Moodle is a highly-configurable application, with many complex settings in many areas of its functionality. These settings can be modified by Moodle administrators through:
Direct code in the main
config.php
fileThe
mdl_config
table via administrative code and interfacesThe
mdl_config_plugins
table via plugin administration
During program execution, all of the main configuration values are stored in the $CFG
global variable. $CFG
is a generic structure. Each configuration variable is an element of the $CFG
structure. For example, the $CFG->theme
contains the text name of your site's selected theme.
The elements of $CFG
are loaded by direct assignment, and from values stored in the database mdl_config
table. The primary point of direct assignment takes place in /config.php
. Here, the initial, necessary configuration settings are made.
There are minimal assignments that must be defined in this file. You are prompted for these values when you install Moodle, and you can change them afterwards by editing this file. The minimal settings are as follows:
$CFG->dbtype = 'mysql'; // mysql or postgres7 (for now) $CFG->dbhost = 'localhost'; // eg localhost or db.isp.com $CFG->dbname = 'moodle'; // database name, eg moodle $CFG->dbuser = 'username'; // your database username $CFG->dbpass = 'password'; // your database password $CFG->prefix = 'mdl_'; // Prefix to use for all table names $CFG->dbpersist = false; // Should database connections be // reused? $CFG->wwwroot = 'http://example.com/moodle'; $CFG->dirroot = '/home/example/public_html/moodle'; $CFG->dataroot = '/home/example/moodledata'; $CFG->directorypermissions = 02777; $CFG->admin = 'admin';
There are other optional settings that can be made in this file. Review your /config-dist.php
file to see all of these settings.
Historically, any configuration settings that did not have a UI available to set these were set via code statements in this file. These often include new, experimental features.
The remainder of the $CFG
elements are set from the database. This is done through a call to the library function get_config
(located in the /lib/moodlelib.php
library file) from /lib/setup.php
, which is included as the last action of config.php
. The important thing to know about the function get_config
is that it will not overwrite any $CFG
setting that has already been set. This means you can overrule any database setting by hardcoding it into config.php
. Also, config.php
clears out the $CFG
structure before it does anything else. This guarantees that nothing sets any configuration variables before config.php
and setup.php
.
As a developer, you can set and use configuration variables for your functionality. You can set a configuration variable through the set_config
function. The set_config
function takes a name, a value, and an optional plugin name as arguments. The name becomes the element of the $CFG
structure and the passed value becomes its value. Additionally, this will be stored into the database mdl_config
table, so that it can be loaded on every program execution from then on. You can also specifically request a configuration variable at any time, by using the get_config
function, but you won't need to, because it will be loaded into the $CFG
structure once it has been set.
The one issue that you need to consider when creating your own configuration variable is that the variable name you choose must be unique. Your variable becomes meaningless if you choose one that already exists. As most customizations you will be developing are likely to be plugins (modules, blocks, and so on), Moodle provides an alternate way to store configuration variables that are plugin specific.
Plugin configuration variables are loaded into the plugin structure itself, rather than into the $CFG
global structure. This means that the configuration variable name only needs to be unique to the plugin. Plugin configuration variables are stored in the mdl_config_plugin
table, with the name of the plugin. They can be set and retrieved by using the same set_config
and get_config
functions as before.
Generally, configuration variables are set by using administration interfaces. Most of the Moodle configuration variables are set through the various settings pages in the Site Administration menu block, which is visible on the home page of your Moodle site. In future sections, you will learn how the plugins add their interfaces to this structure. The following screenshot displays an example of Moodle's Site Administration block:
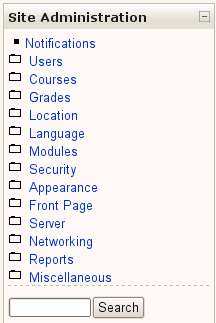