Maven is a software tool for build and project management. It uses a construct known as the Project Object Model (POM), which describes the components of your project and dependency to build the project in an XML format. Maven provides various pre-defined tasks, which facilitate build management and allow extensions to add more specific build tasks. Understanding and using Maven effectively is a vast topic, and there are a lot of books dedicated to using Maven effectively. We intend to provide a very short overview on Maven for readers who are not familiar with the tool.
A key concept in Maven is that of artifacts, a packaged archive like a JAR or WAR, which is created by the build and stored in a repository. Maven maintains artifacts in a repository, indexed by Group ID which specifies the group, Artifact ID which specifies the name of the artifact, and Version which specifies the version number of the artifact. For instance, in the case of the CXF JAX-WS component, the group ID is org.apache.cxf
, artifact ID is cxf-rt-frontend-jaxws
, and the version is 2.2.3
(or the latest). When you build using the Maven tool, a local repository is created for you, typically in your home drive, that is, C:\Documents and Settings\userName\.m2\repository
and all the dependent artifacts required for building the project are copied in their respective groups\artifacts\version
folder in the local repository. Note that while building the project, the Maven tool first checks if the required artifact exists in the local repository and then looks up the Maven central repository (or the repository specified) to download the artifact from the Internet.
Apache CXF also supports Maven-based build and installation and provides various tasks which simplify CXF application management. The CXF artifacts can be accessed from the Maven central repository itself. The complete release is available at the following location: http://repo1.maven.org/maven2/org/apache/cxf/
The following shows an example of POM, and how dependencies are declared to build applications which use the CXF framework using Maven:
<properties> <!-- CXF Version --> <cxf.version>2.2.3</cxf.version> </properties> <dependencies> <dependency> <groupId>org.apache.cxf</groupId> <artifactId>cxf-rt-frontend-jaxws</artifactId> <version>${cxf.version}</version> </dependency> <dependency> <groupId>org.apache.cxf</groupId> <artifactId>cxf-rt-transports-http</artifactId> <version>${cxf.version}</version> </dependency> <dependency>
Each dependency is listed as the <dependency>
tag, with the <groupId>
, <artifactId>
, and <version>
We will show how to use Maven for building the source code as part of Chapter 2. The following shows the pom.xml
from the Chapter2\orderapp
folder of the source code download. Please refer to the inline comments, which are highlighted in bold for an explanation of the tags.
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd"> <modelVersion>4.0.0</modelVersion> <!-- Defines group id for cxfbook --> <groupId>com.packtpub.cxfbook</groupId> <!-- Name of the artifact --> <artifactId>orderapp</artifactId> <!-- Packing format. We want to pakacge this as a WAR archive --> <packaging>war</packaging> <!-- Version for the oderapp arifact --> <version>1.0-SNAPSHOT</version> <name>orderapp maven webapp</name> <properties> <!-- Version of CXF. Change this to latets version for building against latest CXF distribution --> <cxf.version>2.2.3</cxf.version> </properties> <build> <!--Directory where the source code is located--> <sourceDirectory>src</sourceDirectory> <resources> <resource> <!-- Include properties and xml file from src folder--> <directory>src</directory> <includes> <include>**/*.properties</include> <include>**/*.xml</include> </includes> </resource> </resources> <plugins> <plugin> <!-- Maven Plugin used to build WAR archive--> <artifactId>maven-war-plugin</artifactId> <version>2.0</version> <configuration> <!-- Directory for Web application--> <webappDirectory>webapp</webappDirectory> <webResources> </webResources> </configuration> </plugin> <plugin> <!-- Plugin for compiling Java code --> <artifactId>maven-compiler-plugin</artifactId> <configuration> <!-- Java version for compiling the source code--> <source>1.5</source> <target>1.5</target> </configuration> </plugin> </plugins> <finalName>orderapp</finalName> </build> <dependencies> <dependency> <!-- Apache JAX-WS CXF Dependency for WAR and JAX-WS Client--> <groupId>org.apache.cxf</groupId> <artifactId>cxf-rt-frontend-jaxws</artifactId> <version>${cxf.version}</version> </dependency> <dependency> <!-- Apache JAX-WS CXF Dependency for HTTP transport--> <groupId>org.apache.cxf</groupId> <artifactId>cxf-rt-transports-http</artifactId> <version>${cxf.version}</version> </dependency> </dependencies> </project>
To build the Chapter 2 source code, navigate to the Chapter2\orderapp
folder, and type in the following command:
mvn clean install
You will see the following build output, and the WAR file will be generated in the orderapp/target
folder. You can then deploy the WAR file in the Tomcat server.
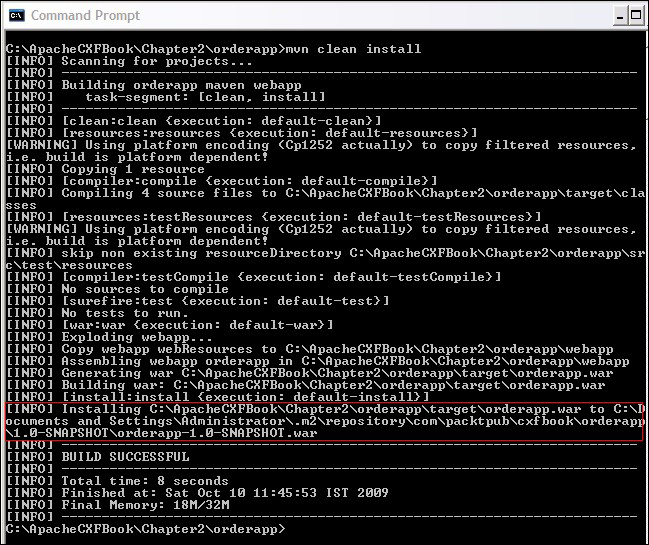
Alternatively, if you wish to deploy using a standalone web server like Jetty, then you can add the following plugin in the POM
file:
<plugins> <plugin> <groupId>org.mortbay.jetty</groupId> <artifactId>maven-jetty-plugin</artifactId> <version>6.1.19</version> </plugin>
You can run the server by giving the following command:
mvn jetty:run
The previous command will start the WAR on localhost port 8080
Once the WAR file is deployed, run the client, and this will invoke the Order Process web service by typing in the following command from the Chapter2\orderapp
folder:
mvn exec:java -Dexec.mainClass=demo.order.client.Client
You will see the following output and "order ID is ORD1234" being printed at the console:
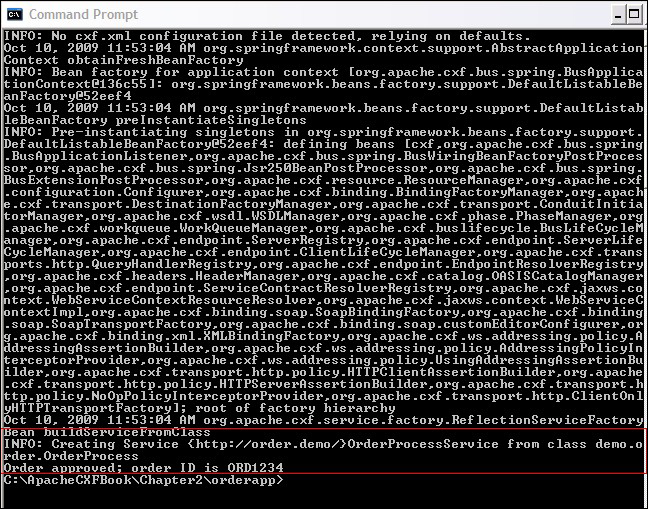
We have thus successfully built, deployed, and executed the code using the Maven tool.