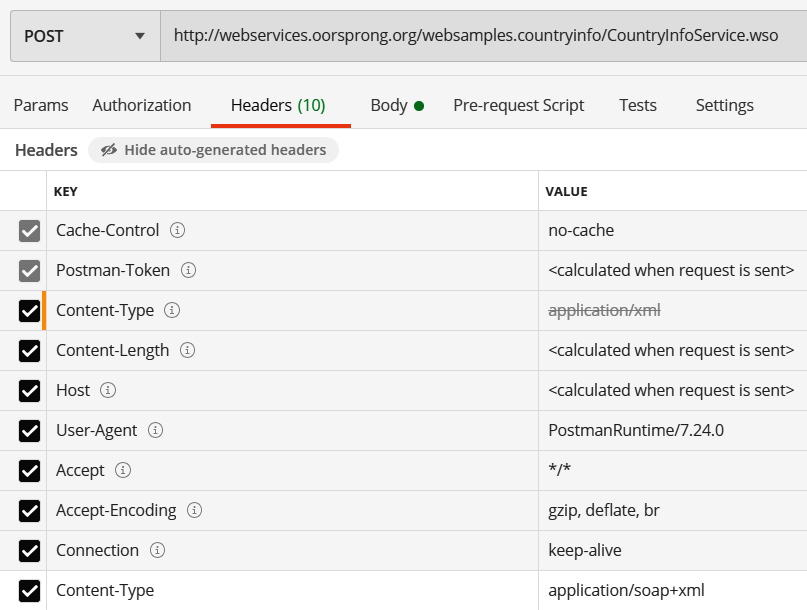
Figure 1.9 – Content-Type header set to application/soap+xml
Now you are finally ready to click on Send. You should get back a list of the continents, but as you can see, there is a lot more complexity to calling SOAP APIs. REST APIs can, of course, have complex bodies specified as well, but the requirement to do this in XML and the existence of the Envelope
node in this indicates that this API is indeed a SOAP API.
GraphQL APIs
SOAP came before REST and, in many ways, REST was designed to deal with some of the shortcomings of SOAP. Of course, in software we are never done making things better, and so we now have a type of API known as GraphQL. GraphQL is a query language and it was designed to deal with some of the situations where REST APIs have shortcomings. RESTful APIs don't know what specific information you might be looking for, and so when you call a REST API endpoint, it gives back all the information it has. This can mean that we are sending extra information that you don't need, or it can mean that we aren't sending all the information you need and that you must call multiple endpoints to get what you want. Either of these cases can slow things down, and for big applications with many users, that can become problematic. GraphQL was designed by Facebook to deal with these issues.
GraphQL is a query language for APIs, and so it requires you to specify in a query what you are looking for. With REST APIs, you will usually only need to know what the different endpoints are in order to find the information you are looking for, but with a GraphQL API, a single endpoint will contain most or all of the information you need and you will use queries to filter down that information to only the bits that you are interested in. This means that with GraphQL APIs, you will need to know the schema or structure of the data so that you know how to properly query it, instead of needing to know what all the endpoints are.
How do you know whether the API you are looking at is a GraphQL API?
Well, if the documentation is telling you about what kinds of queries you need to write, you are almost certainly looking at a GraphQL API. In some ways, a GraphQL API is similar to a SOAP API in that you need to tell the service some information about what you are interested in. However, a SOAP API will always use XML and follow a strict definition in the calls, whereas GraphQL APIs will usually be a bit simpler and are not defined in XML. Also, with GraphQL, the way the schema is defined can vary from one API to another as it does not need to follow a strictly set standard.
GraphQL API example
Let's take a look at a real-life example of calling a GraphQL API to understand it a bit better. This example will use a version of the countries API that you can find hosted at https://countries.trevorblades.com/. You can find information about the schema for it on GitHub: https://github.com/trevorblades/countries. Now, you could just create queries in the playground provided, but for this example, let's look at setting it up in Postman.
Similar to calling a SOAP API, we will need to specify the service we want and do a POST request rather than a GET request. GraphQL queries can be done with GET, but it much easier to specify the query in the body of a POST request, so most GraphQL API calls are sent with the POST method. You will need to choose the GraphQL option on the Body tab and put in the query that you want:
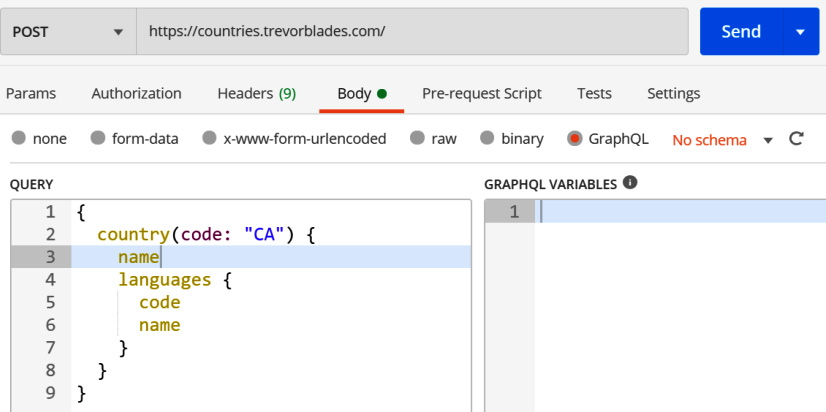
Figure 1.10 – GraphQL query
As you can see, in this example, I have requested the name and languages of Canada. Once I have specified this information, I can click Send and I get back some JSON with the country name and a list of the official languages. If I wanted additional information (say the name of the capital city), I could just modify the query to include a request for that information and send it off again using the same endpoint, and I would get back the new set of information that I requested.
At this point, I have obviously only been able to give a very quick introduction to each of these types of APIs. If you are working with a GraphQL or SOAP API, you may need to spend a bit of time at this point making sure you understand a little more about how they work before proceeding on with this book. Most of the examples through the rest of this book will use RESTful APIs. The concepts of API testing will largely stay the same though, regardless of the type of API testing that you need to do. You should be able to take the things that you will learn in this book and put them to use regardless of the type of API you are working with in your day job.