I can feel now that you have seen how easy is to bind the properties of the model to the presentation layer and you are already starting to think about how it can be used in your existing project. But then you think: hell, no, I need to install some things, run npm install
, change the project's structure, add directives, and change the code.
And here I can tell you: no! No installs, no npms, just grab the vue.js
file, insert it into your HTML page, and use it. That's all, no structure changes, no architectural decisions, no discussions. Just use it. I will show you how we used it at EdEra (
https://www.ed-era.com
) to include a small "check yourself" functionality at the end of a GitBook chapter.
EdEra is a Ukraine-based online educational project whose aim is to transform the whole educational system into something modern, online, interactive, and fun. Actually, I am a co-founder and the chief technical officer of this young nice project, being responsible for the whole technical part of the thing. So, in EdEra, we have some online courses built on top of the open EdX platform ( https://open.edx.org/ ) and some interactive educational books built on top of the great GitBook framework ( http://www.gitbook.org ). Basically, GitBook is a platform based on top of the Node.js technology stack. It allows someone with basic knowledge of the markdown language and basic Git commands to write books and host them in the GitBook servers. EdEra's books can be found at http://ed-era.com/books (beware, they are all in Ukrainian).
Let's see what we have done in our books using Vue.js.
At some point, I decided to include a small quiz at the end of the chapter about personal pronouns in the book that teaches English. Thus, I've included the vue.js
JavaScript file, edited the corresponding .md
file, and included the following HTML code:
<div id="pronouns"> <p><strong>Check yourself :)</strong></p> <textarea class="textarea" v-model="text" v- on:keyup="checkText"> {{ text }} </textarea><i v-bind:class="{ 'correct': correct, 'incorrect': !correct }"></i> </div>
Then I added a custom JavaScript file, where I've included the following code:
$(document).ready(function() { var initialText, correctText; initialText = 'Me is sad because he is more clever than I.'; correctText = 'I am sad because he is more clever than me.'; new Vue({ el: '#pronouns', data: { text: initialText, correct: false }, methods: { checkText: function () { var text; text = this.text.trim(); this.correct = text === correctText; } } }); });
Note
You can check this code at this GitHub page:
https://github.com/chudaol/ed-era-book-english
.
Here's a code of a page written in markdown with inserted HTML:
https://github.com/chudaol/ed-era-book-english/blob/master/2/osobovi_zaimenniki.md
.
And here's a JavaScript code:
https://github.com/chudaol/ed-era-book-english/blob/master/custom/js/quiz-vue.js
.
You can even clone the repository and try it locally using gitbook-cli
(
https://github.com/GitbookIO/gitbook/blob/master/docs/setup.md
).
Let's have a look at this code. You have probably already detected the parts that you have already seen and even tried:
- The
data
object contains two properties:- The
string
property text - The
Boolean
property correct
- The
- The
checkText
method just grabs thetext
property, compares it with the correct text, and assigns the value to the correct value - The
v-on
directive calls thecheckText
method on keyup - The
v-bind
directive binds the classcorrect
to thecorrect
property
Here is how the code looks in my IDE:
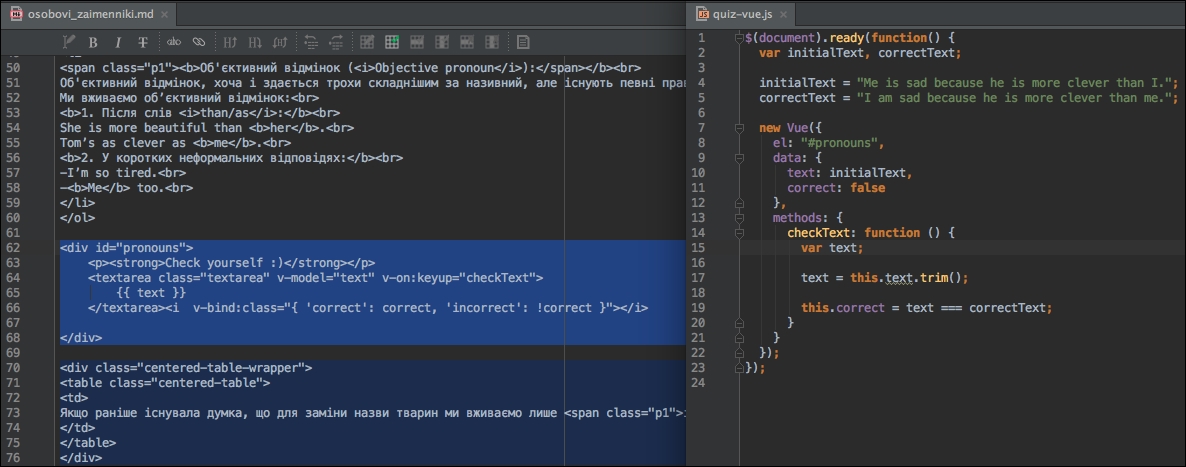
Using Vue in a markdown-driven project
And next is what it looks like in the browser:
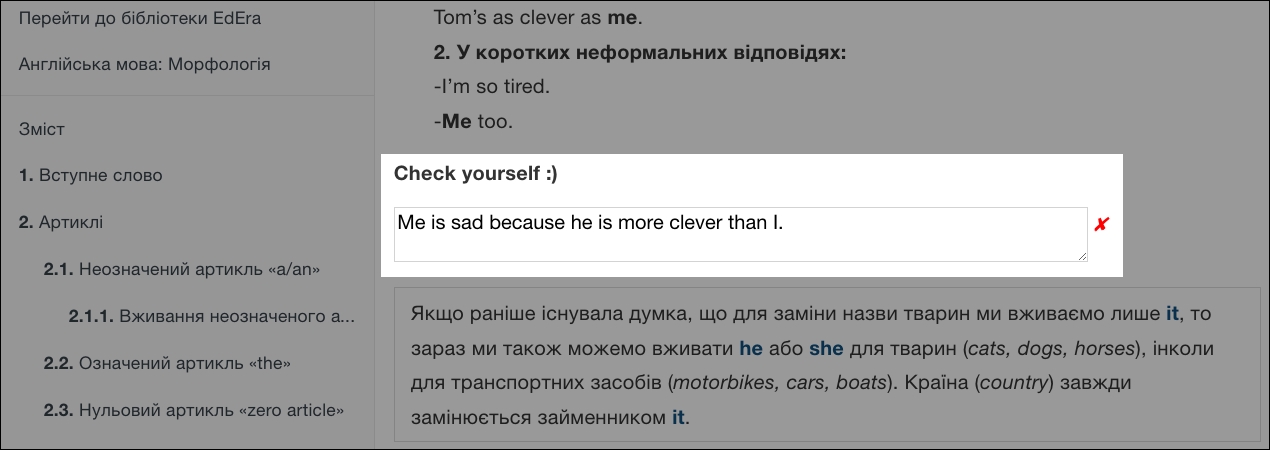
Vue.js in action inside the GitBook page
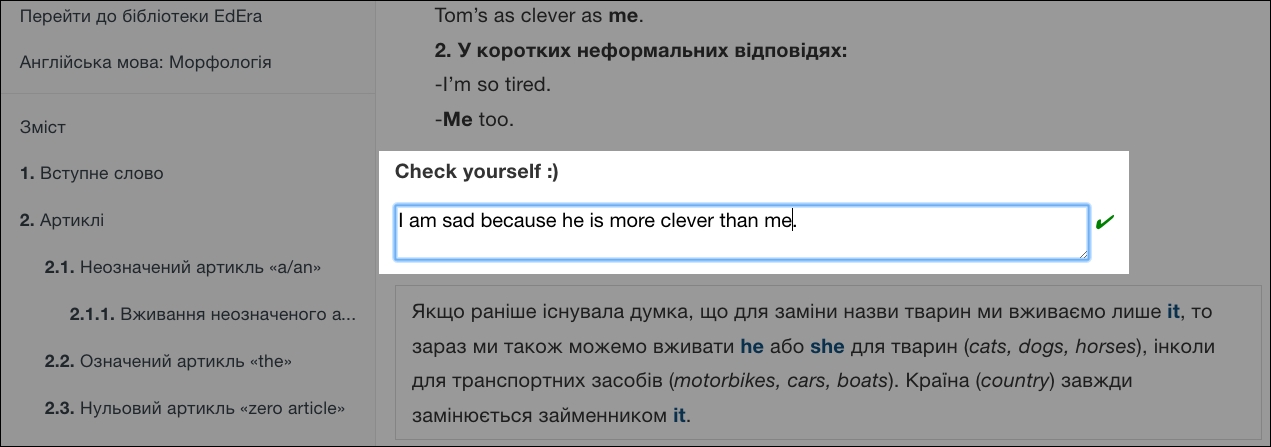
Vue.js in action inside the GitBook page
Check it out at http://english.ed-era.com/2/osobovi_zaimenniki.html .
Amazing, right? Pretty simple, pretty reactive!