For now, we don't have any Vue app running on our web page. The whole library is based on Vue instances, which are the mediators between your View and your data. So, we need to create a new Vue instance to start our app:
// New Vue instance var app = new Vue({ // CSS selector of the root DOM element el: '#root', // Some data data () { return { message: 'Hello Vue.js!', } }, })
The Vue constructor is called with the new
keyword to create a new instance. It has one argument--the option object. It can have multiple attributes (called options), which we will discover progressively in the following chapters. For now, we are using only two of them.
With the el
option, we tell Vue where to add (or "mount") the instance on our web page using a CSS selector. In the example, our instance will use the <div id="root">
DOM element as its root element. We could also use the $mount
method of the Vue instance instead of the el
option:
var app = new Vue({
data () {
return {
message: 'Hello Vue.js!',
}
},
})
// We add the instance to the page
app.$mount('#root')
We will also initialize some data in the data
option with amessage
property that contains a string. Now the Vue app is running, but it doesn't do much, yet.
Note
You can add as many Vue apps as you like on a single web page. Just create a new Vue instance for each of them and mount them on different DOM elements. This comes in handy when you want to integrate Vue in an existing project.
An official debugger tool for Vue is available on Chrome as an extension called Vue.js devtools
. It can help you see how your app is running to help you debug your code. You can download it from the Chrome Web Store (https://chrome.google.com/webstore/search/vue) or from the Firefox addons registry (https://addons.mozilla.org/en-US/firefox/addon/vue-js-devtools/?src=ss).
For the Chrome version, you need to set an additional setting. In the extension settings, enable Allow access to file URLs
so that it can detect Vue on a web page opened from your local drive:

On your web page, open the Chrome Dev Tools with the F12 shortcut (or Shift + command + c on OS X) and search for the Vue
tab (it may be hidden in the More tools...
dropdown). Once it is opened, you can see a tree with our Vue instance named Root
by convention. If you click on it, the sidebar displays the properties of the instance:
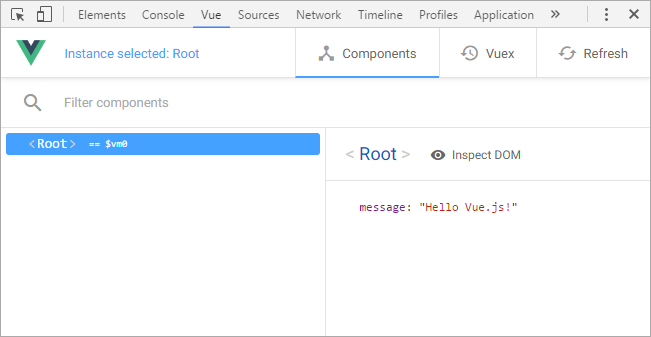
Note
You can drag and drop the devtools
tab to your liking. Don't hesitate to place it among the first tabs, as it will be hidden in the page where Vue is not in development mode or is not running at all.
You can change the name of your instance with the name
option:
var app = new Vue({
name: 'MyApp',
// ...
})
This will help you see where your instance in the devtools is when you will have many more:
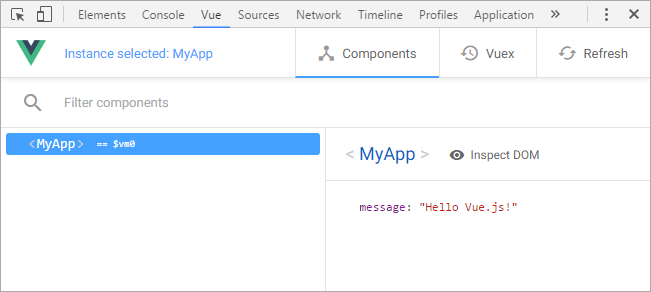