Paging and sorting a table adds interactivity to your site, and can help your users locate specific bits of information. But if they know what they're looking for, rather than having to page or sort, it would be efficient to give them a filter field.
We've come to love it, so stick with the table of movies. This time, underneath the table, add a form field.
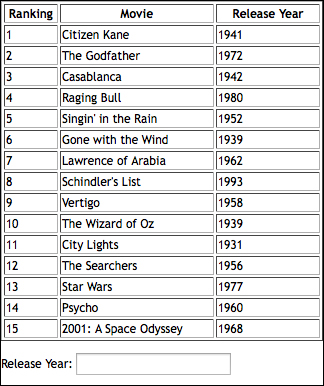
You should be able to bang out this particular table code in your sleep, so we'll forego displaying that code. But underneath the table, add a form field as follows:
<div> Release Year: <input type="text" name="filter" id="filter" /> </div>
The script will let users filter the release year. As they type into the form field, the
keyup
event will trigger the jQuery function, which will hide any rows that don't contain the text input by the user:<script type="text/javascript"> $( document ).ready( function() { $( "input#filter" ).keyup( function() { var filtertext = $( this ).val(); $( "#movies tbody tr" ).each( function( rowindex ) { $( this ).children( "td" ).eq( 2 ).each( function( cellindex ) { if ( $( this ).text().indexOf( filtertext ) < 0 ) { $( this ).parents( "tr" ).hide(); } else { $( this ).parents( "tr" ).show(); } }); }); }) }); </script>
Start off by listening for a keyup
event this time, rather than the click
event that has been used in previous recipes. It's implemented the same way:
$( "input#filter" ).keyup( function() {
The first thing to do is to store the input in a local variable:
var filtertext = $( this ).val();
Now compare that text with the text in the Release Year column. To accomplish this, use two .each()
loops.
The first loop will iterate over all of the rows in the tbody
:
$( "#movies tbody tr" ).each( function( rowindex ) {
With each loop over the table rows, then loop over each td
at index position 2. Say it with me, indexes start at 0, so position 2 is column 3.
$( this ).children( "td" ).eq( 2 ).each( function( cellindex ) {
With each iteration of the inner loop, compare the text in the td
element to the text in the filter input. As long as the text in the td
contains a substring of the text in the filter input, display it. For example, 193 would display 1932 and 1939, but would hide 1940, 1941, and so on. Do this with JavaScript's indexOf()
function. indexOf()
checks for the existence of a substring within a string. If the substring is not found, it returns -1
. Otherwise, it returns the starting position of the first instance of the substring. For now, know that if the value returned is less than 0, the substring was not found, so hide the row.
if ( $( this ).text().indexOf( filtertext ) < 0 ) { $( this ).parents( "tr" ).hide(); } else { $( this ).parents( "tr" ).show(); }
Beyond just hiding rows that don't meet the criteria, use .show()
to display rows that do, as they may have been hidden before, but now meet the filter criteria.
Filter functions can be very powerful. This was just a basic example of how to demonstrate the concept. You could have iterated over every table cell, or given the user a drop-down menu to select the column on which they want to filter. You also could have provided a submit button that filtered only when the button was clicked, rather than on each keyup.