Let's take a moment and compare a monolithic application with a microservice application. The following diagram shows the most obvious difference between microservices and a monolith; monoliths are everything in one piece as opposed to microservices, which are split up in to different parts:
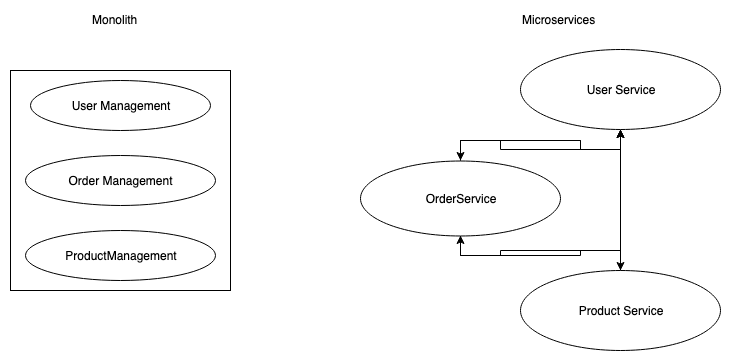
As you can see, the most obvious difference is that a monolith is one code base. Every code is within the same project and connected. In contrast to that, microservices are loose and independent but are often working together.
In the following subsections, we will compare concepts that highlight the difference between microservices and monoliths:
- Reusable code base
- Multiple versions online
- No downtime
- Updates are as slow or fast as required
- Common microservice use cases
Let's start by looking at the reusable code base.