Storyboards are Silverlight entities that are used for describing the animations of various dependency and attached properties. They consist of one or several animation entities each one of which is dedicated to animating just one dependency property. This section gives an overview of storyboard and animation functionality.
We are about to make this rectangle rotate by changing the dependency property, that is, RotationAngle
using a storyboard.
The storyboard can be created as the page's resource by adding the following XAML code above the <Grid x:Name="LayoutRoot" …>
line:
<Storyboard x:Key="RotationStoryboard" Storyboard.TargetName="TheSpinningControl" Storyboard.TargetProperty="(SpinningControlSample:SpinningControl.RotationAngle)"> <DoubleAnimation BeginTime="00:00:00" Duration="00:00:01" From="0" To="360" RepeatBehavior="Forever" /> </Storyboard>
Once this storyboard runs, it will change the RotationAngle
property on the visual element called TheSpinningControl
from 0 to 360 over a period of 1 second and then continue repeating the same change forever, which will result in a rotating rectangle.
The only thing remaining is to start the storyboard based on some event.
We are going to add a button at the bottom of the window, which when clicked will start the rotation. Here is the code we need to add to our XAML file under the </SpinningControl>
end tag in order to create the button:
<Button x:Name="StartRotationButton" Content="Start Rotation" VerticalAlignment="Bottom" HorizontalAlignment="Center" Margin="0,0,0,20" Width="100" Height="25"> </Button>
It would be easy to get the reference to the button, within the MainPage.xaml.cs
(code-behind) file and add a handler to the button's Click
event to pull the storyboard out of the page's resource and start it. However, we do not want to split the button click action triggering a storyboard from the XAML code which defines both the button and the storyboard. This is where MS Expression Blend SDK, mentioned in the Preface, comes in handy.
MS Expression Blend SDK does not require having MS Expression Blend installed. It is simply a number of free and redistributable DLLs that make Silverlight/WPF programming easier.
One can download MS Expression Blend SDK using the URL specified in the Preface, or simply use the two files, Microsoft.Expression.Interactions.dll
and System.Windows.Interactivity.dll
, that come with our code. These files are located in the MSExpressionBlendSDKDlls
folder and you need to add references to them in our SpinningControlSample
project. MS Expression Blend SDK allows us to connect the Click
button event to the ControlStoryboardAction
functionality that starts the animation without any C# code. Also, we can disable the button once it is clicked, by using MS Expression Blend SDK's ChangePropertyAction
object.
Add the following namespace reference to the <UserControl…
tag at the top of the MainPage.xaml
file:
<UserControl … …xmlns:i="http://schemas.microsoft.com/expression/2010/interactivity" xmlns:se="http://schemas.microsoft.com/expression/2010/interactions" …/>
Now we can use i:
and se:
prefixes to access the MS Expression Blend functionality within XAML.
To start the storyboard on a button click, add the following XAML code between the button's start and end tags:
<i:Interaction.Triggers> <!-- MS Expression Blend SDK trigger will start on "Click" event of the button--> <i:EventTrigger EventName="Click"> <!-- ChangePropertyAction below will disable the StartRotationButton after it is clicked first time --> <se:ChangePropertyAction TargetObject="{Binding ElementName=StartRotationButton}" PropertyName="IsEnabled" Value="False" /> <!-- ControlStoryboardAction will start the RotationStoryboard --> <se:ControlStoryboardAction ControlStoryboardOption="Play" Storyboard="{StaticResource RotationStoryboard}" /> </i:EventTrigger> </i:Interaction.Triggers>
You can run the sample now. Once you click the button, the orange rectangle in the middle starts rotating and the button gets disabled:
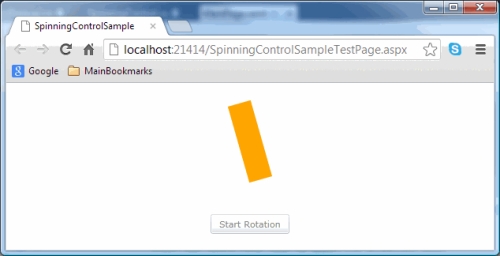
As we learned earlier, Silverlight Storyboards
consist of one or more animation objects. Each animation object controls an animation of one and only one dependency property. Note that only dependency or attached properties can be animated by Silverlight animations. There are two types of animation classes:
Simple animations (that have properties
to
,from
, andby
): Such animations change the dependency property linearly in time (unless easing is used). The propertiesto
andfrom
specify the dependency property value in the beginning and end of the iteration. Using the propertyby
, you can specify by how much the animation should change (obviously if you use the propertyby
, the other two properties are redundant – the animation will simply increase the current value by the value specified in the propertyby
). You can also specify at what point an animation should start, and how long it should last by using theBeginTime
andDuration
properties of the animation class. Since the animations control DPs of different types, there is a specific built-in animation class for every type that is likely to be animated. Animation names usually start with the name of the type. The following Silverlight simple animations are the most important ones:DoubleAnimation
: This animates a double DP (we used it previously to animate theRotationAngle
DP of theSpinningControl
object).ColorAnimation
: This animates color transitions.PointAnimation
: This animatesPoints
, that is, pairs ofdouble
values.
Key frame animations: These animations also allow us to specify property values at certain points between the beginning and the end of the iteration. Correspondingly, key frame animations do not have
to
,from
, andby
properties. Instead, such animations have theKeyFrames
property – a collection that can be populated with objects of the key frame class. Key frame classes differ by the type of interpolation that they use in order to interpolate the value between the key frame times. There areDiscrete
,Linear
, andSpline
interpolations. The names of key frame classes are composed of the interpolation type, animation type (corresponding to the DP type), andKeyFrame
suffix, for example,LinearDoubleKeyFrame
. Key frame animation class names start with the interpolation type followed by the animation type and end with theUsingKeyFrames
suffix. The most important key frame animations are:DoubleAnimationUsingKeyFrames
ColorAnimationUsingKeyFrames
PointAnimationUsingKeyFrames