Adding JavaScript to a web page
There are two ways to link JavaScript to a web page. The first way is to type the JavaScript directly in the HTML between two <script>
tags. In HTML, the first tag, <script>
, is to declare that the following script will be executed. Then we have the content that should be inside this element. Next, we close the script with the same tag, but preceded by a forward slash, </script>
. Or you can link a JavaScript file to the HTML file using the script tag at the head of the HTML page.
Directly in HTML
Here is an example of how to write a very simple web page that will give a pop-up box saying Hi there!
:
<html>
<script type="text/javascript">
alert("Hi there!");
</script>
</html>
If you store this as a .html
file, and open the file in your browser, you will get something like the following screenshot. We will be storing this one as Hi.html
:
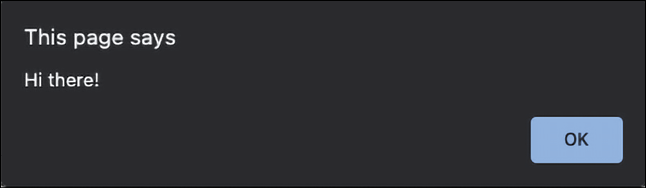
Figure 1.3: JavaScript made this popup with the text "Hi there!" appear
The alert
command will create a popup that provides a message. This message is specified between the parentheses behind the alert.
Right now, we have the content directly within our <html>
tags. This is not a best practice. We will need to create two elements inside <html>
—<head>
and <body>
. In the head element, we write metadata and we also use this part later to connect external files to our HTML file. In the body, we have the content of the web page.
We also need to let the browser know what kind of document we're working on with the <!DOCTYPE>
declaration. Since we're writing JavaScript inside an HTML file, we need to use <!DOCTYPE html>
. Here's an example:
<!DOCTYPE html>
<html>
<head>
<title>This goes in the tab of your browser</title>
</head>
<body>
The content of the webpage
<script>
console.log("Hi there!");
</script>
</body>
</html>
This example web page will display the following: The content of the webpage
. If you look in the browser console, you'll find a surprise! It has executed the JavaScript as well and logs Hi there!
in the console.
Practice exercise 1.2
- Open your code editor and create an HTML file.
- Within your HTML file, set up the HTML tags, doctype, HTML, head, and body, and then proceed and add the script tags.
- Place some JavaScript code within the script tags. You can use
console.log("hello world!")
.
Linking an external file to our web page
You could also link an external file to the HTML file. This is considered a better practice, as it organizes code better and you can avoid very lengthy HTML pages due to the JavaScript. In addition to these benefits, you can reuse the JavaScript on other web pages of your website without having to copy and paste. Say that you have the same JavaScript on 10 pages and you need to make a change to the script. You would only have to change one file if you did it in the way we are showing you in this example.
First, we are going to create a separate JavaScript file. These files have the postfix .js
. I'm going to call it ch1_alert.js
. This will be the content of our file:
alert("Saying hi from a different file!");
Then we are going to create a separate HTML file (using the postfix .html
again). And we are going to give it this content:
<html>
<script type="text/javascript" src="ch1_alert.js"></script>
</html>
Make sure that you put the files in the same location, or that you specify the path to the JavaScript file in your HTML. The names are case-sensitive and should match exactly.
You have two options. You can use a relative path and an absolute path. Let's cover the latter first since that is the easiest to explain. Your computer has a root. For Linux and macOS, it is /
, and for Windows, it is often C:/
. The path to the file starting from the root is the absolute path. This is the easiest to add because it will work on your machine. But there is a catch: on your machine, if this website folder later gets moved to a server, the absolute path will no longer work.
The second, safer option is relative paths. You specify how to get there from the file you are in at that time. So if it's in the same folder, you will only have to insert the name. If it's in a folder called "example" that is inside the folder that your file is in, you will have to specify example/nameOfTheFile.js
. And if it's a folder up, you would have to specify ../nameOfTheFile.js
.
If you open the HTML file, this is what you should get:
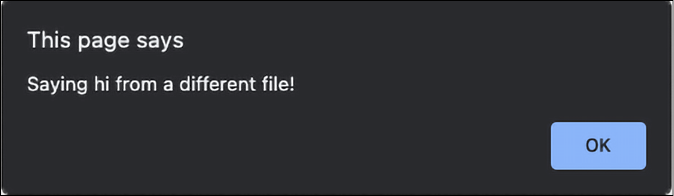
Figure 1.4: Popup created by JavaScript in a different file
Practice exercise 1.3
Linking to a JS JavaScript file: