I know what you've been thinking all this time. "Charlie, why do I need to know how to do these things when there are so many plugins available for jQuery?" That's a very good question.
There are numerous plugins available for jQuery, and specifically for table manipulation. Sometimes the plugins are overkill, though. Maybe a particular plugin doesn't work exactly the way you'd like it to. Perhaps your employer is shy about using third-party code (except, of course, for jQuery itself).
Plugins are great, and they are a significant part of the reason that jQuery is so popular. It's always good to know how to do something on your own, though. Of course once you know how, it never hurts to work smarter, and not harder.
There are two table-related plugins that I generally use when the need arises: tablesorter (http://tablesorter.com/docs/) and DataTables (http://www.datatables.net/).
For both tablesorter and DataTables, download the plugins to your server and call them from your pages. You still need to include jQuery, as the plugins assume you have jQuery set up properly.
After downloading the .js
files from the URLs listed above, add appropriate link's paths to your page's <head>
section. For example:
<script type="text/javascript" src="/path/to/jquery.tablesorter.min.js"></script> <script type="text/javascript" src="/path/to/jquery.datatables.min.js"></script>
Some plugins also offer .css
files, which will be needed to achieve a particular look and feel. We'll forego the aesthetics and dive right into functional implementation.
Both tablesorter and DataTables can be applied to any well-marked up HTML table. As per usual, assume a table with an ID of
movies
.To apply tablesorter to the table, add the following script to the bottom of the page:
<script type="text/javascript"> $( document ).ready( function() { $( "#movies" ).tablesorter(); }); </script>
To apply DataTables to the table, add the following script to the bottom of the page:
<script type="text/javascript"> $( document ).ready( function() { $( "#movies" ).dataTable(); }); </script>
It may be worth pointing out that you would apply one plugin or the other to your
#movies
table, but not both!
For basic functionality, there's very little magic. Simply include the scripts as shown and the rest just works. That's the beauty of the extensible jQuery plugin architecture.
You might not notice any significant changes to the appearance of your table with either plugin. Although with DataTables you'll see some extra goodies around the table, such as a dropdown to display "n" records, a filter form field, and Previous/Next links for pagination. See the following screenshot for reference:
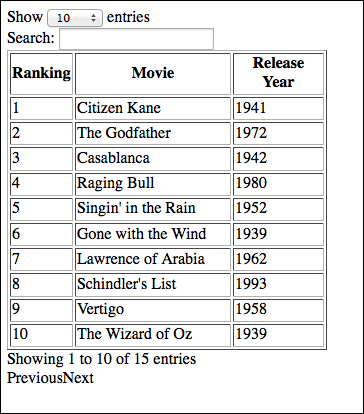
Both tablesorter and DataTables include CSS files with their downloads. Include them into the page in much the same way that you included the .js
files, and you will have tables that are not only functional but also aesthetically pleasing.
Add the following line to your tablesorter page:
<link rel="stylesheet" href="tablesorter.css" type="text/css" media="print, projection, screen" />
The preceding code yields the following table:
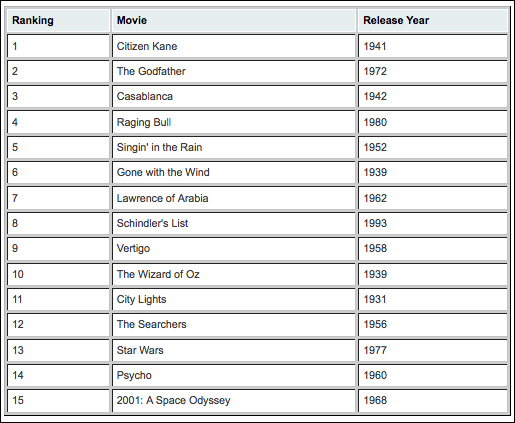
Likewise, add the following line of code to your DataTables page:
<link rel="stylesheet" href="dataTables.css" type="text/css" media="print, projection, screen" />
The preceding code yields the following table:
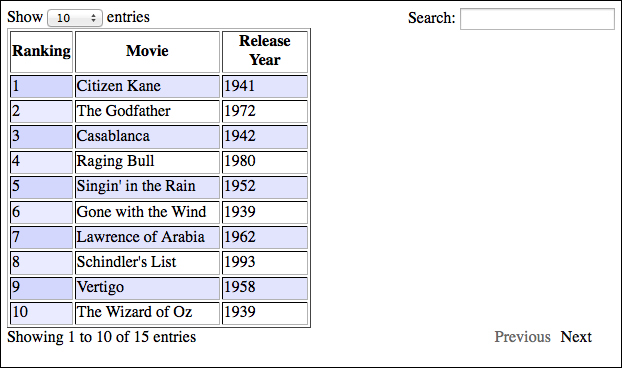
Both downloads include additional stylesheets as well as images, such as up/down arrows for column headers to indicate sorting. Additionally, you can tweak the styles as you see fit.
As impressive as the out-of-the-box functionality may be, both plugins also offer a number of options.
Tablesorter shows a number of examples at http://tablesorter.com/docs/#Examples. They include forcing a default sort order, appending table data with AJAX, and sorting fields that contain markup (for example, ignoring the markup and sorting on the text).
DataTables examples are located at http://www.datatables.net/examples/. While by default both tablesorter and DataTables can be utilized in a single line of code, and while they both have various options, DataTables is insanely configurable.
No disrespect to tablesorter. It essentially does one thing, and it does it well. If table sorting is all that you need, DataTables may be overkill. But for a Swiss army knife of table-related functionality, DataTables is impressive.